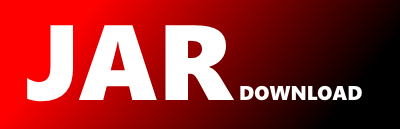
net.sf.filePiper.model.FileMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.model;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Pattern;
import net.sf.sfac.file.FilePathUtils;
import net.sf.sfac.file.InvalidPathException;
import org.apache.log4j.Logger;
public class FileMatcher {
static Logger log = Logger.getLogger(FileMatcher.class);
private FilePathUtils baseDir;
private List includes;
private List excludes;
private List directoryIncludes;
private List directoryExcludes;
public FileMatcher(File sourceBaseDir, String includesPattern, String excludesPattern) {
baseDir = new FilePathUtils(sourceBaseDir);
includesPattern = (includesPattern == null) ? null : includesPattern.replace('\\', '/');
excludesPattern = (excludesPattern == null) ? null : excludesPattern.replace('\\', '/');
includes = getProcessedPatterns(includesPattern, true);
excludes = getProcessedPatterns(excludesPattern, false);
directoryIncludes = getDirectoryIncludes(includesPattern);
directoryExcludes = getDirectoryExcludes(excludesPattern);
}
public boolean matchFile(File fil) {
return matches(fil, includes, excludes);
}
public boolean matchDirectoryTree(File fil) {
return matches(fil, directoryIncludes, directoryExcludes);
}
private boolean matches(File fil, List incl, List excl) {
if ((incl == null) && (excl == null)) return true;
String filePath;
try {
filePath = baseDir.getRelativeFilePath(fil.getAbsolutePath());
} catch (InvalidPathException e) {
throw new IllegalArgumentException("Inavlid path: " + fil.getAbsolutePath(), e);
}
if (filePath.equals(".")) return true;
boolean included = patternMatches(filePath, incl, true);
if (included) {
return !patternMatches(filePath, excl, false);
}
return false;
}
private List getDirectoryIncludes(String includesAntPattern) {
List result = null;
for (String antPattern : tokenizePattern(includesAntPattern)) {
antPattern = normalize(antPattern);
if (antPattern.startsWith("**")) {
// if at least one include pattern start with '**', we cannot build includes at directory level
result = null;
break;
} else {
int includeEnd = -1;
int doubleStarIndex = antPattern.indexOf("**");
boolean expand = false;
if (doubleStarIndex > 0) {
includeEnd = doubleStarIndex - 1;
expand = true;
} else {
int lastSlashIndex = antPattern.lastIndexOf("/");
if (lastSlashIndex > 0) includeEnd = lastSlashIndex;
}
if (includeEnd > 0) {
String[] pathElements = antPattern.substring(0, includeEnd).split("/");
StringBuffer pathBuffer = new StringBuffer();
for (String el : pathElements) {
if (pathBuffer.length() > 0) pathBuffer.append("/");
pathBuffer.append(el);
String path = pathBuffer.toString();
Pattern pat = getProcessedPattern(path, false);
if (pat != null) {
log.info("Directory include pattern = " + path + " = " + pat);
if (result == null) result = new ArrayList();
result.add(pat);
}
}
if (expand) {
String path = pathBuffer.toString();
Pattern pat = getProcessedPattern(path, true);
if (pat != null) {
log.info("Directory include pattern = " + path + "/** = " + pat);
if (result == null) result = new ArrayList();
result.add(pat);
}
}
}
}
}
return result;
}
private List getDirectoryExcludes(String excludesAntPattern) {
List result = null;
for (String antPattern : tokenizePattern(excludesAntPattern)) {
antPattern = normalize(antPattern);
if (antPattern.endsWith("/**/*")) {
String subPattern = antPattern.substring(0, antPattern.length() - 5);
Pattern pat = getProcessedPattern(subPattern, false);
if (pat != null) {
log.info("Directory exclude pattern = " + subPattern + " = " + pat);
if (result == null) result = new ArrayList();
result.add(pat);
}
}
}
return result;
}
private String normalize(String antPattern) {
if (antPattern.endsWith("**")) return antPattern + "/*";
if (antPattern.endsWith("/")) return antPattern + "**/*";
return antPattern;
}
private List getProcessedPatterns(String antPatternList, boolean include) {
List result = null;
for (String antPattern : tokenizePattern(antPatternList)) {
antPattern = normalize(antPattern);
Pattern pat = getProcessedPattern(antPattern, false);
if (pat != null) {
log.info("File " + (include ? "include" : "exclude") + " pattern = " + antPattern + " = " + pat);
if (result == null) result = new ArrayList();
result.add(pat);
}
}
return result;
}
private String[] tokenizePattern(String antPattern) {
String[] tokens = new String[0];
if (antPattern != null) {
antPattern = antPattern.trim();
if (!antPattern.equals("")) {
tokens = antPattern.split("\\s*,\\s*");
}
}
return tokens;
}
private Pattern getProcessedPattern(String antPattern, boolean expanded) {
if (antPattern == null) return null;
antPattern = antPattern.trim();
if (antPattern.equals("")) return null;
// build the regular expression corresponding to the given ant-like pattern
StringBuffer regExpr = new StringBuffer();
int len = antPattern.length();
String separatorPattern = (File.separatorChar == '\\') ? "\\\\" : File.separator;
for (int i = 0; i < len; i++) {
char ch = antPattern.charAt(i);
switch (ch) {
case '[':
case ']':
case '(':
case ')':
case '.':
regExpr.append("\\");
regExpr.append(ch);
break;
case '/':
regExpr.append(separatorPattern);
break;
case '?':
regExpr.append("[^");
regExpr.append(separatorPattern);
regExpr.append("]");
break;
case '*':
if (antPattern.regionMatches(i, "**/", 0, 3)) {
regExpr.append("(.*");
regExpr.append(separatorPattern);
regExpr.append(")*");
i += 2;
} else {
regExpr.append("[^");
regExpr.append(separatorPattern);
regExpr.append("]*");
}
break;
default:
regExpr.append(ch);
}
}
if (expanded) regExpr.append(".*");
return Pattern.compile(regExpr.toString());
}
private boolean patternMatches(String filePath, List patterns, boolean defaultMatch) {
boolean match = defaultMatch;
if ((patterns != null) && (patterns.size() > 0)) {
match = false;
for (Pattern includePattern : patterns) {
if (includePattern.matcher(filePath).matches()) {
match = true;
break;
}
}
}
return match;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy