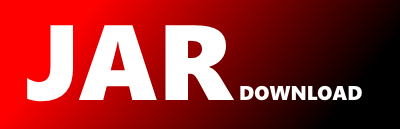
dcutils.cards.mtg.ManaCost Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dcutils Show documentation
Show all versions of dcutils Show documentation
Contains convenience classes for: tuples, ranges, graphs, etc.
package dcutils.cards.mtg;
// Import JDK Classes
import java.util.ArrayList;
/**
* Represents how much mana a card costs to play in Magic the Gathering.
* @author dca
*/
public class ManaCost extends ArrayList implements PrettyPrintable {
/** Unique ID for serialization */
private static final long serialVersionUID = -576307043970583870L;
public ManaCost(Mana ... mana) {
for(Mana manaItem : mana) {
this.add(manaItem);
} // END loop
} // END constructor
public int getConvertedManaCost() {
int total = 0;
for(Mana mana : this) {
if(mana instanceof ColorlessMana) {
total = totalColorlessMana(total, (ColorlessMana)mana);
} else if(mana instanceof HybridMana) {
final HybridMana hMana = (HybridMana)mana;
if(hMana.first() instanceof ColorlessMana) {
total = totalColorlessMana(total, (ColorlessMana)hMana.first());
} else if(hMana.second() instanceof ColorlessMana) {
total = totalColorlessMana(total, (ColorlessMana)hMana.second());
} else {
total++;
} // END if/else
} else {
total++;
} // END if/else
} // END mana loop
return total;
} // END getConvertedManaCost
private int totalColorlessMana(int total, ColorlessMana mana) {
final ColorlessMana cMana = (ColorlessMana)mana;
final Integer cNum = cMana.getNum();
if(null != cNum) {
total += cNum;
} // END if
return total;
} // END totalColorlessMana
@Override
public String toString() {
StringBuffer buffer = new StringBuffer(1024);
buffer.append(this.getClass().getSimpleName());
buffer.append(" ");
buffer.append(super.toString());
return buffer.toString();
} // END toString
@Override
public String prettyPrint() {
return this.toString()
.replace("[", String.format("[%n\t"))
.replace(", ", String.format(",%n\t"))
.replace("]", String.format("%n]"));
} // END prettyPrint
} // END class ManaCost
© 2015 - 2025 Weber Informatics LLC | Privacy Policy