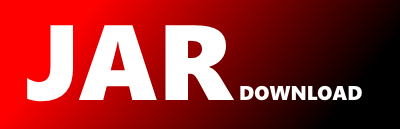
net.sf.filePiper.gui.PipeEndsEditor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.gui;
import java.awt.Container;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.util.Arrays;
import javax.swing.ButtonGroup;
import javax.swing.JButton;
import javax.swing.JComponent;
import javax.swing.JFileChooser;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.JTextField;
import net.sf.filePiper.model.FileProcessor;
import net.sf.filePiper.model.Pipeline;
import net.sf.sfac.file.FileType;
import net.sf.sfac.gui.editor.cmp.BaseObjectEditor;
import net.sf.sfac.gui.utils.FileDropListener;
import net.sf.sfac.setting.Settings;
import org.apache.log4j.Logger;
public class PipeEndsEditor extends BaseObjectEditor implements PipeEndsDescriptions {
private static final String DEFAULT_CHOOSER_SRC_DIRECTORY = "chooser.default.src.dir";
private static final String DEFAULT_CHOOSER_DEST_DIRECTORY = "chooser.default.dest.dir";
private static Logger log = Logger.getLogger(PipeEndsEditor.class);
private Pipeline pipeline;
private PiperMainPanel mainPane;
private boolean sourceMultiFile;
/** The output cardinality used when setting up the GUI */
private int currentOutputCardinality;
private int outputDestination;
private int outputNameChoice;
private Settings setts;
private JPanel sourcePane;
private JLabel sourceTypeLabel;
private JRadioButton sourceSingleChoice;
private JRadioButton sourceMultiChoice;
private JLabel sourceBaseLabel;
private JTextField sourceBase;
private TextfieldFileDropAdapter sourceBaseDropAdapter;
private JButton sourceHelper;
private JLabel includePatternLabel;
private JTextField includePattern;
private JLabel excludePatternLabel;
private JTextField excludePattern;
private JPanel destinationPane;
private JLabel outputDestinationLabel;
private JRadioButton fileDestination;
private JRadioButton consoleDestination;
private JLabel outputNameTypeLabel;
private JRadioButton currentNameChoice;
private JRadioButton proposedNameChoice;
private JRadioButton newNameChoice;
private JLabel destinationBaseLabel;
private JTextField destinationBase;
private TextfieldFileDropAdapter destinationBaseDropAdapter;
private JButton destinationHelper;
public PipeEndsEditor(Settings settgns, PiperMainPanel mainPanel) {
setts = settgns;
mainPane = mainPanel;
}
public JComponent getSourcePanel() {
buildGui();
return sourcePane;
}
public JComponent getDestinationPanel() {
buildGui();
return destinationPane;
}
private void buildGui() {
if (sourcePane == null) {
createSourcePane();
createDestinationPane();
synchronizeEditableState();
updateGui();
}
}
private void createSourcePane() {
sourcePane = new JPanel(new GridBagLayout());
// line 0: type (single or multiple)
sourceTypeLabel = new JLabel("Multiplicity");
sourceTypeLabel.setToolTipText(SOURCE_TYPE_DESCRIPTION);
ButtonGroup radios = new ButtonGroup();
sourceSingleChoice = new JRadioButton("Single");
sourceSingleChoice.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
setMultiFile(false);
}
});
radios.add(sourceSingleChoice);
sourceMultiChoice = new JRadioButton("Multiple");
sourceMultiChoice.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
setMultiFile(true);
}
});
radios.add(sourceMultiChoice);
sourcePane.add(sourceTypeLabel, new GridBagConstraints(0, 0, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(0, 10, 0, 4), 0, 0));
sourcePane.add(sourceSingleChoice, new GridBagConstraints(1, 0, 1, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.NONE, new Insets(0, 0, 0, 4), 0, 0));
sourcePane.add(sourceMultiChoice, new GridBagConstraints(2, 0, 1, 1, 1.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.NONE, new Insets(0, 0, 0, 0), 0, 0));
// line 1: file or directory
sourceBaseLabel = new JLabel("Input file");
sourceBaseLabel.setToolTipText(BASE_DIRECTORY_DESCRIPTION);
sourceBase = new JTextField(20);
sourceBaseDropAdapter = new TextfieldFileDropAdapter(sourceBase);
sourceHelper = new JButton("...");
sourceHelper.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent ev) {
displayHelper(true);
}
});
sourcePane.add(sourceBaseLabel, new GridBagConstraints(0, 1, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(2, 10, 0, 4), 0, 0));
sourcePane.add(sourceBase, new GridBagConstraints(1, 1, 2, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.HORIZONTAL, new Insets(2, 0, 0, 4), 0, 0));
sourcePane.add(sourceHelper, new GridBagConstraints(3, 1, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(2, 0, 0, 10), 0, 0));
// line 2: file includes pattern
includePatternLabel = new JLabel("Includes");
includePatternLabel.setToolTipText(INCLUDES_DESCRIPTION);
includePattern = new JTextField(20);
sourcePane.add(includePatternLabel, new GridBagConstraints(0, 2, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(2, 10, 0, 4), 0, 0));
sourcePane.add(includePattern, new GridBagConstraints(1, 2, 3, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.HORIZONTAL, new Insets(2, 0, 0, 10), 0, 0));
// line 3: file excludes pattern
excludePatternLabel = new JLabel("Excludes");
excludePatternLabel.setToolTipText(EXCLUDES_DESCRIPTION);
excludePattern = new JTextField(20);
sourcePane.add(excludePatternLabel, new GridBagConstraints(0, 3, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(2, 10, 0, 4), 0, 0));
sourcePane.add(excludePattern, new GridBagConstraints(1, 3, 3, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.HORIZONTAL, new Insets(2, 0, 0, 10), 0, 0));
}
private void createDestinationPane() {
destinationPane = new JPanel(new GridBagLayout());
// line 0: output destination (file or console)
outputDestinationLabel = new JLabel("Output destination");
outputDestinationLabel.setToolTipText(OUTPUT_DESTINATION_DESCRIPTION);
ButtonGroup destinationRadios = new ButtonGroup();
fileDestination = new JRadioButton("File");
fileDestination.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
setOutputDestination(Pipeline.OUTPUT_TO_FILE);
}
});
destinationRadios.add(fileDestination);
consoleDestination = new JRadioButton("Console");
consoleDestination.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
setOutputDestination(Pipeline.OUTPUT_TO_CONSOLE);
}
});
destinationRadios.add(consoleDestination);
destinationPane.add(outputDestinationLabel, new GridBagConstraints(0, 0, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(0, 10, 0, 4), 0, 0));
destinationPane.add(fileDestination, new GridBagConstraints(1, 0, 1, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.NONE, new Insets(0, 0, 0, 4), 0, 0));
destinationPane.add(consoleDestination, new GridBagConstraints(2, 0, 1, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.NONE, new Insets(0, 0, 0, 0), 0, 0));
// line 1: new name type (current, proposed or new)
outputNameTypeLabel = new JLabel("Output file name");
outputNameTypeLabel.setToolTipText(OUTPUT_FILE_NAME_DESCRIPTION);
ButtonGroup radios = new ButtonGroup();
currentNameChoice = new JRadioButton("Current");
currentNameChoice.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
setOutputNameChoice(Pipeline.OUTPUT_NAME_CURRENT);
}
});
radios.add(currentNameChoice);
proposedNameChoice = new JRadioButton("Proposed");
proposedNameChoice.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
setOutputNameChoice(Pipeline.OUTPUT_NAME_PROPOSED);
}
});
radios.add(proposedNameChoice);
newNameChoice = new JRadioButton("New");
newNameChoice.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
setOutputNameChoice(Pipeline.OUTPUT_NAME_NEW);
}
});
radios.add(newNameChoice);
destinationPane.add(outputNameTypeLabel, new GridBagConstraints(0, 1, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(0, 10, 0, 4), 0, 0));
destinationPane.add(currentNameChoice, new GridBagConstraints(1, 1, 1, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.NONE, new Insets(0, 0, 0, 4), 0, 0));
destinationPane.add(proposedNameChoice, new GridBagConstraints(2, 1, 1, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.NONE, new Insets(0, 0, 0, 0), 0, 0));
destinationPane.add(newNameChoice, new GridBagConstraints(3, 1, 1, 1, 1.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.NONE, new Insets(0, 0, 0, 0), 0, 0));
// line 2: file or directory
destinationBaseLabel = new JLabel("Output file");
destinationBaseLabel.setToolTipText(OUTPUT_FILE_DESCRIPTION);
destinationBase = new JTextField(20);
destinationBaseDropAdapter = new TextfieldFileDropAdapter(destinationBase);
destinationBaseDropAdapter.setDirectoryOnly(true);
destinationHelper = new JButton("...");
destinationHelper.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent ev) {
displayHelper(false);
}
});
