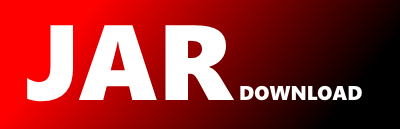
net.sf.cuf.ui.table.TableSortInfo Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.ui.table;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* TableSortInfo holds all information about current sorting order and
* sorting direction.
* The order of the entries is important, because this is the prio in which
* compares of multiple columns will be performed.
*
* @author Jörg Eichhorn, sd&m AG
*/
public class TableSortInfo
{
/** List of {@link Entry}s. */
private List mSortingCriteria;
/**
* Constructor.
*/
public TableSortInfo()
{
mSortingCriteria = new ArrayList<>();
}
/**
* Copy constructor for immutable copies.
* The new instance will see all changes applied to the original object.
* @param pOriginal the object we copy
*/
private TableSortInfo(final TableSortInfo pOriginal)
{
mSortingCriteria = Collections.unmodifiableList(pOriginal.mSortingCriteria);
}
/**
* Create an immutable clone of this object.
* The clone will see all changes applied to the original object.
* @return cloned object
*/
public TableSortInfo cloneImmutable()
{
return new TableSortInfo(this);
}
/**
* Clear the current sorting.
*/
public void clear()
{
mSortingCriteria.clear();
}
/**
* Get the number of sorting information entries.
* @return number of entries
*/
public int size()
{
return mSortingCriteria.size();
}
/**
* Check if there is a sorting defined for a column.
* @param pColumnIndex model index of a column
* @return true
if there is a sorting entry for the column
*/
public boolean isSorted(final int pColumnIndex)
{
return find(pColumnIndex) >= 0;
}
/**
* Find a sorting entry for a column.
* @param pColumnIndex model index of a column
* @return entry index of the sorting info or -1 if the column is not sorted
*/
public int find(final int pColumnIndex)
{
int entryIndex = 0;
for (final Object aSortingCriteria : mSortingCriteria)
{
Entry entry = (Entry) aSortingCriteria;
if (entry.getPColumn() == pColumnIndex) return entryIndex;
entryIndex++;
}
return -1;
}
/**
* Get colum information from a sorting entry.
* @param pEntryIndex index of sorting entry
* @return model column index
*/
public int getColumn(final int pEntryIndex)
{
return mSortingCriteria.get(pEntryIndex).getPColumn();
}
/**
* Get ascending/descending information from a sorting entry.
* @param pEntryIndex index of sorting entry
* @return true
if sorting is to be done ascending
*/
public boolean isAscending(final int pEntryIndex)
{
return mSortingCriteria.get(pEntryIndex).isAscending();
}
/**
* Append sorting information entry.
* The sorting information is appended to the currently defined sorting.
* For a new sorting use {@link #clear} first.
* @param pColumn model index of column to be sorted
* @param pAscending true
if sorting is to be done ascending
*/
public void sortByColumn(final int pColumn, final boolean pAscending)
{
Entry entry = new Entry(pColumn, pAscending);
// remove sorting info for same column.
// no need to check if column was previously added, because remove will not
// fail, if no such element is present in sortingColumns.
// remove returns a boolean to indicate successful removal, which is silently ignored at this point.
mSortingCriteria.remove(entry);
// add new sorting info for column at the end of sortingColumns.
// it is assumed, that no sorting info for that column is in sortingColumns at this point.
mSortingCriteria.add(entry);
}
/*** Inner classes ********************************************************/
/**
* Immutable sorting information entry.
*/
public static class Entry
{
/**
* column model-index
*/
private int mColumn;
/**
* sorting direction
*/
private boolean mAscending;
/**
* Construct a new TableSortInfo.
*
* @param pColumn the column as model-index
* @param pAscending the sorting direction
*/
public Entry(final int pColumn, final boolean pAscending)
{
mColumn = pColumn;
mAscending = pAscending;
}
/**
* Get column as model-index
*
* @return column as model-index
*/
public int getPColumn()
{
return mColumn;
}
/**
* Get sorting direction
*
* @return sorting direction is ascending
*/
public boolean isAscending()
{
return mAscending;
}
/**
* Check if two TableSortInfo.Entry objects refer to the same column.
* This notion of equality is useful for keeping objects in data structures.
*
* @param pOther the other entry
* @return this and obj are equal
*/
public boolean equals(final Object pOther)
{
if (pOther instanceof Entry)
{
Entry other = (Entry) pOther;
return mColumn == other.mColumn;
}
else
{
// cannot be equal, if other object is not a TableSortInfo.Entry
return false;
}
}
/**
* Generate a hash code based on the column information.
*
* @return a hash code consistent with {@link #equals(Object)}
*/
public int hashCode()
{
return mColumn;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy