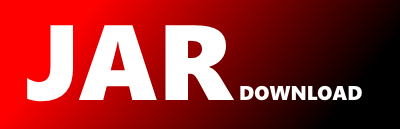
net.sf.filePiper.gui.borders.FileProcessorBorder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.gui.borders;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.Insets;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.List;
import javax.swing.ComboBoxModel;
import javax.swing.JComboBox;
import net.sf.sfac.gui.layout.MultiBorder;
import net.sf.sfac.gui.layout.MultiBorderConstraints;
import net.sf.sfac.gui.layout.MultiBorderLayout;
import net.sf.sfac.gui.framework.SharedResources;
/**
* Border allowing to choose the file processor.
*
* @see MultiBorderLayout
* @see MultiBorderConstraints
* @author Olivier Berlanger
*/
public class FileProcessorBorder extends MultiBorder {
private JComboBox combo;
private RolloverButton addButton;
private RolloverButton removeButton;
private List listeners;
/**
* Creates an ExpandBorder using an EtchedBorder.
*/
public FileProcessorBorder(ComboBoxModel allChoices) {
this(new JComboBox(allChoices));
}
/**
* Creates an ExpandBorder using an EtchedBorder.
*/
public FileProcessorBorder(JComboBox choicesCombo) {
combo = choicesCombo;
addButton = new RolloverButton(SharedResources.getIcon("borderAdd.gif"));
addButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
addPerformed();
}
});
removeButton = new RolloverButton(SharedResources.getIcon("borderRemove.gif"));
removeButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
removePerformed();
}
});
}
public void removePerformed() {
if (listeners != null) {
for (Listener lis : listeners) {
lis.removeActionPerformed(this);
}
}
}
public void addPerformed() {
if (listeners != null) {
for (Listener lis : listeners) {
lis.addActionPerformed(this);
}
}
}
public void setAddButtonEnabled(boolean enabled) {
addButton.setEnabled(enabled);
}
public boolean isAddButtonEnabled() {
return addButton.isEnabled();
}
public void setRemoveButtonEnabled(boolean enabled) {
removeButton.setEnabled(enabled);
}
public boolean isRemoveButtonEnabled() {
return removeButton.isEnabled();
}
public static interface Listener {
public void removeActionPerformed(FileProcessorBorder source);
public void addActionPerformed(FileProcessorBorder source);
}
/**
* Check that the expand button is well added at the end of the list of border components. If not, add It.
*/
protected void registerExtraComponents() {
registerExtraComponent(removeButton);
registerExtraComponent(addButton);
registerExtraComponent(combo);
}
protected void layoutExtraComponents(Container cont) {
// combo-box (top-left side of border)
Dimension comboDim = combo.getPreferredSize();
Insets borderInsets = getUpdatedInsets(cont);
int posX = x + 20;
int posY = y + ((borderInsets.top - comboDim.height) / 2);
combo.setBounds(posX, posY, comboDim.width, comboDim.height);
// buttons (top-right side of border)
Dimension buttonDim = addButton.getPreferredSize();
posX = x + width - 10 - buttonDim.width;
posY = y + ((borderInsets.top - buttonDim.height) / 2);
removeButton.setBounds(posX, posY, buttonDim.width, buttonDim.height);
posX = posX - 4 - buttonDim.width;
addButton.setBounds(posX, posY, buttonDim.width, buttonDim.height);
}
protected void updateInsets(Insets insetToUpdate) {
Dimension comboDim = combo.getPreferredSize();
if (insetToUpdate.top < comboDim.height) insetToUpdate.top = comboDim.height;
Dimension buttonDim = addButton.getPreferredSize();
if (insetToUpdate.top < buttonDim.height) insetToUpdate.top = buttonDim.height;
if (insetToUpdate.left < 5) insetToUpdate.left = 5;
if (insetToUpdate.right < 5) insetToUpdate.right = 5;
if (insetToUpdate.bottom < 5) insetToUpdate.bottom = 5;
}
public void addBorderListsner(Listener lis) {
if (listeners == null) listeners = new ArrayList();
listeners.add(lis);
}
public void removeBorderListsner(Listener lis) {
if (listeners != null) listeners.remove(lis);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy