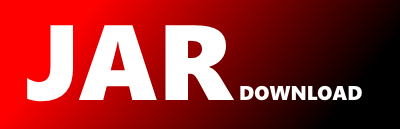
net.sf.cuf.ui.table.TableHeaderIcon Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.ui.table;
import java.awt.Component;
import java.awt.Graphics;
import java.awt.SystemColor;
import javax.swing.AbstractButton;
import javax.swing.Icon;
/**
* Icons for TableHeader in {@link TableHeaderRenderer}.
*
* @author Jörg Eichhorn, sd&m AG
*/
class TableHeaderIcon implements Icon
{
/** ascending or descending icon? */
private boolean mAscending = true;
/** the ascending icon */
protected static final Icon ICON_SORT_ASCENDING = new TableHeaderIcon(true);
/** the descending icon */
protected static final Icon ICON_SORT_DESCENDING = new TableHeaderIcon(false);
/**
* Create a new TableHeaderIcon.
*
* @param pAscending true
for ascending icon, false
for descending icon
*/
private TableHeaderIcon(final boolean pAscending)
{
mAscending = pAscending;
}
/**
* Get ascending icon.
*
* @return the ascending icon
*/
public static Icon getIconSortAscending()
{
return ICON_SORT_ASCENDING;
}
/**
* Get descending icon.
*
* @return the descending icon
*/
public static Icon getIconSortDescending()
{
return ICON_SORT_DESCENDING;
}
/**
* Returns the icon's height.
*
* @return an int specifying the fixed height of the icon.
*/
public int getIconHeight()
{
return 12;
}
/**
* Returns the icon's width.
*
* @return an int specifying the fixed width of the icon.
*/
public int getIconWidth()
{
return 8;
}
/**
* Draw the icon at the specified location.
*
* @param pComponent the component to draw in
* @param pGraphics the graphics-context to use
* @param pX the x-coordinate to draw the icon at
* @param pY the y-coordinate to draw the icon at
*/
public void paintIcon(final Component pComponent, final Graphics pGraphics, final int pX, final int pY)
{
boolean selected = ((AbstractButton) pComponent).isSelected() || ((AbstractButton) pComponent).getModel().isPressed();
int maxX = mAscending ? 7 : 5;
int maxY = 11;
pGraphics.translate(pX, pY);
if (selected)
{
if (mAscending)
{
pGraphics.setColor(SystemColor.controlDkShadow);
pGraphics.drawLine(1, 0, 1, maxY);
pGraphics.setColor(SystemColor.controlShadow);
pGraphics.drawLine(2, 0, maxX, maxY);
pGraphics.drawLine(2, maxY, maxX - 1, maxY);
pGraphics.setColor(SystemColor.control);
pGraphics.drawLine(2, 2, 2, maxY - 1);
pGraphics.setColor(SystemColor.controlShadow);
pGraphics.drawLine(3, 6, 3, 9);
pGraphics.drawLine(4, 8, 4, 9);
pGraphics.setColor(SystemColor.controlDkShadow);
pGraphics.drawLine(3, maxY - 1, maxX - 1, maxY - 1);
pGraphics.drawLine(3, 4, maxX - 2, maxY - 2);
}
else
{
pGraphics.setColor(SystemColor.controlDkShadow);
pGraphics.drawLine(0, 0, maxX - 1, maxY - 2);
pGraphics.drawLine(1, 0, maxX - 1, 0);
pGraphics.setColor(SystemColor.controlShadow);
pGraphics.drawLine(maxX, 0, maxX, maxY);
pGraphics.setColor(SystemColor.control);
pGraphics.drawLine(1, 1, 4, 1);
pGraphics.setColor(SystemColor.controlShadow);
pGraphics.drawLine(2, 2, 3, 2);
pGraphics.drawLine(2, 3, 3, 3);
pGraphics.drawLine(3, 4, 3, 5);
pGraphics.setColor(SystemColor.controlDkShadow);
pGraphics.drawLine(maxX - 1, 2, maxX - 1, 7);
}
}
else
{
if (mAscending)
{
pGraphics.setColor(SystemColor.controlDkShadow);
pGraphics.drawLine(1, 0, 1, maxY);
pGraphics.setColor(SystemColor.controlHighlight);
pGraphics.drawLine(2, 0, 8, maxY);
pGraphics.drawLine(2, maxY, maxX, maxY);
}
else
{
pGraphics.setColor(SystemColor.controlDkShadow);
pGraphics.drawLine(1, 0, maxX, 0);
pGraphics.drawLine(0, 0, maxX - 1, maxY - 2);
pGraphics.setColor(SystemColor.controlHighlight);
pGraphics.drawLine(maxX, 1, maxX, maxY);
}
}
pGraphics.translate(-pX, -pY);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy