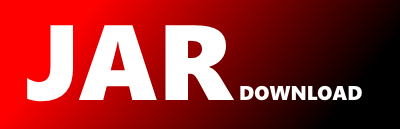
net.sf.filePiper.model.ToolModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.model;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.Iterator;
import java.util.List;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import net.sf.sfac.setting.Settings;
import net.sf.sfac.setting.SubSettingsProxy;
import org.apache.log4j.Logger;
import sun.misc.Service;
public class ToolModel {
private static Logger log = Logger.getLogger(ToolModel.class);
private Settings sett;
private SubSettingsProxy pipelineSettings;
private Pipeline pipeline;
private List availableProcessors;
private List listeners;
public ToolModel(Settings setts) {
sett = setts;
pipelineSettings = new SubSettingsProxy(sett, "currentPipeline");
log.info("Initialize pipeline from settings");
pipeline = new Pipeline(pipelineSettings);
}
public Settings getSettings() {
return sett;
}
public Settings getPipelineSettings() {
return pipelineSettings;
}
public void updatePipelineSettings(Settings profile) {
pipelineSettings.clear();
pipelineSettings.copyValues(profile);
log.info("Copy pipeline settings from profile: " + profile.getStringProperty(SubSettingsProxy.SUB_SETTING_NAME, "???"));
pipeline.reset();
fireDataChanged();
}
public Pipeline getPipeline() {
return pipeline;
}
@SuppressWarnings("unchecked")
public List getAvailableProcessors() {
if (availableProcessors == null) {
availableProcessors = new ArrayList();
log.info("SPI retrieving of all FileProcessor");
Iterator plugInDefs = Service.providers(FileProcessor.class, getClass().getClassLoader());
while (plugInDefs.hasNext()) {
FileProcessor processor = plugInDefs.next();
log.info(" * FileProcessor = " + processor.getClass());
availableProcessors.add(processor);
}
Collections.sort(availableProcessors, new Comparator() {
public int compare(FileProcessor fp1, FileProcessor fp2) {
return fp1.getProcessorName().compareTo(fp2.getProcessorName());
}
});
}
return availableProcessors;
}
public FileProcessor getPrototype(FileProcessor fp) {
FileProcessor proto = null;
for (FileProcessor processor : getAvailableProcessors()) {
if (processor.getClass() == fp.getClass()) {
proto = processor;
break;
}
}
return proto;
}
public void addChangeListener(ChangeListener l) {
if (listeners == null) listeners = new ArrayList();
listeners.add(l);
}
public void removeChangeListener(ChangeListener l) {
if (listeners != null) listeners.remove(l);
}
public void fireDataChanged() {
if (listeners != null) {
ChangeEvent ev = new ChangeEvent(this);
for (ChangeListener l : listeners) {
l.stateChanged(ev);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy