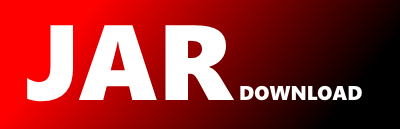
net.sf.filePiper.processors.ReplaceProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.processors;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.IOException;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import net.sf.filePiper.model.FileProcessorEnvironment;
import net.sf.filePiper.model.OneToOneTextFileProcessor;
import net.sf.sfac.editor.EditorConfig;
/**
* Processor replacing an string by another in the input file.
*
* @author BEROL
*/
public class ReplaceProcessor extends OneToOneTextFileProcessor {
private static final String REPLACED_STRING = "replaced.string";
private static final String REPLACEMENT_STRING = "replacement.string";
private static final String REPLACEMENT_TYPE = "replacement.by.line";
private static final String SEARCH_METHOD = "search.method";
private static enum SearchMethod {
LITTERAL("Litteral"), CASE_INSENSITIVE("Case Insensitive"), REG_EXPR("Regular Expr.");
private String text;
SearchMethod(String txt) {
text = txt;
}
@Override
public String toString() {
return text;
}
}
@Override
public String getProcessorName() {
return "Replace all";
}
@Override
public String getProcessorDescription() {
return "Replace all occurences of a string by another
(allows regular experssions like in String.replaceAll(...))";
}
@EditorConfig(label = "Find", index = 0)
public String getReplaced() {
return getSettings().getStringProperty(REPLACED_STRING, "");
}
public void setReplaced(String newReplaced) {
getSettings().setStringProperty(REPLACED_STRING, newReplaced);
}
@EditorConfig(label = "Matching method", index = 1)
public SearchMethod getSearchMethod() {
return getSettings().getEnumProperty(SEARCH_METHOD, SearchMethod.LITTERAL);
}
public void setSearchMethod(SearchMethod method) {
getSettings().setEnumProperty(SEARCH_METHOD, method);
}
@EditorConfig(label = "Replace By", index = 2)
public String getReplacement() {
return getSettings().getStringProperty(REPLACEMENT_STRING, "");
}
public void setReplacement(String newReplacement) {
getSettings().setStringProperty(REPLACEMENT_STRING, newReplacement);
}
@EditorConfig(label = "Process line by line", index = 3)
public boolean getReplacementByLine() {
return getSettings().getBooleanProperty(REPLACEMENT_TYPE, true);
}
public void setReplacementByLine(boolean byLine) {
getSettings().setBooleanProperty(REPLACEMENT_TYPE, byLine);
}
@Override
public String getProposedNameSuffix() {
return "rplc";
}
private int getPatternFlags() {
switch (getSearchMethod()) {
case LITTERAL:
return Pattern.LITERAL;
case CASE_INSENSITIVE:
return Pattern.LITERAL | Pattern.CASE_INSENSITIVE;
default:
return 0;
}
}
@Override
public void process(BufferedReader in, BufferedWriter out, FileProcessorEnvironment env) throws IOException {
boolean regExp = (getSearchMethod() == SearchMethod.REG_EXPR);
Pattern replacedPattern = Pattern.compile(getReplaced(), getPatternFlags());
String replacement = getReplacement();
if (getReplacementByLine()) {
String line;
while (((line = in.readLine()) != null) && env.shouldContinue()) {
out.write(replace(line, replacedPattern, replacement, regExp));
out.newLine();
linesProcessed(1);
}
} else {
// Read the whole file in a string
// Remark: this will explode the RAM with very big files
char[] charBuffer = new char[1024];
StringBuilder sb = new StringBuilder();
int readCount;
while (((readCount = in.read(charBuffer)) > 0) && env.shouldContinue()) {
sb.append(charBuffer, 0, readCount);
}
String fileContent = sb.toString();
// replace and write to output
if (env.shouldContinue()) {
String replacedFileContent = replace(fileContent, replacedPattern, replacement, regExp);
out.write(replacedFileContent);
}
bytesProcessed(fileContent.length());
}
}
private String replace(String src, Pattern replacedPattern, String replacement, boolean regExp) {
if (regExp) {
return replacedPattern.matcher(src).replaceAll(replacement);
} else {
return replacedPattern.matcher(src).replaceAll(Matcher.quoteReplacement(replacement.toString()));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy