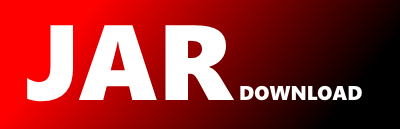
dcutils.cards.mtg.Keyword Maven / Gradle / Ivy
package dcutils.cards.mtg;
// Import JDK Classes
import java.util.Arrays;
import java.util.Iterator;
import java.util.List;
/**
* Represents all the keywords found on cards in Magic the Gathering.
* @author dca
*/
public enum Keyword implements Iterable {
AWAKEN("Awaken"),
BATTALION("Battalion"),
BESTOW("Betow"),
BOLSTER("Bolster"),
CIPHER("Cipher"),
COHORT("Cohort"),
CONSTELLATION("Constellation"),
CONVERGE("Converge"),
CONVOKE("Convoke", String.format("Your creatures can help cast this spell. Each creature you tap while casting this spell pays for %s or one mana of that creature's color.", new ManaCost(new ColorlessMana(1)))),
DASH("Dash", "You may cast this spell for its dash cost. If you do, it gains haste, and it's returned from the battlefield to its owner's hand at the beginning of the next end step."),
DEATHTOUCH("Deathtouch", "Any amount of damage this deals to a creature is enough to destroy it."),
DEFENDER("Defender", "This creature can't attack."),
DELIRIUM("Delirium"),
DELVE("Delve", String.format("Each card you exile from your graveyard while casting this spell pays for %s.", new ManaCost(new ColorlessMana(1)))),
DEVOID("Devoid", "This card has no color."),
DOUBLE_STRIKE("Double-strike", "This creature deals both first-strike and regular combat damage."),
ENCHANT("Enchant"),
EQUIP("Equip"),
EVOLVE("Evolve", "Whenever a creature enters the battlefield under your control, if that creature has greater power or toughness than this creature, put a +1/+1 counter on this creature."),
EXPLOIT("Exploit", "When this creature enters the battlefield, you may sacrifice a creature."),
EXTORT("Extort"),
FEROCIOUS("Ferocious"),
FIRST_STRIKE("First-strike"),
FLASH("Flash", "You may cast this spell any time you could cast an instant."),
FLYING("Flying", "This creature can't be blocked except by creatures with flying or reach."),
FORESTWALK("Forestwalk", "This creature can't be blocked as long as defending player controls a Forest."),
FUSE("Fuse", "You may cast one or both halves of this card from your hand."),
FORMIDABLE("Formidable"),
HASTE("Haste"),
HEROIC("Heroic"),
HEXPROOF("Hexproof", "This creature can't be the target of spells or abilities your opponents control."),
INDESTRUCTIBLE("Indestructible"),
INGEST("Ingest", "Whenever this creature deals combat damage to a player, that player exiles the top card of his or her library."),
INSPIRED("Inspired"),
INTIMIDATE("Intimidate"),
INVESTIGATE("Investigate", String.format("Put a colorless Clue artifact token onto the battlefield with \"%s, Sacrifice this artifact: Draw a card.\"", new ManaCost(new ColorlessMana(2)))),
ISLANDWALK("Islandwalk", "This creature can't be blocked as long as defending player controls an Island."),
LANDFALL("Landfall"),
LANDWALK("Landwalk"),
LIFELINK("Lifelink", "Damage dealt by this creature also causes you to gain that much life."),
MADNESS("Madness", "If you discard this card, discard it into exile. When you do, cast it for its madness cost or put it into your graveyard."),
MANIFEST("Manifest", "onto the battlefield face down as a 2/2 creature. Turn it face up any time for its mana cost if it's a creature card."),
MEGAMORPH("Megamorph", String.format("You may cast this card face down as a 2/2 creature for %s. Turn it face up any time for its megamorph cost and put a +1/+1 counter on it.", new ManaCost(new ColorlessMana(3)))),
MENACE("Menace", "This creature can't be blocked except by two or more creatures."),
MONSTROSITY("Monstrosity"),
MORPH("Morph", String.format("You may cast this card face down as a 2/2 creature for %s. Turn it face up any time for its morph cost.", new ManaCost(new ColorlessMana(3)))),
OUTLAST("Outlast", "Put a +1/+1 counter on this creature. Outlast only as a sorcery."),
OVERLOAD("Overload"),
POPULATE("Populate", "Put a token onto the battlefield that's a copy of a creature token you control."),
PROTECTION("Protection"),
PROWESS("Prowess", "Whenever you cast a noncreature spell, this creature gets +1/+1 until end of turn."),
RAID("Raid"),
RALLY("Rally"),
RAMPAGE("Rampage"),
REACH("Reach", "This creature can block creatures with flying."),
REBOUND("Rebound", "If you cast this spell from your hand, exile it as it resolves. At the beginning of your next upkeep, you may cast this card from exile without paying its mana cost."),
RENOWN("Renown", "When this creature deals combat damage to a player, if it isn't renowned, put a +1/+1 counter on it and it becomes renowned."),
SCAVENGE("Scavenge", "Exile this card from your graveyard: Put a number of +1/+1 counters equal to this card's power on target creature. Scavenge only as a sorcery."),
SCRY("Scry"),
SHROUD("Shroud"),
Skulk("Skulk", "This creature can't be blocked by creatures with greater power."),
SPELL_MASTERY("Spell-mastery"),
STRIVE("Strive"),
SUPPORT("Support"),
SURGE("Surge", "You may cast this spell for its surge cost if your or a teammate has cast another spell this turn."),
TRAMPLE("Trample", "If this creature would assign enough damage to its blockers to destroy them, you may have it assign the rest of its damage to defending player or planeswalker."),
TRIBUTE("Tribute"),
UNLEASH("Unleash"),
VIGILANCE("Vigilance", "Attacking doesn't cause this creature to tap.");
/** The name of the mana.
*/
private String name;
/** The description of the keyword.
*/
private String desc;
/**
* The constructor initializes the name.
* @param name The name of the creature type.
*/
private Keyword(String name) {
this.name = name;
this.desc = "";
} // END constructor
/**
* The constructor initializes the name and description.
* @param name The name of the keyword.
* @param desc The description of the keyword.
*/
private Keyword(String name, String desc) {
this.name = name;
this.desc = desc;
} // END constructor
/**
* The getter for the name of the keyword.
* @return name The name of the keyword.
*/
public String getName() {
return this.name;
} // END getName
/**
* The getter for the description of the keyword.
* @return desc The description of the keyword.
*/
public String getDesc() {
return this.desc;
} // END getDesc
public boolean hasDesc() {
return !"".equals(this.desc);
} // END hasDesc
/**
* Returns an array of all enum values.
* @return Keyword[] An array of all enum values.
*/
public static Keyword[] asArray() {
return values();
} // END asArray
/**
* Returns a list of all enum values.
* @return List A list of all enum values.
*/
public static List asList() {
return Arrays.asList(asArray());
} // END asList
/**
* Returns an iterator over all enum values.
* @return Iterator An iterator over all enum values.
*/
@Override
public Iterator iterator() {
return asList().iterator();
} // END iterator
/**
* The getter for the name of the mana.
* @return name The name of the mana.
*/
@Override
public String toString() {
return getName();
} // END toString
} // END enum Keyword
© 2015 - 2025 Weber Informatics LLC | Privacy Policy