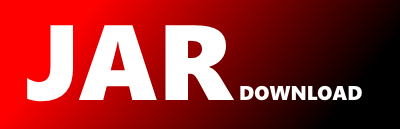
org.eclipse.jetty.server.ConnectionFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ehcache Show documentation
Show all versions of ehcache Show documentation
Ehcache is an open source, standards-based cache used to boost performance,
offload the database and simplify scalability. Ehcache is robust, proven and full-featured and
this has made it the most widely-used Java-based cache.
//
// ========================================================================
// Copyright (c) 1995-2018 Mort Bay Consulting Pty. Ltd.
// ------------------------------------------------------------------------
// All rights reserved. This program and the accompanying materials
// are made available under the terms of the Eclipse Public License v1.0
// and Apache License v2.0 which accompanies this distribution.
//
// The Eclipse Public License is available at
// http://www.eclipse.org/legal/epl-v10.html
//
// The Apache License v2.0 is available at
// http://www.opensource.org/licenses/apache2.0.php
//
// You may elect to redistribute this code under either of these licenses.
// ========================================================================
//
package org.eclipse.jetty.server;
import java.util.List;
import org.eclipse.jetty.http.BadMessageException;
import org.eclipse.jetty.http.HttpFields;
import org.eclipse.jetty.http.MetaData;
import org.eclipse.jetty.io.Connection;
import org.eclipse.jetty.io.EndPoint;
/**
* A Factory to create {@link Connection} instances for {@link Connector}s.
*
* A Connection factory is responsible for instantiating and configuring a {@link Connection} instance
* to handle an {@link EndPoint} accepted by a {@link Connector}.
*
* A ConnectionFactory has a protocol name that represents the protocol of the Connections
* created. Example of protocol names include:
*
* - http
- Creates a HTTP connection that can handle multiple versions of HTTP from 0.9 to 1.1
* - h2
- Creates a HTTP/2 connection that handles the HTTP/2 protocol
* - SSL-XYZ
- Create an SSL connection chained to a connection obtained from a connection factory
* with a protocol "XYZ".
* - SSL-http
- Create an SSL connection chained to a HTTP connection (aka https)
* - SSL-ALPN
- Create an SSL connection chained to a ALPN connection, that uses a negotiation with
* the client to determine the next protocol.
*
*/
public interface ConnectionFactory
{
/* ------------------------------------------------------------ */
/**
* @return A string representing the primary protocol name.
*/
public String getProtocol();
/* ------------------------------------------------------------ */
/**
* @return A list of alternative protocol names/versions including the primary protocol.
*/
public List getProtocols();
/**
* Creates a new {@link Connection} with the given parameters
* @param connector The {@link Connector} creating this connection
* @param endPoint the {@link EndPoint} associated with the connection
* @return a new {@link Connection}
*/
public Connection newConnection(Connector connector, EndPoint endPoint);
public interface Upgrading extends ConnectionFactory
{
/* ------------------------------------------------------------ */
/** Create a connection for an upgrade request.
* This is a variation of {@link #newConnection(Connector, EndPoint)} that can create (and/or customise)
* a connection for an upgrade request. Implementations may call {@link #newConnection(Connector, EndPoint)} or
* may construct the connection instance themselves.
*
* @param connector The connector to upgrade for.
* @param endPoint The endpoint of the connection.
* @param upgradeRequest The meta data of the upgrade request.
* @param responseFields The fields to be sent with the 101 response
* @return Null to indicate that request processing should continue normally without upgrading. A new connection instance to
* indicate that the upgrade should proceed.
* @throws BadMessageException Thrown to indicate the upgrade attempt was illegal and that a bad message response should be sent.
*/
public Connection upgradeConnection(Connector connector, EndPoint endPoint, MetaData.Request upgradeRequest,HttpFields responseFields) throws BadMessageException;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy