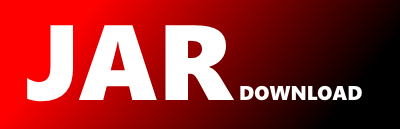
net.sf.doolin.tabular.excel.ExcelWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of doolin-tabular Show documentation
Show all versions of doolin-tabular Show documentation
This Doolin GUI module is an extension that allows writing
tabular data (CSV, Excel).
The newest version!
/*
* Created on Aug 14, 2007
*/
package net.sf.doolin.tabular.excel;
import java.io.IOException;
import java.io.OutputStream;
import java.util.Date;
import net.sf.doolin.tabular.io.TabularWriter;
import net.sf.doolin.tabular.model.TabularColumn;
import net.sf.doolin.tabular.model.TabularModel;
import org.apache.commons.lang.ObjectUtils;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFCellStyle;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
/**
* Writes to an Excel workbook.
*
* @author Damien Coraboeuf
* @param
* Type of object per row
*/
public class ExcelWriter implements TabularWriter {
public void write(OutputStream output, TabularModel model)
throws IOException {
// Creates the workbook
HSSFWorkbook workbook = new HSSFWorkbook();
// Creates a sheet
HSSFSheet sheet = workbook.createSheet();
// Freezes the first row
sheet.createFreezePane(0, 1, 0, 1);
// 1 - Titles
HSSFRow row = sheet.createRow(0);
int cellIndex = 0;
int columnCount = model.getColumnCount();
for (int i = 0; i < columnCount; i++) {
TabularColumn column = model.getColumn(i);
String title = column.getTitle();
// Creates the cell
HSSFCell cell = row.createCell(cellIndex++);
// Value
cell.setCellValue(title);
}
// N - Rows
int rowIndex = 0;
T item;
while ((item = model.next()) != null) {
// New row
row = sheet.createRow(rowIndex + 1);
// For each column
cellIndex = 0;
for (int col = 0; col < columnCount; col++) {
TabularColumn column = model.getColumn(col);
// Data for this cell
Object value = column.getValue(item);
// Creates the cell
HSSFCell cell = row.createCell(cellIndex++);
// Sets the value
if (value != null) {
if (value instanceof Boolean) {
cell.setCellValue((Boolean) value);
} else if (value instanceof Double) {
cell.setCellValue((Double) value);
} else if (value instanceof Integer) {
cell.setCellValue((Integer) value);
} else if (value instanceof Date) {
cell.setCellValue((Date) value);
// Set the format
HSSFCellStyle style = workbook.createCellStyle();
style.setDataFormat((short) 0xF);
cell.setCellStyle(style);
} else {
String string = ObjectUtils.toString(value, "");
cell.setCellValue(string);
}
}
}
// New row
rowIndex++;
}
// Writes the workbook
workbook.write(output);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy