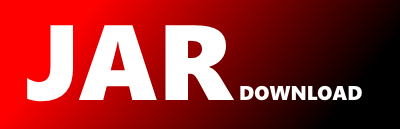
net.sf.cuf.ui.table.TableMap Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.ui.table;
import javax.swing.event.TableModelEvent;
import javax.swing.event.TableModelListener;
import javax.swing.table.AbstractTableModel;
import javax.swing.table.TableModel;
/**
* In a chain of data manipulators some behaviour is common. TableMap
* provides most of this behavour and can be subclassed by filters
* that only need to override a handful of specific methods. TableMap
* implements TableModel by routing all requests to its model, and
* TableModelListener by routing all events to its listeners. Inserting
* a TableMap which has not been subclassed into a chain of table filters
* should have no effect.
*
* Taken originally from "The Java Tutorial" by Sun Microsystems.
* Original Source was version 1.4 12/17/97 author Philip Milne
*
* @author Jörg Eichhorn, sd&m AG
*/
public class TableMap extends AbstractTableModel implements TableModelListener
{
/**
* Reference to the original table model.
*/
protected TableModel mModel;
/**
* Get the original table model.
* @return the original table model
*/
public TableModel getModel()
{
return mModel;
}
/**
* Set a new TableModel.
*
* @param pModel the new TableModel
*/
public void setModel(final TableModel pModel)
{
mModel = pModel;
pModel.addTableModelListener(this);
}
// By default, implement TableModel by forwarding all messages
// to the model.
/**
* Returns the value for the cell at aColumn
and
* aRow
.
*
* @param pRow the row whose value is to be queried
* @param pColumn the column whose value is to be queried
* @return the value Object at the specified cell
*/
public Object getValueAt(final int pRow, final int pColumn)
{
return mModel.getValueAt(pRow, pColumn);
}
/**
* Sets the value in the cell at aColumn
and
* aRow
to aValue
.
*
* @param pValue the new value
* @param pRow the row whose value is to be changed
* @param pColumn the column whose value is to be changed
*/
public void setValueAt(final Object pValue, final int pRow, final int pColumn)
{
mModel.setValueAt(pValue, pRow, pColumn);
}
/**
* Returns the number of rows in the model. A
* JTable
uses this method to determine how many rows it
* should display. This method should be quick, as it
* is called frequently during rendering.
*
* @return the number of rows in the model
*/
public int getRowCount()
{
return (mModel == null) ? 0 : mModel.getRowCount();
}
/**
* Returns the number of columns in the model. A
* JTable
uses this method to determine how many columns it
* should create and display by default.
*
* @return the number of columns in the model
*/
public int getColumnCount()
{
return (mModel == null) ? 0 : mModel.getColumnCount();
}
/**
* Returns the name of the column at aColumn
. This is used
* to initialize the table's column header name. Note: this name does
* not need to be unique; two columns in a table can have the same name.
*
* @param pColumn the index of the column
* @return the name of the column
*/
public String getColumnName(final int pColumn)
{
return mModel.getColumnName(pColumn);
}
/**
* Returns the most specific superclass for all the cell values
* in the column. This is used by the JTable
to set up a
* default renderer and editor for the column.
*
* @param pColumn the index of the column
* @return the common ancestor class of the object values in the model.
*/
public Class getColumnClass(final int pColumn)
{
return mModel.getColumnClass(pColumn);
}
/**
* Returns true if the cell at row
and
* column
* is editable. Otherwise, setValueAt
on the cell will not
* change the value of that cell.
*
* @param pRow the row whose value to be queried
* @param pColumn the column whose value to be queried
* @return true if the cell is editable
*/
public boolean isCellEditable(final int pRow, final int pColumn)
{
return mModel.isCellEditable(pRow, pColumn);
}
//
// Implementation of the TableModelListener interface,
//
/**
* This fine grain notification tells listeners the exact range
* of cells, rows, or columns that changed.
*
* @param pEvent the TableModelEvent containing notification
*/
public void tableChanged(final TableModelEvent pEvent)
{
fireTableChanged(pEvent);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy