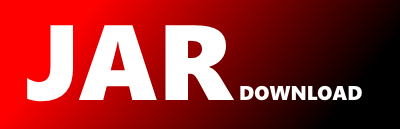
net.sf.ezmorph.MorpherRegistry Maven / Gradle / Ivy
/*
* Copyright 2006 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.sf.ezmorph;
import java.io.Serializable;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import net.sf.ezmorph.object.IdentityObjectMorpher;
/**
* Convenient class that manages Morphers.
* A MorpherRehistry manages a group of Morphers. A Morpher will always be
* associated with a target class, it is possible to have several Morphers
* registered for a target class, if this is the case, the first Morpher will be
* used when performing a conversion and no specific Morpher is selected in
* advance.
* {@link MorphUtils} may be used to register standard Morphers for primitive
* types and primitive wrappers, as well as arrays of those types.
*
* @author Andres Almiray
*/
public class MorpherRegistry implements Serializable
{
private static final long serialVersionUID = -4805239625300200760L;
private Map morphers = new HashMap();
public MorpherRegistry()
{
}
/**
* Deregisters all morphers.
*/
public void clear()
{
morphers.clear();
}
/**
* Deregister the specified Morpher.
* The registry will remove the target Class
from the morphers
* Map if it has no other registered morphers.
*
* @param morpher the target Morpher to remove
*/
public void deregisterMorpher( Morpher morpher )
{
List registered = (List) morphers.get( morpher.morphsTo() );
if( registered != null && !registered.isEmpty() ){
registered.remove( morpher );
if( registered.isEmpty() ){
morphers.remove( morpher.morphsTo() );
}
}
}
/**
* Returns a morpher for clazz
.
* If several morphers are found for that class, it returns the first. If no
* Morpher is found it will return the IdentityObjectMorpher.
*
* @param clazz the target class for which a Morpher may be associated
*/
public Morpher getMorpherFor( Class clazz )
{
List registered = (List) morphers.get( clazz );
if( registered == null || registered.isEmpty() ){
// no morpher registered for clazz
return IdentityObjectMorpher.getInstance();
}else{
return (Morpher) registered.get( 0 );
}
}
/**
* Returns all morphers for clazz
.
* If no Morphers are found it will return an array containing the
* IdentityObjectMorpher.
*
* @param clazz the target class for which a Morpher or Morphers may be
* associated
*/
public Morpher[] getMorphersFor( Class clazz )
{
List registered = (List) morphers.get( clazz );
if( registered == null || registered.isEmpty() ){
// no morphers registered for clazz
return new Morpher[] { IdentityObjectMorpher.getInstance() };
}else{
Morpher[] morphs = new Morpher[registered.size()];
int k = 0;
for( Iterator i = registered.iterator(); i.hasNext(); ){
morphs[k++] = (Morpher) i.next();
}
return morphs;
}
}
/**
* Morphs and object to the specified target class.
* This method uses reflection to invoke primitive Morphers and Morphers that
* do not implement ObjectMorpher.
*
* @param target the target class to morph to
* @param value the value to morph
* @return an instance of the target class if a suitable Morpher was found
* @throws MorphException if an error occurs during the conversion
*/
public Object morph( Class target, Object value )
{
Morpher morpher = getMorpherFor( target );
if( morpher instanceof ObjectMorpher ){
return ((ObjectMorpher) morpher).morph( value );
}else{
try{
Method morphMethod = morpher.getClass()
.getDeclaredMethod( "morph", new Class[] { Object.class } );
return morphMethod.invoke( morpher, new Object[] { value } );
}
catch( Exception e ){
throw new MorphException( e );
}
}
}
/**
* Register a Morpher for a target Class
.
* The target class is the class this Morpher morphs to. If there are another
* morphers registered to that class, it will be appended to a List.
*
* @param morpher a Morpher to register. The method morphsTo()
* is used to associate the Morpher to a target Class
*/
public void registerMorpher( Morpher morpher )
{
List registered = (List) morphers.get( morpher.morphsTo() );
if( registered == null ){
registered = new ArrayList();
morphers.put( morpher.morphsTo(), registered );
}
if( !registered.contains( morpher ) ){
registered.add( morpher );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy