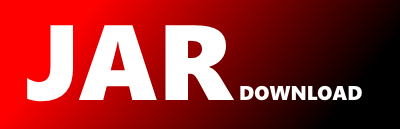
net.sf.filePiper.processors.ImageSizerProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.processors;
import java.awt.Graphics;
import java.awt.image.BufferedImage;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.imageio.ImageIO;
import net.sf.filePiper.model.FileProcessorEnvironment;
import net.sf.filePiper.model.InputFileInfo;
import net.sf.filePiper.model.OneToOneByteFileProcessor;
import net.sf.sfac.editor.EditorConfig;
import org.apache.log4j.Logger;
/**
* Processor resizing an JPEG image file.
*
* @author BEROL
*/
public class ImageSizerProcessor extends OneToOneByteFileProcessor {
public enum ImageFormat {
jpg, png, gif
}
private static final String TARGET_SIZE = "image.target.size";
private static final String TARGET_FORMAT = "image.target.format";
private static Logger log = Logger.getLogger(ImageSizerProcessor.class);
@Override
public String getProcessorName() {
return "Image Sizer";
}
@Override
public String getProcessorDescription() {
return "Resize images so that the largest image dimension
don't exceed the given size (in pixels).";
}
@EditorConfig(label = "Image bigger size (pixels)")
public int getTargetSize() {
return getSettings().getIntProperty(TARGET_SIZE, 400);
}
public void setTargetSize(int newSize) {
getSettings().setIntProperty(TARGET_SIZE, newSize);
}
@EditorConfig(label = "Target image format")
public ImageFormat getTargetFormat() {
return getSettings().getEnumProperty(TARGET_FORMAT, ImageFormat.jpg);
}
public void setTargetFormat(ImageFormat newFormat) {
getSettings().setEnumProperty(TARGET_FORMAT, newFormat);
}
@Override
public String getProposedNameSuffix() {
return "sized";
}
@Override
protected void setProposedFilePath(InputFileInfo info) {
super.setProposedFilePath(info);
info.setProposedExtension(getTargetFormat().name());
}
@Override
public void process(InputStream is, OutputStream os, FileProcessorEnvironment env) throws IOException {
BufferedImage img = ImageIO.read(is);
int width = img.getWidth(null);
int height = img.getHeight(null);
int targetSize = getTargetSize();
// rough estimation of the image size in bytes (it's just for visual reporting)
bytesProcessed(width * height);
int targetWidth;
int targetHeight;
if (!env.shouldContinue()) return;
if ((width > targetSize) || (height > targetSize)) {
if (width > height) {
targetWidth = targetSize;
targetHeight = (int) ((((double) targetSize) / width) * height);
} else {
targetWidth = (int) ((((double) targetSize) / height) * width);
targetHeight = targetSize;
}
if (log.isDebugEnabled())
log.debug("Resize image " + getCurrentInputFileInfo().getInputRelativePath() + " from " + width + " x "
+ height + " to " + targetWidth + " x " + targetHeight);
BufferedImage targetImg = new BufferedImage(targetWidth, targetHeight, BufferedImage.TYPE_INT_RGB);
Graphics g = targetImg.getGraphics();
g.drawImage(img, 0, 0, targetWidth, targetHeight, 0, 0, width, height, null);
if (!env.shouldContinue()) return;
ImageIO.write(targetImg, getTargetFormat().name(), os);
} else {
if (log.isDebugEnabled())
log.debug("No resize for image " + getCurrentInputFileInfo().getInputRelativePath() + " at " + width + " x "
+ height);
if (!env.shouldContinue()) return;
ImageIO.write(img, getTargetFormat().name(), os);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy