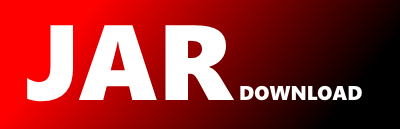
org.dozer.cache.DozerCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dozer Show documentation
Show all versions of dozer Show documentation
Dozer is a powerful Java Bean to Java Bean mapper that recursively copies data from one object to another
/*
* Copyright 2005-2008 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dozer.cache;
import org.apache.commons.lang.builder.ReflectionToStringBuilder;
import org.apache.commons.lang.builder.ToStringStyle;
import org.dozer.stats.GlobalStatistics;
import org.dozer.stats.StatisticType;
import org.dozer.stats.StatisticsManager;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
/**
* Map backed Cache implementation.
*
* @author tierney.matt
* @author dmitry.buzdin
*/
public class DozerCache implements Cache {
private final String name;
private final LRUMap cacheMap;
StatisticsManager statMgr = GlobalStatistics.getInstance().getStatsMgr();
public DozerCache(final String name, final int maximumSize) {
if (maximumSize < 1) {
throw new IllegalArgumentException("Dozer cache max size must be greater than 0");
}
this.name = name;
this.cacheMap = new LRUMap(maximumSize); // TODO This should be in Collections.synchronizedMap()
}
public void clear() {
cacheMap.clear();
}
public synchronized void put(KeyType key, ValueType value) {
if (key == null) {
throw new IllegalArgumentException("Cache entry key cannot be null");
}
CacheEntry cacheEntry = new CacheEntry(key, value);
cacheMap.put(cacheEntry.getKey(), cacheEntry);
}
public ValueType get(KeyType key) {
if (key == null) {
throw new IllegalArgumentException("Key cannot be null");
}
CacheEntry result = cacheMap.get(key);
if (result != null) {
statMgr.increment(StatisticType.CACHE_HIT_COUNT, name);
return result.getValue();
} else {
statMgr.increment(StatisticType.CACHE_MISS_COUNT, name);
return null;
}
}
public void addEntries(Collection> entries) {
for (CacheEntry entry : entries) {
cacheMap.put(entry.getKey(), entry);
}
}
public Collection> getEntries() {
return cacheMap.values();
}
public String getName() {
return name;
}
public long getSize() {
return cacheMap.size();
}
public long getMaxSize() {
return cacheMap.maximumSize;
}
public boolean containsKey(KeyType key) {
return cacheMap.containsKey(key);
}
public Set keySet() {
return cacheMap.keySet();
}
@Override
public String toString() {
return ReflectionToStringBuilder.toString(this, ToStringStyle.MULTI_LINE_STYLE);
}
class LRUMap extends LinkedHashMap> {
private int maximumSize;
LRUMap(int maximumSize) {
this.maximumSize = maximumSize;
}
@Override
protected boolean removeEldestEntry(Map.Entry> eldest) {
return size() > maximumSize;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy