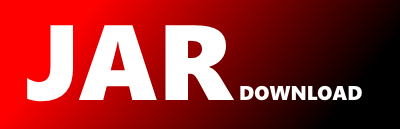
net.sf.filePiper.gui.GuiPipelineEnvironment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.gui;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.OutputStream;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.swing.JOptionPane;
import javax.swing.SwingUtilities;
import javax.swing.Timer;
import net.sf.sfac.gui.cmp.ConsoleDialog;
import net.sf.sfac.gui.ExceptionDialog;
import org.apache.log4j.Logger;
import net.sf.filePiper.model.BasicPipeLineEnvironment;
import net.sf.filePiper.model.FileProcessor;
import net.sf.filePiper.model.Pipeline;
import net.sf.filePiper.model.PipelineEnvironment;
public class GuiPipelineEnvironment implements PipelineEnvironment {
private static final Logger log = Logger.getLogger(GuiPipelineEnvironment.class);
private Pipeline pipeline;
private BasicPipeLineEnvironment asynchEnvironment;
private PiperMainPanel mainPane;
private Timer guiUpdateTimer;
private Map processBars;
private Boolean inconditionalOverwrite = null;
private ConsoleDialog console;
public GuiPipelineEnvironment(Pipeline processPipeline, PiperMainPanel mainPanel) {
pipeline = processPipeline;
mainPane = mainPanel;
processBars = new HashMap();
List processors = pipeline.getProcessors();
List procBars = mainPane.getProcessorStatus();
int len = procBars.size();
for (int i = 0; i < len; i++) {
processBars.put(processors.get(i), procBars.get(i));
}
asynchEnvironment = new BasicPipeLineEnvironment(processPipeline);
}
public void startUpdate() {
if (guiUpdateTimer != null) stopUpdate();
guiUpdateTimer = new Timer(333, new ActionListener() {
public void actionPerformed(ActionEvent newE) {
updateGui();
}
});
guiUpdateTimer.start();
}
public void stopUpdate() {
if (guiUpdateTimer != null) {
guiUpdateTimer.stop();
guiUpdateTimer = null;
if (asynchEnvironment.isErrored()) {
Exception ex = asynchEnvironment.getException();
ExceptionDialog.showExceptionDialog(mainPane, "Processing error", "Processing aborted by exception\n"
+ ex, ex);
}
}
updateGui();
mainPane.finished();
}
void updateGui() {
if (log.isDebugEnabled()) log.debug("--- GUI Update --- running=" + isRunning() + ", aborted=" + isAborted());
mainPane.getSourceStatus().setStatus(getSourceBarText());
for (FileProcessor processor : pipeline.getProcessors()) {
StatusBar bar = processBars.get(processor);
bar.setStatus(processor.getStatusMessage());
}
mainPane.getDestinationStatus().setStatus(getDestinationBarText());
}
private String getSourceBarText() {
return getText("Processing", "processed", asynchEnvironment.getCurrentScannedDirectory(), asynchEnvironment
.getCurrentInputFile(), asynchEnvironment.getInputFileCount(), 0, false);
}
private String getDestinationBarText() {
return getText("Writing", "written", null, asynchEnvironment.getCurrentOutputFile(), asynchEnvironment
.getOutputFileCount(), asynchEnvironment.getSkippedFileCount(), asynchEnvironment.isOutputToConsole());
}
private String getText(String actionNameOngoing, String actionNameDone, File scanning, File fil, int fileCount,
int skippedCount, boolean toConsole) {
boolean running = asynchEnvironment.isRunning();
StringBuffer sb = new StringBuffer();
if (running) {
if (fileCount == 0) {
if (skippedCount == 0) {
if (scanning != null) {
sb.append("Scanning dir: ");
sb.append(scanning.getAbsolutePath());
} else {
sb.append("Starting ...");
}
} else {
sb.append(skippedCount);
sb.append(" skipped");
}
} else {
if (scanning != null) {
sb.append("Scanning dir: ");
sb.append(scanning.getAbsolutePath());
} else {
sb.append(actionNameOngoing);
sb.append(" file ");
if (fileCount > 1) {
sb.append("[");
sb.append(fileCount);
sb.append("] ");
}
if (toConsole) sb.append(" to console");
else
sb.append(fil);
if (skippedCount > 0) {
sb.append(" (");
sb.append(skippedCount);
sb.append(" skipped)");
}
}
}
} else {
if (asynchEnvironment.isAborted()) {
sb.append("File processing aborted !");
} else if (asynchEnvironment.isErrored()) {
sb.append("File processing stopped by exception: " + asynchEnvironment.getException());
} else {
if (fileCount == 0) {
sb.append("No file ");
} else if (fileCount == 1) {
sb.append("File ");
if (!toConsole) {
sb.append(fil);
sb.append(" ");
}
} else {
sb.append(fileCount);
sb.append(" files ");
}
sb.append(actionNameDone);
if (toConsole) sb.append(" to console");
sb.append(".");
if (skippedCount > 0) {
sb.append(" (");
sb.append(skippedCount);
sb.append(" skipped)");
}
}
}
return sb.toString();
}
private void asynchStopUpdates() {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
stopUpdate();
}
});
}
// ------------------ PipelineEnvironment interface implementation ------------------------------------
public void startProcessing() {
inconditionalOverwrite = null;
asynchEnvironment.startProcessing();
}
public void abortProcessing() {
asynchEnvironment.abortProcessing();
}
public void finished(Exception e) {
asynchEnvironment.finished(e);
asynchStopUpdates();
}
public File getCurrentInputFile() {
return asynchEnvironment.getCurrentInputFile();
}
public File getCurrentOutputFile() {
return asynchEnvironment.getCurrentOutputFile();
}
public void outputtingToFile(File dest) {
asynchEnvironment.outputtingToFile(dest);
}
public void scanningDirectory(File dir) {
asynchEnvironment.scanningDirectory(dir);
}
public void processInputFile(File src) {
asynchEnvironment.processInputFile(src);
}
public boolean shouldContinue() {
return asynchEnvironment.shouldContinue();
}
public boolean canOverwriteFile(File existingFile) {
if (inconditionalOverwrite != null) {
return inconditionalOverwrite.booleanValue();
}
int res = JOptionPane.showOptionDialog(mainPane, "File " + existingFile.getAbsolutePath()
+ "\naleady exists\nOverwrite ?", "File exists", JOptionPane.YES_NO_CANCEL_OPTION, JOptionPane.WARNING_MESSAGE,
null, new String[] { "Yes", "Yes to all", "No", "No to all", "Abort" }, "Yes");
switch (res) {
case 0:
// Yes
return true;
case 1:
// Yes to all
inconditionalOverwrite = Boolean.TRUE;
return true;
case 2:
// No
return false;
case 3:
// No to all
inconditionalOverwrite = Boolean.FALSE;
return false;
}
// abort
abortProcessing();
inconditionalOverwrite = Boolean.FALSE;
return false;
}
public void fileSkipped(File dest) {
asynchEnvironment.fileSkipped(dest);
}
void getConsoleFromMainPanel() {
assert (SwingUtilities.isEventDispatchThread());
console = mainPane.getConsole();
mainPane.setConsoleVisible(true);
}
public OutputStream getConsoleStream() {
if (console == null) {
try {
SwingUtilities.invokeAndWait(new Runnable() {
public void run() {
getConsoleFromMainPanel();
}
});
} catch (Exception e) {
throw new IllegalStateException("Unable to show console", e);
}
}
return console.getConsoleStream();
}
public boolean isRunning() {
return asynchEnvironment.isRunning();
}
public boolean isAborted() {
return asynchEnvironment.isAborted();
}
public boolean isErrored() {
return asynchEnvironment.isErrored();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy