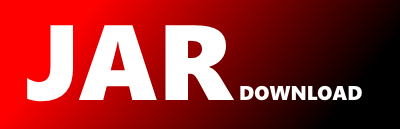
dcutils.tuples.Pair Maven / Gradle / Ivy
package dcutils.tuples;
// Import Java JDK Classes
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.Comparator;
import java.util.Iterator;
import java.util.List;
/**
* Contains exactly two items.
* @author dca
*/
public class Pair implements Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy