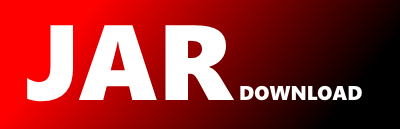
net.sf.filePiper.processors.BreakLinesProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.processors;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import net.sf.filePiper.model.FileProcessorEnvironment;
import net.sf.filePiper.model.OneToOneTextFileProcessor;
import net.sf.sfac.editor.EditorConfig;
import net.sf.sfac.setting.Settings;
import net.sf.sfac.utils.Comparison;
/**
* Processor replacing a pattern by line breaks.
*
* @author BEROL
*/
public class BreakLinesProcessor extends OneToOneTextFileProcessor {
private static final String BREAK_PATTERN = "break.pattern";
private static final String KEEP_BREAK_PATTERN = "break.keep.pattern";
private Settings sett;
@Override
public String getProcessorName() {
return "Break lines";
}
@Override
public String getProcessorDescription() {
return "Break lines when encountering a defined pattern.";
}
@EditorConfig(label = "Break at", index = 0)
public String getBreakString() {
return sett.getStringProperty(BREAK_PATTERN, "");
}
public void setBreakString(String searchString) {
sett.setStringProperty(BREAK_PATTERN, searchString);
}
@EditorConfig(label = "Keep break string", index = 1)
public boolean getKeepBreakString() {
return sett.getBooleanProperty(KEEP_BREAK_PATTERN, true);
}
public void setKeepBreakString(boolean newKeepBreakString) {
sett.setBooleanProperty(KEEP_BREAK_PATTERN, newKeepBreakString);
}
@Override
public void init(Settings settgns) {
sett = settgns;
}
@Override
public void process(BufferedReader in, BufferedWriter out, FileProcessorEnvironment env) throws Exception {
StringBuilder sb = new StringBuilder();
String breakString = getBreakString();
boolean keep = getKeepBreakString();
if (Comparison.isEmpty(breakString)) throw new IllegalStateException("You must define a break string");
int ch;
while ((ch = in.read()) >= 0) {
sb.append((char) ch);
if (endsWidth(sb, breakString)) {
int len = sb.length();
if (!keep) sb.setLength(len - breakString.length());
out.write(sb.toString());
out.newLine();
sb.setLength(0);
}
}
}
private boolean endsWidth(StringBuilder sb, String str) {
int strLen = str.length();
int bufferOffset = sb.length() - strLen;
if (bufferOffset < 0) return false;
for (int i = 0; i < strLen; i++) {
char ch = str.charAt(i);
if (ch != sb.charAt(bufferOffset + i)) {
return false;
}
}
return true;
}
@Override
public String getProposedNameSuffix() {
return "break";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy