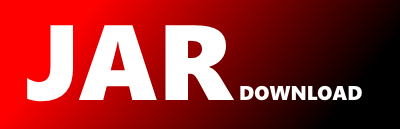
net.sf.filePiper.model.StatusHolder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.model;
/**
* Processor of a file.
*
* @author berol
*/
public class StatusHolder {
private ExecutionPhase currentPhase;
private int inputFileCount;
private int outputFileCount;
private long lineCount;
private long byteCount;
private volatile String lastMessage;
public StatusHolder() {
currentPhase = ExecutionPhase.NONE;
}
public void reset(ExecutionPhase newPhase) {
if (newPhase == null) throw new IllegalArgumentException("Phase cannot be null, use ExecutionPhase.NONE instead");
currentPhase = newPhase;
inputFileCount = 0;
outputFileCount = 0;
lineCount = 0;
byteCount = 0;
lastMessage = null;
}
public String getStatusMessage() {
String message = lastMessage;
if (message == null) {
switch (currentPhase) {
case STARTING:
message = getStartingMessage();
break;
case RUNNING:
message = getRunningMessage();
break;
case DONE:
message = getDoneMessage();
break;
case ABORTED:
message = getAbortedMessage();
break;
case ERRORED:
message = getErrorMessage();
break;
default:
message = "";
}
lastMessage = message;
}
return message;
}
public void setCurrentPhase(ExecutionPhase newPhase) {
if (newPhase == null) throw new IllegalArgumentException("Phase cannot be null, use ExecutionPhase.NONE instead");
lastMessage = null;
currentPhase = newPhase;
}
/**
* Report that a new input stream is being processed.
*/
public void inputFileStarted() {
runningInfoReceived();
inputFileCount++;
}
/**
* Report that a new output stream is being processed.
* Note: the default implementation of getStatusMessage() doesn't use this number. It is present for subclasses that might
* use it.
*/
public void outputFileStarted() {
runningInfoReceived();
outputFileCount++;
}
/**
* Report that some lines of the input stream have been processed.
* Each FileProcessor can choose to work in 'line' or 'byte' unit.
*
* @param nbrLineProcessed
* number of lines that were processed.
*/
public void linesProcessed(long nbrLineProcessed) {
runningInfoReceived();
lineCount += nbrLineProcessed;
}
/**
* Report that some bytes of the input stream have been processed.
* Each FileProcessor can choose to work in 'line' or 'byte' unit.
*
* @param nbrByteProcessed
* number of lines that were processed.
*/
public void bytesProcessed(long nbrByteProcessed) {
runningInfoReceived();
byteCount += nbrByteProcessed;
}
// ------------------------ getters ------------------------------------------------
private void runningInfoReceived() {
if ((currentPhase == ExecutionPhase.NONE) || (currentPhase == ExecutionPhase.STARTING)) {
currentPhase = ExecutionPhase.RUNNING;
}
lastMessage = null;
}
public ExecutionPhase getCurrentPhase() {
return currentPhase;
}
public int getInputFileCount() {
return inputFileCount;
}
public int getOutputFileCount() {
return outputFileCount;
}
public long getLineCount() {
return lineCount;
}
public long getByteCount() {
return byteCount;
}
// ------------------------ implementation ------------------------------------------------
protected String getStartingMessage() {
return "Waiting for input...";
}
protected String getRunningMessage() {
StringBuilder sb = new StringBuilder();
appendLineOrByteCount(byteCount, lineCount, sb);
sb.append(" processed from ");
appendCount(inputFileCount, "file", sb);
sb.append("...");
return sb.toString();
}
protected String getDoneMessage() {
StringBuilder sb = new StringBuilder();
appendLineOrByteCount(byteCount, lineCount, sb);
sb.append(" done from ");
appendCount(inputFileCount, "file", sb);
if (inputFileCount > 1) {
sb.append(" (");
appendLineOrByteCount(byteCount / inputFileCount, lineCount / inputFileCount, sb);
sb.append(" per file)");
}
sb.append(".");
return sb.toString();
}
protected String getAbortedMessage() {
return "Aborted after: " + getDoneMessage();
}
protected String getErrorMessage() {
return "Error after: " + getDoneMessage();
}
protected void appendLineOrByteCount(long bytes, long lines, StringBuilder sb) {
if (bytes > 0) appendCount(bytes, "byte", sb);
else appendCount(lines, "line", sb);
}
protected void appendCount(long count, String entityName, StringBuilder sb) {
sb.append(count);
sb.append(' ');
sb.append(entityName);
if (count > 1) sb.append("s");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy