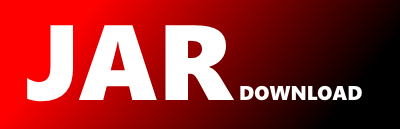
net.sf.filePiper.gui.SizeAndUnitEditor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.gui;
import java.awt.Color;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ButtonGroup;
import javax.swing.JComponent;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.JTextField;
import net.sf.sfac.gui.editor.cmp.BaseObjectEditor;
import net.sf.filePiper.processors.SizeAndUnit;
public class SizeAndUnitEditor extends BaseObjectEditor {
// private Settings setts;
private String description;
private String sizeLabelText;
private int units;
private SizeAndUnit editedObj;
private JPanel editorPane;
private JLabel descriptionLabel;
private JLabel unitTypeLabel;
private JLabel sizeLabel;
private JLabel sizeUnitsLabel;
private JRadioButton lineUnitChoice;
private JRadioButton byteUnitChoice;
private JTextField sizeTextField;
public SizeAndUnitEditor(String descript, String text) {
description = descript;
sizeLabelText = text;
}
private void buildGui() {
if (editorPane == null) {
editorPane = new JPanel(new GridBagLayout());
// line 0: description
String desc = getDescription();
if (desc != null) {
descriptionLabel = new JLabel(desc);
descriptionLabel.setForeground(Color.DARK_GRAY);
editorPane.add(descriptionLabel, new GridBagConstraints(0, 0, 4, 1, 0.0, 0.0, GridBagConstraints.CENTER,
GridBagConstraints.NONE, new Insets(0, 0, 0, 0), 0, 0));
}
// line 1: units (line or byte)
unitTypeLabel = new JLabel("Units");
ButtonGroup radios = new ButtonGroup();
lineUnitChoice = new JRadioButton("Lines");
lineUnitChoice.setSelected(units == SizeAndUnit.UNIT_LINE);
lineUnitChoice.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
setUnitChoice(SizeAndUnit.UNIT_LINE);
}
});
radios.add(lineUnitChoice);
byteUnitChoice = new JRadioButton("Bytes");
byteUnitChoice.setSelected(units == SizeAndUnit.UNIT_BYTE);
byteUnitChoice.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
setUnitChoice(SizeAndUnit.UNIT_BYTE);
}
});
radios.add(byteUnitChoice);
editorPane.add(unitTypeLabel, new GridBagConstraints(0, 1, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(0, 8, 0, 4), 0, 0));
editorPane.add(lineUnitChoice, new GridBagConstraints(1, 1, 1, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.NONE, new Insets(0, 0, 0, 4), 0, 0));
editorPane.add(byteUnitChoice, new GridBagConstraints(2, 1, 1, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.NONE, new Insets(0, 0, 0, 0), 0, 0));
// line 2: file or directory
sizeLabel = new JLabel(getSizeLabelText());
sizeTextField = new JTextField(8);
sizeUnitsLabel = new JLabel((units == SizeAndUnit.UNIT_BYTE) ? "bytes" : "lines");
editorPane.add(sizeLabel, new GridBagConstraints(0, 2, 1, 1, 0.0, 0.0, GridBagConstraints.EAST,
GridBagConstraints.NONE, new Insets(2, 8, 0, 4), 0, 0));
editorPane.add(sizeTextField, new GridBagConstraints(1, 2, 2, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.HORIZONTAL, new Insets(2, 0, 0, 4), 0, 0));
editorPane.add(sizeUnitsLabel, new GridBagConstraints(3, 2, 1, 1, 0.0, 0.0, GridBagConstraints.WEST,
GridBagConstraints.NONE, new Insets(2, 0, 0, 0), 0, 0));
synchronizeEditableState();
}
}
void setUnitChoice(int newUnitChoice) {
if (units != newUnitChoice) {
units = newUnitChoice;
if ((byteUnitChoice != null) && (units == SizeAndUnit.UNIT_BYTE) && !byteUnitChoice.isSelected()) {
byteUnitChoice.setSelected(true);
}
if ((lineUnitChoice != null) && (units == SizeAndUnit.UNIT_LINE) && !lineUnitChoice.isSelected()) {
lineUnitChoice.setSelected(true);
}
if (sizeUnitsLabel != null) sizeUnitsLabel.setText((units == SizeAndUnit.UNIT_BYTE) ? "bytes" : "lines");
}
}
@Override
protected void synchronizeEditableState() {
sizeTextField.setEditable(isEditable());
sizeTextField.setEnabled(isEnabled());
lineUnitChoice.setEnabled(isEditable() && isEnabled());
byteUnitChoice.setEnabled(isEditable() && isEnabled());
}
public void edit(Object objToEdit) {
editedObj = (SizeAndUnit) objToEdit;
}
public Object getEditedObject() {
return editedObj;
}
public JComponent getEditorComponent() {
buildGui();
return editorPane;
}
public boolean isModified() {
return false;
}
public void updateModel() {
if ((editedObj != null) && (editorPane != null)) {
int size = 0;
String text = sizeTextField.getText();
if (text != null) {
text = text.trim();
if (!text.equals("")) {
try {
size = Integer.parseInt(text);
} catch (Exception e) {
size = 0;
sizeTextField.setText("0");
}
}
}
editedObj.setSize(size);
editedObj.setUnits(units);
}
}
public void updateView() {
if (editorPane != null) {
if (editedObj == null) {
setEnabled(false);
sizeTextField.setText("");
} else {
setEnabled(true);
sizeTextField.setText(String.valueOf(editedObj.getSize()));
setUnitChoice(editedObj.getUnits());
}
}
}
public String getDescription() {
return description;
}
public void setDescription(String newDescription) {
description = newDescription;
}
public String getSizeLabelText() {
return sizeLabelText;
}
public void setSizeLabelText(String newSizeLabelText) {
sizeLabelText = newSizeLabelText;
}
public JComponent getObjectComponent() {
return getEditorComponent();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy