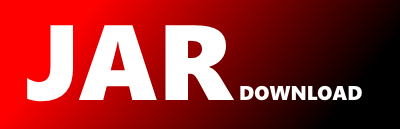
net.sf.cuf.ui.table.ContextMenuActionVisibilityDialog Maven / Gradle / Ivy
package net.sf.cuf.ui.table;
import net.sf.cuf.ui.SwingDecorator;
import javax.swing.JOptionPane;
/**
* Context menu action to perform a {@link VisibilityDialog} and apply the results on the table.
*
* @author Hendrik Wördehoff, sd&m AG
*/
public class ContextMenuActionVisibilityDialog
extends ContextMenuAction
{
/**
* Visibility dialog for the table.
* Will be instantiated when needed.
*/
private VisibilityDialog mVisDialog = null;
public String getKennung()
{
return mAdapter.getContextMenuKennung()+"_SHOWHIDE";
}
/**
* @return always true
*/
public boolean isEnabled()
{
return true;
}
/**
* The visibility dialog is opened initialised with the current model and visibility of the table.
* Afterwards the changes of the customer are applied to the table.
*
* The table returned by {@link ContextMenuAdapter#getTable} must be a {@link SortingTable}.
* @throws IllegalStateException if the table does not (yet) reside in a {@link java.awt.Frame}
*/
public void performAction()
throws IllegalStateException
{
SortingTable table = (SortingTable) mAdapter.getTable();
boolean[] visibility = table.getColumnsVisible();
outer:
for ( ; ; )
{
// show the dialog
visibility = getVisibilityDialog().show(mAdapter.getTable().getModel(), visibility);
// evaluate the result
if ( visibility == null )
{
return; // return without changing the visibility
}
// is at least one column still visible?
for (final boolean aVisibility : visibility)
{
if (aVisibility)
{
break outer; // proceed with changing the visibility
}
}
// warning message when trying to hide all columns
JOptionPane.showMessageDialog(mAdapter.getFrameForTable(),
SwingDecorator.getText ("TABLEVIS_MESSAGE"),
SwingDecorator.getTitle("TABLEVIS_MESSAGE"),
JOptionPane.WARNING_MESSAGE);
}
// change the visibility
table.setColumnsVisible(visibility);
}
/**
* Instantiate the visibility dialog lazily.
* This call only succeeds after the table has been embedded into a window.
* @return the visibility dialog for this context menu
* @throws IllegalStateException if the table does not (yet) reside in a {@link java.awt.Frame}
*/
private VisibilityDialog getVisibilityDialog()
throws IllegalStateException
{
if ( mVisDialog == null )
{
mVisDialog = new VisibilityDialog(mAdapter.getFrameForTable());
}
return mVisDialog;
}
}