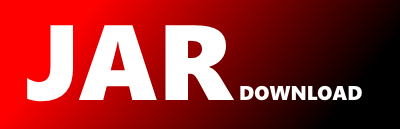
dcutils.rpg.currency.Currency Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dcutils Show documentation
Show all versions of dcutils Show documentation
Contains convenience classes for: tuples, ranges, graphs, etc.
package dcutils.rpg.currency;
// Import Java JDK Classes
import java.util.Arrays;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
/**
* A currency object wraps an integer.
* This integer is the overall value (total) of the currency.
* It can be defined by another integer, Quantities, or Units.
* It is divisible by Units, as to express it in readable terms.
* Example usage:
Currency c = new Currency(
Quantity.getQuantity(Unit.SILVER, 10),
Quantity.getQuantity(Unit.GOLD, 15),
Quantity.getQuantity(Unit.COPPER, 5)
);
System.out.println(c); /* PRINTS
Gold (15)
Silver (10)
Copper (5)
*/
System.out.println(c.getBaseValue()); /* PRINTS
151005
*/
c.add(Quantity.getQuantity(Unit.SILVER, 95));
System.out.println(c); /* PRINTS
Gold (16)
Silver (5)
Copper (5)
*/
System.out.println(c.getBaseValue()); /* PRINTS
160505
*/
* @see Quantity
* @see Unit
* @author dca
*/
public class Currency implements Comparator {
/** The value of the currency. All added currencies and quantities get added to this single value.
*/
private int baseValue;
/**
* The constructor initializes the base value of this currency
* with the supplied integer value.
* @param value The supplied integer value.
*/
public Currency(int value) {
setBaseValue(value);
} // END constructor
/**
* The constructor initializes the base value of this currency
* with the supplied array of quantities.
* @param quantities The supplied array of quantities.
*/
public Currency(Quantity ... quantities) {
if(null != quantities) {
for(Quantity quantity : quantities) {
add(quantity);
} // END loop
} // END if
} // END constructor
/**
* The copy constructor initializes the base value of this currency
* with the supplied currency.
* @param currency The supplied currency.
*/
public Currency(Currency currency) {
if(null != currency) {
add(currency);
} // END if
} // END constructor
/**
* The getter for the base value of the currency.
* @return baseValue The base value of the currency.
*/
public int getBaseValue() {
return baseValue;
} // END getBaseValue
/**
* The setter for the base value of the currency.
* @param value The value to set to the base value of the currency.
*/
public void setBaseValue(int value) {
baseValue = value;
} // END setBaseValue
/**
* Adds the supplied quantity to the base value of the currency.
* @param quantity The supplied quantity.
*/
public void add(Quantity quantity) {
if(null != quantity) {
baseValue += quantity.getBaseValue();
} // END if
} // END add
/**
* Adds the supplied currency to the base value of this currency.
* @param currency The supplied currency.
*/
public void add(Currency currency) {
if(null != currency) {
baseValue += currency.getBaseValue();
} // END if
} // END add
/**
* Subtracts the supplied quantity from the base value of the currency.
* This only happens if the base value was greater than or equal to that of the supplied quantity.
* @param quantity The supplied quantity.
* @return True if the base value was greater than or equal to that of the supplied quantity; false otherwise.
*/
public boolean subtract(Quantity quantity) {
if(canAfford(quantity)) {
if(null != quantity) {
baseValue -= quantity.getBaseValue();
} // END if
return true;
} else {
return false;
} // END if/else
} // END subtract
/**
* Subtracts the supplied currency from the base value of this currency.
* This only happens if the base value was greater than or equal to that of the supplied currency.
* @param currency The supplied currency.
* @return True if the base value was greater than or equal to that of the supplied currency; false otherwise.
*/
public boolean subtract(Currency currency) {
if(canAfford(currency)) {
if(null != currency) {
baseValue -= currency.getBaseValue();
} // END if
return true;
} else {
return false;
} // END if/else
} // END subtract
/**
* Determines if the base value is greater than or equal to that of the supplied quantity.
* @param cost The supplied quantity.
* @return True if the base value is greater than or equal to that of the supplied quantity; false otherwise.
*/
public boolean canAfford(Quantity cost) {
if(null != cost) {
return baseValue >= cost.getBaseValue();
} else {
return true;
} // END if/else
} // END canAfford
/**
* Determines if the base value is greater than or equal to that of the supplied currency.
* @param cost The supplied currency.
* @return True if the base value is greater than or equal to that of the supplied currency; false otherwise.
*/
public boolean canAfford(Currency cost) {
if(null != cost) {
return baseValue >= cost.getBaseValue();
} else {
return true;
} // END if/else
} // END canAfford
/**
* Returns a string representation of this currency, specified in terms of highest-order quantities.
* @return String A string representation of this currency.
*/
@Override
public String toString() {
final StringBuffer buffer = new StringBuffer(512);
final List units = Arrays.asList(Unit.values());
int tempValue = baseValue;
Collections.reverse(units); // highest to lowest
for(Unit unit : units) {
if(tempValue >= unit.getBaseValue() || !buffer.toString().isEmpty()) {
int amt = tempValue / unit.getBaseValue();
int rem = tempValue % unit.getBaseValue();
if(!buffer.toString().isEmpty()) buffer.append(String.format("%n"));
buffer.append(new Quantity(unit, amt));
tempValue = rem;
} // END if
} // END loop
return buffer.toString();
} // END toString
/**
* Decides if an object is equal to this currency.
* In order for an object to be equal to this currency:
* the object must also be a currency, must not be null, and its baseValue must be equal to this baseValue.
* @param obj The item to check for value equality.
* @return True or false
*/
@Override
public boolean equals(Object obj) {
if(null == obj) {
return false;
} else if(obj instanceof Currency) {
Currency currency = (Currency)obj;
return this.getBaseValue() == currency.getBaseValue();
} else {
return false;
} // END if/else
} // END equals
/**
* Creates a hash code for this currency.
* The hash code uses prime numbers and the baseValue.
* @return integer The hash code.
*/
@Override
public int hashCode() {
int hashCode = 101;
hashCode = (11 * hashCode) + baseValue;
return hashCode;
} // END hashCode
@Override
public int compare(Currency lhs, Currency rhs) {
if(null == lhs ^ null == rhs) return -1;
else if(null == lhs && null == rhs) return 0;
else return lhs.getBaseValue() - rhs.getBaseValue();
} // END compare
} // END class Currency
© 2015 - 2025 Weber Informatics LLC | Privacy Policy