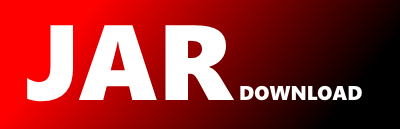
net.sf.expectit.SingleInput Maven / Gradle / Ivy
package net.sf.expectit;
/*
* #%L
* net.sf.expectit
* %%
* Copyright (C) 2014 Alexey Gavrilov
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import net.sf.expectit.filter.Filter;
import net.sf.expectit.matcher.Matcher;
import java.io.EOFException;
import java.io.IOException;
import java.io.InputStream;
import java.io.Writer;
import java.nio.ByteBuffer;
import java.nio.channels.Pipe;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.charset.Charset;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Future;
/**
* Represents a single output.
*/
class SingleInput {
public static final int BUFFER_SIZE = 1024;
private final InputStream input;
private final StringBuilder buffer;
private final Charset charset;
private final Writer echoOutput;
private final Filter[] filters;
private Future
© 2015 - 2025 Weber Informatics LLC | Privacy Policy