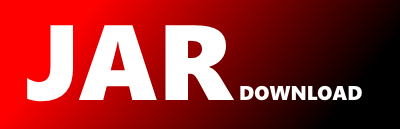
dcutils.cards.mtg.ColorlessMana Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dcutils Show documentation
Show all versions of dcutils Show documentation
Contains convenience classes for: tuples, ranges, graphs, etc.
package dcutils.cards.mtg;
/**
* Represents a colorless mana type in Magic the Gathering.
* Examples are:
*
: 1 colorless mana
*
: 2 colorless mana
*
: 3 colorless mana
*
: 4 colorless mana
*
: 5 colorless mana
* @author dca
*/
public class ColorlessMana implements Mana {
/** The name of the mana.
*/
private String name = "colorless";
/** The number of colorless mana.
*/
private Integer num;
/**
* The constructor initializes the num to null,
* indicating X colorless mana.
*/
public ColorlessMana() {
this.num = null; // null implies X
} // END constructor
/**
* The constructor initializes the number of colorless mana.
* @param num
*/
public ColorlessMana(Integer num) {
this.num = num;
} // END constructor
/**
* The getter for the name of the colorless mana.
* This is always "colorless".
* @return String "colorless"
*/
@Override
public String getName() {
return this.name;
} // END getName
/**
* The getter for the number of colorless mana.
* @return Integer The number of colorless mana.
*/
public Integer getNum() {
return this.num;
} // END getNum
/**
* The getter for the code letter of the mana.
* @return code The code letter of the mana.
*/
@Override
public String getCode() {
return (null != num ? String.valueOf(num) : "X");
} // END getCode
/**
* Returns a string representation of the colorless mana.
* This will be either:
* {@literal #}:colorless
* X:colorless
* @return String The colon delimited number and name of this colorless mana.
*/
@Override
public String toString() {
return String.format(
"%s:%s",
(null != num ? num : "X"), getName()
);
} // END toString
/**
* Decides if an object is equal to this colorless mana.
* In order for an object to be equal to this colorless mana:
* The object must also be colorless mana, and must have the same number.
* @param obj The item to check for value equality.
* @return True or false
*/
@Override
public boolean equals(Object obj) {
if(null == obj) {
return false;
} else if(obj instanceof ColorlessMana) {
ColorlessMana mana = (ColorlessMana)obj;
return mana.getNum() == this.getNum();
} else {
return false;
} // END if/else
} // END equals
/**
* Creates a hash code for this colorless mana.
* The hash code uses prime numbers and the number of colorless mana.
* @return integer The hash code.
*/
@Override
public int hashCode() {
int hashCode = 101;
if(null != num) hashCode = (11 * hashCode) + num;
return hashCode;
} // END hashCode
} // END class ColorlessMana
© 2015 - 2025 Weber Informatics LLC | Privacy Policy