destinationPane.add(destinationBaseLabel, new GridBagConstraints(0, 2, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(2, 10, 0, 4), 0, 0));
destinationPane.add(destinationBase, new GridBagConstraints(1, 2, 3, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.HORIZONTAL, new Insets(2, 0, 0, 4), 0, 0));
destinationPane.add(destinationHelper, new GridBagConstraints(4, 2, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(2, 0, 0, 10), 0, 0));
}
void setOutputDestination(int newOutputDestination) {
if (outputDestination != newOutputDestination) {
outputDestination = newOutputDestination;
updateGui();
}
}
void setMultiFile(boolean multi) {
if (sourceMultiFile != multi) {
sourceMultiFile = multi;
if (pipeline != null) pipeline.setSourceMultiFile(multi);
mainPane.checkCardinality();
updateGui();
}
}
void setOutputNameChoice(int newOutputNameChoice) {
if (outputNameChoice != newOutputNameChoice) {
outputNameChoice = newOutputNameChoice;
updateGui();
}
}
private void updateGui() {
if (sourcePane != null) {
boolean cmpEditable = isEditable();
boolean cmpEnabled = isEnabled();
// source
if (sourceMultiFile) sourceMultiChoice.setSelected(true);
else sourceSingleChoice.setSelected(true);
sourceBaseLabel.setText(sourceMultiFile ? "Base directory" : "Input file");
sourceBaseLabel.setToolTipText(sourceMultiFile ? BASE_DIRECTORY_DESCRIPTION : INPUT_FILE_DESCRIPTION);
sourceBaseDropAdapter.setDirectoryOnly(sourceMultiFile);
includePatternLabel.setVisible(sourceMultiFile);
excludePatternLabel.setVisible(sourceMultiFile);
includePattern.setVisible(sourceMultiFile);
excludePattern.setVisible(sourceMultiFile);
sourceBase.setEditable(cmpEditable);
includePattern.setEditable(cmpEditable);
excludePattern.setEditable(cmpEditable);
sourceBase.setEnabled(cmpEnabled);
includePattern.setEnabled(cmpEnabled);
excludePattern.setEnabled(cmpEnabled);
sourceSingleChoice.setEnabled(cmpEditable && cmpEnabled);
sourceMultiChoice.setEnabled(cmpEditable && cmpEnabled);
sourceHelper.setEnabled(cmpEditable && cmpEnabled);
// destination
currentOutputCardinality = pipeline.getOutputCardinality();
boolean outputToFile = outputDestination == Pipeline.OUTPUT_TO_FILE;
boolean outputIsUsed = currentOutputCardinality > FileProcessor.NONE;
boolean destinationMultiple = currentOutputCardinality == FileProcessor.MANY;
if (outputToFile) fileDestination.setSelected(true);
else consoleDestination.setSelected(true);
if (outputNameChoice == Pipeline.OUTPUT_NAME_CURRENT) currentNameChoice.setSelected(true);
else if (outputNameChoice == Pipeline.OUTPUT_NAME_PROPOSED) proposedNameChoice.setSelected(true);
else if (outputNameChoice == Pipeline.OUTPUT_NAME_NEW) {
if (destinationMultiple) {
outputNameChoice = Pipeline.OUTPUT_NAME_PROPOSED;
proposedNameChoice.setSelected(true);
} else {
newNameChoice.setSelected(true);
}
}
boolean showDestinationField = outputToFile
&& (destinationMultiple || (outputNameChoice == Pipeline.OUTPUT_NAME_NEW));
outputNameTypeLabel.setVisible(outputToFile);
currentNameChoice.setVisible(outputToFile);
proposedNameChoice.setVisible(outputToFile);
newNameChoice.setVisible(outputToFile);
destinationBaseLabel.setText(destinationMultiple ? "Target directory" : "Output file");
destinationBaseLabel.setToolTipText(destinationMultiple ? TARGET_DIRECTORY_DESCRIPTION : OUTPUT_FILE_DESCRIPTION);
destinationBaseLabel.setVisible(showDestinationField);
destinationBase.setVisible(showDestinationField);
destinationHelper.setVisible(showDestinationField);
fileDestination.setEnabled(outputIsUsed && cmpEditable && cmpEnabled);
consoleDestination.setEnabled(outputIsUsed && cmpEditable && cmpEnabled);
destinationBase.setEditable(outputToFile && outputIsUsed && cmpEditable);
destinationBase.setEnabled(outputToFile && outputIsUsed && cmpEnabled);
destinationHelper.setEnabled(outputToFile && outputIsUsed && cmpEnabled && cmpEnabled);
currentNameChoice.setEnabled(outputToFile && outputIsUsed && cmpEditable && cmpEnabled);
proposedNameChoice.setEnabled(outputToFile && outputIsUsed && cmpEditable && cmpEnabled);
newNameChoice.setEnabled(outputToFile && outputIsUsed && (!destinationMultiple) && cmpEditable && cmpEnabled);
sourcePane.revalidate();
destinationPane.revalidate();
Container parent = sourcePane.getParent();
if (parent != null) {
parent.invalidate();
parent.repaint();
}
}
}
void displayHelper(boolean forSource) {
// create & configure file chooser
JFileChooser chooser = new JFileChooser();
chooser.setMultiSelectionEnabled(false);
chooser.setFileSelectionMode(sourceMultiFile ? JFileChooser.DIRECTORIES_ONLY : JFileChooser.FILES_ONLY);
chooser.setDialogTitle(sourceMultiFile ? "Select a directory" : "Select a file");
// set selection
String defaultDirKey = forSource ? DEFAULT_CHOOSER_SRC_DIRECTORY : DEFAULT_CHOOSER_DEST_DIRECTORY;
File currentFile = getFile(forSource ? sourceBase : destinationBase);
if ((currentFile != null) && sourceMultiFile && currentFile.isFile()) currentFile = currentFile.getParentFile();
if (currentFile == null) {
try {
String initialPath = setts.getFileProperty(defaultDirKey, null, true);
if (initialPath != null) chooser.setCurrentDirectory(new File(initialPath));
} catch (Exception e) {
log.warn("Cannot configure chooser current directory", e);
}
} else {
chooser.setSelectedFile(currentFile);
}
// show file chooser
JPanel parent = (forSource) ? sourcePane : destinationPane;
if (chooser.showOpenDialog(parent) == JFileChooser.APPROVE_OPTION) {
File selected = chooser.getSelectedFile();
String path = (selected == null) ? "" : selected.getAbsolutePath();
try {
setts.setFileProperty(defaultDirKey, chooser.getCurrentDirectory().getAbsolutePath());
} catch (Exception e) {
log.warn("Cannot persist chooser current directory", e);
}
if (forSource) sourceBase.setText(path);
else destinationBase.setText(path);
}
}
@Override
protected void synchronizeEditableState() {
updateGui();
}
@Override
public void edit(Object objToEdit) {
pipeline = (Pipeline) objToEdit;
super.edit(objToEdit);
}
@Override
public Object getEditedObject() {
return pipeline;
}
public JComponent getEditorComponent() {
// not supposed to be called
return getSourcePanel();
}
public void updateModel() {
if (log.isDebugEnabled()) log.debug("<<< Update model of pipeline: " + pipeline);
if ((pipeline != null) && (sourcePane != null)) {
pipeline.setSourceMultiFile(sourceMultiFile);
pipeline.setOutputDestination(outputDestination);
pipeline.setOutputNameChoice(outputNameChoice);
pipeline.setSource(getFile(sourceBase));
pipeline.setIncludesPattern(includePattern.getText());
pipeline.setExcludesPattern(excludePattern.getText());
pipeline.setOutputFile(getFile(destinationBase));
}
}
private File getFile(JTextField field) {
String text = field.getText();
if ((text == null) || text.trim().equals("")) return null;
return new File(text);
}
private String getString(File fil) {
return (fil == null) ? "" : fil.getAbsolutePath();
}
public void updateView() {
if (sourcePane != null) {
if (log.isDebugEnabled()) log.debug(">>> Update view for pipeline: " + pipeline);
if (pipeline == null) {
setEnabled(false);
sourceBase.setText("");
includePattern.setText("");
excludePattern.setText("");
destinationBase.setText("");
} else {
setEnabled(true);
setMultiFile(pipeline.isSourceMultiFile());
setOutputDestination(pipeline.getOutputDestination());
setOutputNameChoice(pipeline.getOutputNameChoice());
sourceBase.setText(getString(pipeline.getSource()));
includePattern.setText(pipeline.getIncludesPattern());
excludePattern.setText(pipeline.getExcludesPattern());
destinationBase.setText(getString(pipeline.getOutputFile()));
}
}
}
public void checkCardinality() {
if ((sourcePane != null) && (pipeline != null) && (currentOutputCardinality != pipeline.getOutputCardinality())) {
updateGui();
}
}
public JComponent getObjectComponent() {
// not supposed to be called
return getSourcePanel();
}
private static class TextfieldFileDropAdapter implements FileDropListener.FileDropTarget {
private JTextField tf;
private boolean directoryOnly;
public TextfieldFileDropAdapter(JTextField field) {
tf = field;
FileDropListener lis = new FileDropListener(this, (FileType[]) null);
lis.registerToComponent(tf);
}
public void setDirectoryOnly(boolean newDirectoryOnly) {
directoryOnly = newDirectoryOnly;
}
public void filesDropped(File[] files, int dropAction) {
if ((files != null) && (files.length > 0)) {
System.out.println(">>> " + files.length + " file dropped = " + Arrays.toString(files));
fileDropped(files[0]);
}
}
private void fileDropped(File f) {
if ((f != null) && directoryOnly && !f.isDirectory()) {
f = f.getParentFile();
}
if (f != null) {
tf.setText(f.getAbsolutePath());
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy