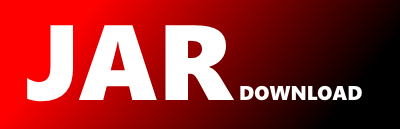
net.sf.filePiper.model.PipelineStart Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.model;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import org.apache.log4j.Logger;
/**
* First component of the pipeline.
* This components scan the file system for input files and inject them in the pipeline.
*
* @author BEROL
*/
public class PipelineStart {
private static Logger log = Logger.getLogger(PipelineStart.class);
private Pipeline pipeline;
public PipelineStart(Pipeline thePipeline) {
pipeline = thePipeline;
}
public void processInputFiles(PipeComponent firstProcessor, PipelineEnvironment reporter) throws IOException {
File source = pipeline.getSource();
if (pipeline.isSourceMultiFile()) {
if (source == null) throw new IllegalArgumentException("You must define a source directory");
if (!source.exists())
throw new IOException("The source directory '" + source.getAbsolutePath() + "' does not exist!");
FileMatcher matcher = new FileMatcher(source, pipeline.getIncludesPattern(), pipeline.getExcludesPattern());
processInputFiles(source, matcher, firstProcessor, reporter);
} else {
if (source == null) throw new IllegalArgumentException("You must define a source file");
if (!source.exists()) throw new IOException("The source file '" + source.getAbsolutePath() + "' does not exist!");
if (!source.isFile()) throw new IOException("The source '" + source.getAbsolutePath() + "' must be a file!");
processFile(source, firstProcessor, reporter);
}
}
private void processInputFiles(File dir, FileMatcher matcher, PipeComponent firstProcessor, PipelineEnvironment reporter)
throws IOException {
if (!reporter.shouldContinue()) return;
if (!dir.exists()) throw new IOException("Base directory '" + dir.getAbsolutePath() + "' must exist");
if (!dir.isDirectory()) throw new IOException("Base directory '" + dir.getAbsolutePath() + "' must be a directory");
reporter.scanningDirectory(dir);
if (matcher.matchDirectoryTree(dir)) {
File[] subs = dir.listFiles();
for (File fil : subs) {
if (!reporter.shouldContinue()) return;
if (fil.isDirectory()) {
processInputFiles(fil, matcher, firstProcessor, reporter);
} else if (fil.isFile() && (fil.length() > 0)) {
if (matcher.matchFile(fil)) {
processFile(fil, firstProcessor, reporter);
}
}
}
} else {
if (log.isDebugEnabled()) log.debug("Skip directory tree: " + dir);
}
}
private void processFile(File fil, PipeComponent firstProcessor, PipelineEnvironment reporter) throws IOException {
if (fil.length() == 0) return;
reporter.processInputFile(fil);
File source = pipeline.getSource();
InputFileInfo info = new InputFileInfo(pipeline.isSourceMultiFile() ? source : null, fil);
if (log.isDebugEnabled()) log.debug("----------- Open file line = " + info);
InputStream is = new FileInputStream(fil);
firstProcessor.processInputStream(is, info);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy