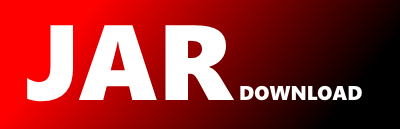
com.fasterxml.jackson.databind.deser.impl.JavaUtilCollectionsDeserializers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ehcache Show documentation
Show all versions of ehcache Show documentation
Ehcache is an open source, standards-based cache used to boost performance,
offload the database and simplify scalability. Ehcache is robust, proven and full-featured and
this has made it the most widely-used Java-based cache.
package com.fasterxml.jackson.databind.deser.impl;
import java.util.*;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JavaType;
import com.fasterxml.jackson.databind.JsonDeserializer;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.deser.std.StdDelegatingDeserializer;
import com.fasterxml.jackson.databind.type.TypeFactory;
import com.fasterxml.jackson.databind.util.Converter;
/**
* Helper class used to contain logic for deserializing "special" containers
* from {@code java.util.Collections} and {@code java.util.Arrays}. This is needed
* because they do not have usable no-arguments constructor: however, are easy enough
* to deserialize using delegating deserializer.
*
* @since 2.9.4
*/
public abstract class JavaUtilCollectionsDeserializers
{
private final static int TYPE_SINGLETON_SET = 1;
private final static int TYPE_SINGLETON_LIST = 2;
private final static int TYPE_SINGLETON_MAP = 3;
private final static int TYPE_UNMODIFIABLE_SET = 4;
private final static int TYPE_UNMODIFIABLE_LIST = 5;
private final static int TYPE_UNMODIFIABLE_MAP = 6;
public final static int TYPE_AS_LIST = 7;
// 10-Jan-2018, tatu: There are a few "well-known" special containers in JDK too:
private final static Class> CLASS_AS_ARRAYS_LIST = Arrays.asList(null, null).getClass();
private final static Class> CLASS_SINGLETON_SET;
private final static Class> CLASS_SINGLETON_LIST;
private final static Class> CLASS_SINGLETON_MAP;
private final static Class> CLASS_UNMODIFIABLE_SET;
private final static Class> CLASS_UNMODIFIABLE_LIST;
private final static Class> CLASS_UNMODIFIABLE_MAP;
static {
Set> set = Collections.singleton(Boolean.TRUE);
CLASS_SINGLETON_SET = set.getClass();
CLASS_UNMODIFIABLE_SET = Collections.unmodifiableSet(set).getClass();
List> list = Collections.singletonList(Boolean.TRUE);
CLASS_SINGLETON_LIST = list.getClass();
CLASS_UNMODIFIABLE_LIST = Collections.unmodifiableList(list).getClass();
Map,?> map = Collections.singletonMap("a", "b");
CLASS_SINGLETON_MAP = map.getClass();
CLASS_UNMODIFIABLE_MAP = Collections.unmodifiableMap(map).getClass();
}
public static JsonDeserializer> findForCollection(DeserializationContext ctxt,
JavaType type)
throws JsonMappingException
{
JavaUtilCollectionsConverter conv;
// 10-Jan-2017, tatu: Some types from `java.util.Collections`/`java.util.Arrays` need bit of help...
if (type.hasRawClass(CLASS_AS_ARRAYS_LIST)) {
conv = converter(TYPE_AS_LIST, type, List.class);
} else if (type.hasRawClass(CLASS_SINGLETON_LIST)) {
conv = converter(TYPE_SINGLETON_LIST, type, List.class);
} else if (type.hasRawClass(CLASS_SINGLETON_SET)) {
conv = converter(TYPE_SINGLETON_SET, type, Set.class);
} else if (type.hasRawClass(CLASS_UNMODIFIABLE_LIST)) {
conv = converter(TYPE_UNMODIFIABLE_LIST, type, List.class);
} else if (type.hasRawClass(CLASS_UNMODIFIABLE_SET)) {
conv = converter(TYPE_UNMODIFIABLE_SET, type, Set.class);
} else {
return null;
}
return new StdDelegatingDeserializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy