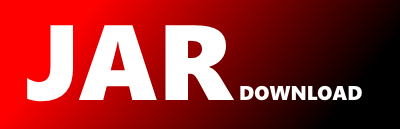
net.sf.cuf.ui.table.TableHeaderRenderer Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.ui.table;
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.Insets;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTable;
import javax.swing.border.BevelBorder;
import javax.swing.table.TableCellRenderer;
/**
* Special TableCellRenderer for tableheader of a {@link SortingTable}.
*
* @author Jörg Eichhorn, sd&m AG
*/
public class TableHeaderRenderer extends JPanel implements TableCellRenderer
{
/** Display Tableheader for ascending sorted column */
public static final int ASCENDING = +1;
/** Display Tableheader for unsorted column */
public static final int NONE = 0;
/** Display Tableheader for descending sorted column */
public static final int DESCENDING= -1;
// GUI-Komponenten
private JLabel mLabelCaption = new JLabel();
private JPanel mPanelButtons = new JPanel();
private JButton mButtonSortAscending = new JButton(TableHeaderIcon.getIconSortAscending());
private JButton mButtonSortDescending = new JButton(TableHeaderIcon.getIconSortDescending());
// current sorting direction of column, initially unsorted
private int mDirection = NONE;
// true after we have initialized ourself
private boolean mInitialized= false;
/**
* Create a new renderer.
*/
public TableHeaderRenderer()
{
initialize();
}
/**
* Set sorting direction of this tableheaders column.
*
* @param pDirection on of {@link #ASCENDING}, {@link #NONE}, {@link #DESCENDING}
*/
public void setDirection(final int pDirection)
{
if (pDirection != ASCENDING &&
pDirection != NONE &&
pDirection != DESCENDING)
{
throw new IllegalArgumentException("invalid value for pDirection");
}
mDirection = pDirection;
}
/**
* Checks what kind of button got hit at position (x
, y
).
*
* @param pX x-coordinate
* @param pY y-coordinate
* @return {@link #ASCENDING} or {@link #NONE} or {@link #DESCENDING}, depending on button that got hit
*/
public int hitButton(final int pX, final int pY)
{
Component component = mPanelButtons.findComponentAt(pX - mPanelButtons.getX(), pY - mPanelButtons.getY());
if (component != null && component.equals(mButtonSortAscending)) return ASCENDING;
if (component != null && component.equals(mButtonSortDescending)) return DESCENDING;
return NONE;
}
/**
* Implementation of {@link TableCellRenderer} interface.
*
* @param pTable the JTable
that is asking the
* renderer to draw; can be null
* @param pValue the value of the cell to be rendered. It is
* up to the specific renderer to interpret
* and draw the value. For example, if
* value
* is the string "true", it could be rendered as a
* string or it could be rendered as a check
* box that is checked. null
is a
* valid value
* @param pIsSelected true if the cell is to be rendered with the
* selection highlighted; otherwise false
* @param pHasFocus if true, render cell appropriately. For
* example, put a special border on the cell, if
* the cell can be edited, render in the color used
* to indicate editing
* @param pRow the row index of the cell being drawn. When
* drawing the header, the value of
* row
is -1
* @param pCol the column index of the cell being drawn
* @return the tableHeaderRenderer to use
*/
public Component getTableCellRendererComponent(final JTable pTable, final Object pValue, final boolean pIsSelected, final boolean pHasFocus, final int pRow, final int pCol)
{
mLabelCaption.setText(' ' + pValue.toString());
boolean ascending = mDirection == ASCENDING;
boolean descending = mDirection == DESCENDING;
mLabelCaption.setHorizontalAlignment(JLabel.LEFT);
mPanelButtons.setVisible(true);
mButtonSortAscending.setVisible(true);
mButtonSortAscending.getModel().setArmed(ascending);
mButtonSortAscending.getModel().setPressed(ascending);
mButtonSortDescending.setVisible(true);
mButtonSortDescending.getModel().setArmed(descending);
mButtonSortDescending.getModel().setPressed(descending);
return this;
}
/**
* Notification from the UIFactory that the L&F has changed.
* We call JPanel's updateUI and update our own widgets.
*/
public void updateUI()
{
super.updateUI();
if (mInitialized)
{
mLabelCaption.updateUI();
mPanelButtons.updateUI();
}
}
/**
* setup everything.
*/
private void initialize()
{
setBorder(new BevelBorder(BevelBorder.RAISED));
setLayout(new BorderLayout());
mPanelButtons.setLayout(new BoxLayout(mPanelButtons, BoxLayout.X_AXIS));
mPanelButtons.add(mButtonSortAscending);
mPanelButtons.add(mButtonSortDescending);
mButtonSortAscending.setBorder(null);
mButtonSortAscending.setBorderPainted(false);
mButtonSortAscending.setContentAreaFilled(false);
mButtonSortAscending.setMargin(new Insets(2, 0, 2, 0));
mButtonSortDescending.setBorder(null);
mButtonSortDescending.setBorderPainted(false);
mButtonSortDescending.setContentAreaFilled(false);
mButtonSortDescending.setMargin(new Insets(2, 0, 2, 0));
add(mLabelCaption, BorderLayout.CENTER);
add(mPanelButtons, BorderLayout.EAST);
mInitialized= true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy