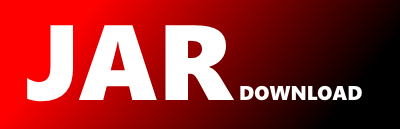
net.sf.filePiper.model.OneToOneByteFileProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.model;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import net.sf.sfac.editor.EditorConfig;
import net.sf.sfac.gui.editor.EditorFactory;
import net.sf.sfac.gui.editor.ObjectEditor;
import net.sf.sfac.gui.editor.cmp.ReadOnlyObjectEditor;
import net.sf.sfac.setting.Settings;
import org.apache.log4j.Logger;
/**
* Base implementation for FileProcessor with ONE to ONE cardinality and processing byte streams.
* There is only 3 remaining abstract methods very simple to implement.
*
* @author BEROL
*/
public abstract class OneToOneByteFileProcessor implements FileProcessor {
private Logger log = Logger.getLogger(OneToOneByteFileProcessor.class);
private Settings setts;
private InputFileInfo currentInfo;
private StatusHolder holder = new StatusHolder();
public void init(Settings sett) {
setts = sett;
}
protected Settings getSettings() {
return setts;
}
protected InputFileInfo getCurrentInputFileInfo() {
return currentInfo;
}
protected void setCurrentInputFileInfo(InputFileInfo info) {
currentInfo = info;
}
public void startBatch(FileProcessorEnvironment env) {
holder.reset(ExecutionPhase.STARTING);
}
public void endBatch(FileProcessorEnvironment env) {
holder.setCurrentPhase(env.getCurrentPhase());
}
public int getOutputCardinality(int inputCardinality) {
return inputCardinality;
}
public void process(InputStream is, InputFileInfo info, FileProcessorEnvironment env) throws IOException {
try {
inputFileStarted();
setCurrentInputFileInfo(info);
setProposedFilePath(info);
OutputStream os = env.getOutputStream(info);
process(is, os, env);
if (log.isDebugEnabled()) log.debug("Processor " + this + " closes output stream");
os.close();
setCurrentInputFileInfo(null);
} catch (Exception e) {
holder.setCurrentPhase(ExecutionPhase.ERRORED);
IOException ioe = new IOException("Exception while processing input " + info);
ioe.initCause(e);
throw ioe;
}
}
protected void setProposedFilePath(InputFileInfo info) {
info.addProposedNameSuffix(getProposedNameSuffix());
String proposedExtension = getProposedExtension(info);
if (proposedExtension != null) {
info.setProposedExtension(proposedExtension);
}
}
protected String getProposedExtension(InputFileInfo info) {
return null;
}
public ObjectEditor getEditor() {
if (EditorFactory.containsEditorAnnotations(getClass())) {
ObjectEditor ed = EditorFactory.getInstance().createEditor(getClass());
if (!getClass().isAnnotationPresent(EditorConfig.class)) {
ed.setProperty(ObjectEditor.EDITOR_LABEL_PROPERTY, null);
ed.setProperty(ObjectEditor.EDITOR_DESCRIPTION_PROPERTY, getProcessorDescription());
}
return ed;
} else {
return new ReadOnlyObjectEditor(getProcessorDescription());
}
}
public String getProcessorDescription() {
return getProcessorName() + " processor";
}
public String getStatusMessage() {
return holder.getStatusMessage();
}
public void inputFileStarted() {
holder.inputFileStarted();
}
public void linesProcessed(int nbrLineProcessed) {
holder.linesProcessed(nbrLineProcessed);
}
public void bytesProcessed(int nbrByteProcessed) {
holder.bytesProcessed(nbrByteProcessed);
}
// -------------------------- Method to implement or override -----------------------
public abstract String getProcessorName();
public abstract String getProposedNameSuffix();
public abstract void process(InputStream is, OutputStream os, FileProcessorEnvironment env) throws Exception;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy