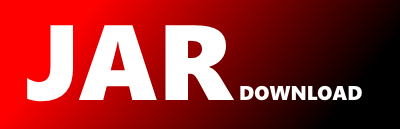
net.sf.filePiper.model.BasicPipeLineEnvironment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.model;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.util.List;
public class BasicPipeLineEnvironment implements PipelineEnvironment {
private Pipeline pipeline;
private boolean running;
private boolean aborted;
private File scannedDir;
private File currentSource;
private File currentDestination;
private int fileProcessedCount;
private int outputFileCount;
private int skippedFileCount;
private boolean outputToConsole;
private Exception ex;
public BasicPipeLineEnvironment(Pipeline processPipeline) {
pipeline = processPipeline;
}
public void dumpStatus() {
System.out.println("" + fileProcessedCount + " files processed");
System.out.println("" + outputFileCount + " files written (" + skippedFileCount + " skipped)");
List processors = pipeline.getProcessors();
for (FileProcessor processor : processors) {
System.out.print(" * " + processor + ": " + processor.getStatusMessage());
}
if (isAborted()) {
System.out.println(" ------- Aborted by user");
} else if (isErrored()) {
System.out.println(" ------- Aborted on exception:");
ex.printStackTrace(System.out);
}
}
public void abortProcessing() {
aborted = true;
}
// -------------------- Getters of PipelineEnvironment state ----------------------------------------
public boolean isRunning() {
return running;
}
public boolean isAborted() {
return aborted;
}
public boolean isErrored() {
return ex != null;
}
public int getInputFileCount() {
return fileProcessedCount;
}
public int getOutputFileCount() {
return outputFileCount;
}
public int getSkippedFileCount() {
return skippedFileCount;
}
public boolean isOutputToConsole() {
return outputToConsole;
}
public Exception getException() {
return ex;
}
// -------------------- FileProcessorEnvironment interface implementation ----------------------------------------
public void startProcessing() {
running = true;
aborted = false;
fileProcessedCount = 0;
outputFileCount = 0;
skippedFileCount = 0;
currentSource = null;
currentDestination = null;
outputToConsole = false;
}
public void scanningDirectory(File dir) {
scannedDir = dir;
}
public void processInputFile(File newSrc) {
fileProcessedCount++;
currentSource = newSrc;
scannedDir = null;
}
public void outputtingToFile(File newDest) {
outputFileCount++;
currentDestination = newDest;
outputToConsole = (newDest == null);
}
public File getCurrentScannedDirectory() {
return scannedDir;
}
public File getCurrentInputFile() {
return currentSource;
}
public File getCurrentOutputFile() {
return currentDestination;
}
public void finished(Exception e) {
running = false;
if ((e != null) && (ex == null)) ex = e;
}
public boolean shouldContinue() {
return !aborted && (ex == null);
}
/**
* Always overwrite by default.
*/
public boolean canOverwriteFile(File newFil) {
return true;
}
public void fileSkipped(File dest) {
skippedFileCount++;
}
public OutputStream getConsoleStream() {
return new ConsoleStream();
}
static class ConsoleStream extends OutputStream {
@Override
public void write(int b) throws IOException {
System.out.write(b);
}
@Override
public void write(byte[] b) throws IOException {
System.out.write(b);
}
@Override
public void write(byte[] b, int start, int len) throws IOException {
System.out.write(b, start, len);
}
@Override
public void close() {
System.out.flush();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy