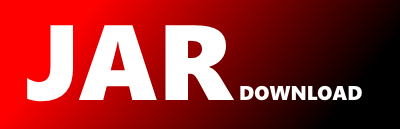
net.sf.doolin.tabular.csv.CSVWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doolin-tabular Show documentation
Show all versions of doolin-tabular Show documentation
This Doolin GUI module is an extension that allows writing
tabular data (CSV, Excel).
The newest version!
/*
* Created on Aug 14, 2007
*/
package net.sf.doolin.tabular.csv;
import java.io.BufferedWriter;
import java.io.IOException;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import net.sf.doolin.tabular.io.TabularWriter;
import net.sf.doolin.tabular.model.TabularColumn;
import net.sf.doolin.tabular.model.TabularModel;
import org.apache.commons.lang.StringUtils;
/**
* Writer for CSV format.
*
* @author Damien Coraboeuf
* @param
* Type of object per row
*/
public class CSVWriter implements TabularWriter {
private String encoding = "UTF-8";
private String separator = ",";
private static final Map, CSVAdapter> defaultAdapters = new HashMap, CSVAdapter>();
static {
defaultAdapters.put(boolean.class, new PrimitiveCSVAdapter());
defaultAdapters.put(byte.class, new PrimitiveCSVAdapter());
defaultAdapters.put(short.class, new PrimitiveCSVAdapter());
defaultAdapters.put(int.class, new PrimitiveCSVAdapter());
defaultAdapters.put(long.class, new PrimitiveCSVAdapter());
defaultAdapters.put(float.class, new PrimitiveCSVAdapter());
defaultAdapters.put(double.class, new PrimitiveCSVAdapter());
defaultAdapters.put(BigDecimal.class, new PrimitiveCSVAdapter());
defaultAdapters.put(Boolean.class, new PrimitiveCSVAdapter());
defaultAdapters.put(Byte.class, new PrimitiveCSVAdapter());
defaultAdapters.put(Short.class, new PrimitiveCSVAdapter());
defaultAdapters.put(Integer.class, new PrimitiveCSVAdapter());
defaultAdapters.put(Long.class, new PrimitiveCSVAdapter());
defaultAdapters.put(Float.class, new PrimitiveCSVAdapter());
defaultAdapters.put(Double.class, new PrimitiveCSVAdapter());
defaultAdapters.put(String.class, new StringCSVAdapter());
}
private CSVAdapter defaultAdapter = new StringCSVAdapter();
private Map, CSVAdapter> adapters = new HashMap, CSVAdapter>();
/**
* Returns the defaultAdapter
property.
*
* @return defaultAdapter
property.
*/
public CSVAdapter getDefaultAdapter() {
return this.defaultAdapter;
}
/**
* Returns the encoding
property. It defaults to UTF-8.
*
* @return encoding
property.
*/
public String getEncoding() {
return this.encoding;
}
/**
* Returns the separator
property. It defaults to ",".
*
* @return separator
property.
*/
public String getSeparator() {
return this.separator;
}
/**
* Sets the defaultAdapter
property.
*
* @param defaultAdapter
* defaultAdapter
property.
*/
public void setDefaultAdapter(CSVAdapter defaultAdapter) {
this.defaultAdapter = defaultAdapter;
}
/**
* Sets the encoding
property. It defaults to UTF-8.
*
* @param encoding
* encoding
property.
*/
public void setEncoding(String encoding) {
this.encoding = encoding;
}
/**
* Sets the separator
property. It defaults to ",".
*
* @param separator
* separator
property.
*/
public void setSeparator(String separator) {
this.separator = separator;
}
public void write(OutputStream output, TabularModel model)
throws IOException {
// Creates a writer wrapper, using specified encoding
PrintWriter writer = new PrintWriter(new BufferedWriter(
new OutputStreamWriter(output, this.encoding)));
try {
// 1 - Columns
List line = new ArrayList();
int columnCount = model.getColumnCount();
for (int i = 0; i < columnCount; i++) {
TabularColumn column = model.getColumn(i);
String name = column.getTitle();
line.add(name);
}
writeLine(writer, line);
// N - Rows
model.reset();
T item;
while ((item = model.next()) != null) {
// New line
line = new ArrayList();
// For each column
for (int col = 0; col < columnCount; col++) {
TabularColumn column = model.getColumn(col);
// Data for this cell
Object value = column.getValue(item);
// Adapt for CSV
String csvValue = getCSVValue(value);
// Appends it to the line
line.add(csvValue);
}
// Writes the line
writeLine(writer, line);
}
} finally {
// Ok
writer.flush();
}
}
/**
* Adapts a value for CSV
*
* @param value
* Value to adapt
* @return Adapted value
*/
protected String getCSVValue(Object value) {
if (value == null) {
return "";
} else {
// Adapter
CSVAdapter adapter;
// Current adapter
Class> type = value.getClass();
adapter = this.adapters.get(type);
// Default adapters
if (adapter == null) {
adapter = defaultAdapters.get(type);
}
// Default adapters
if (adapter == null) {
adapter = this.defaultAdapter;
}
// Adapt
String string = adapter.getCSVValue(value);
// Ok
return string;
}
}
/**
* Writes a line in the CSV destination.
*
* @param writer
* Writer to write to
* @param line
* Line to write
*/
protected void writeLine(PrintWriter writer, List line) {
String wholeLine = StringUtils.join(line.iterator(), this.separator);
writer.println(wholeLine);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy