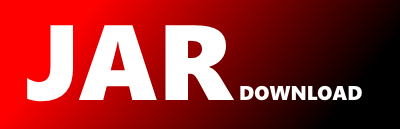
net.sf.filePiper.processors.SearchProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.processors;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import net.sf.filePiper.model.ExecutionPhase;
import net.sf.filePiper.model.FileProcessor;
import net.sf.filePiper.model.FileProcessorEnvironment;
import net.sf.filePiper.model.InputFileInfo;
import net.sf.filePiper.model.Pipeline;
import net.sf.filePiper.model.StatusHolder;
import net.sf.sfac.editor.EditorConfig;
import net.sf.sfac.gui.editor.EditorFactory;
import net.sf.sfac.gui.editor.ObjectEditor;
import net.sf.sfac.setting.Settings;
import net.sf.sfac.string.ReaderCharIterator;
import net.sf.sfac.string.StringCharIterator;
import net.sf.sfac.string.StringUtils;
/**
* Processor searching a string in input file(s) -- like grep.
* The output of this processor is the list of matches.
*
* @author BEROL
*/
public class SearchProcessor implements FileProcessor {
private static final String SEARCH_STRING = "search.string";
private static final String SEARCH_BY_LINE = "search.by.line";
private static final String SEARCH_METHOD = "search.method";
private static final String SEARCH_OUTPUT = "search.output";
private static enum SearchMethod {
LITTERAL("Litteral"), CASE_INSENSITIVE("Case Insensitive"), REG_EXPR("Regular Expr."), LENIENT("Lenient");
private String text;
SearchMethod(String txt) {
text = txt;
}
@Override
public String toString() {
return text;
}
}
private static enum OutputType {
REPORT("Report"), LINES("Lines"), FILE_NAMES("File Names");
private String text;
OutputType(String txt) {
text = txt;
}
@Override
public String toString() {
return text;
}
}
private Matcher m;
private Settings sett;
private BufferedWriter out;
private StatusHolder holder = new StatusHolder() {
@Override
protected String getRunningMessage() {
StringBuilder sb = new StringBuilder();
appendLineOrByteCount(getByteCount(), getLineCount(), sb);
sb.append(" processed from ");
appendCount(getInputFileCount(), "file", sb);
sb.append(" (");
appendCount(getOutputFileCount(), "file", sb);
sb.append(" found)...");
return sb.toString();
}
@Override
protected String getDoneMessage() {
StringBuilder sb = new StringBuilder();
appendLineOrByteCount(getByteCount(), getLineCount(), sb);
sb.append(" searched from ");
appendCount(getInputFileCount(), "file", sb);
sb.append(" (");
appendCount(getOutputFileCount(), "file", sb);
sb.append(" found).");
return sb.toString();
}
};
public String getProcessorName() {
return "Search for expression";
}
public String getProcessorDescription() {
return "Search a string in files and generate a text report of files/lines matched.";
}
@EditorConfig(label = "Find", index = 0)
public String getSearchString() {
return sett.getStringProperty(SEARCH_STRING, "");
}
public void setSearchString(String searchString) {
sett.setStringProperty(SEARCH_STRING, searchString);
}
@EditorConfig(label = "Search line by line", index = 2)
public boolean getSearchByLine() {
return sett.getBooleanProperty(SEARCH_BY_LINE, true);
}
public void setSearchByLine(boolean byLine) {
sett.setBooleanProperty(SEARCH_BY_LINE, byLine);
}
@EditorConfig(label = "Matching method", index = 1)
public SearchMethod getSearchMethod() {
return sett.getEnumProperty(SEARCH_METHOD, SearchMethod.LITTERAL);
}
public void setSearchMethod(SearchMethod method) {
sett.setEnumProperty(SEARCH_METHOD, method);
}
@EditorConfig(label = "Output Type", index = 3)
public OutputType getOutputType() {
return sett.getEnumProperty(SEARCH_OUTPUT, OutputType.REPORT);
}
public void setOutputType(OutputType type) {
sett.setEnumProperty(SEARCH_OUTPUT, type);
}
public void init(Settings settgns) {
sett = settgns;
}
public void startBatch(FileProcessorEnvironment env) throws IOException {
// reset temporary variables
m = null;
holder.reset(ExecutionPhase.STARTING);
// output file header
Pipeline line = env.getPipeline();
File dir = line.getSource();
if (dir.isFile()) dir = dir.getAbsoluteFile().getParentFile();
InputFileInfo info = new InputFileInfo(dir, new File(dir, "SearchResult.txt"));
OutputStream os = env.getOutputStream(info);
out = new BufferedWriter(new OutputStreamWriter(os));
if (getOutputType() == OutputType.REPORT) {
out.write("Search ");
out.write(getSearchMethod().toString());
out.write(" for: ");
out.write(getSearchString());
out.newLine();
out.write(getSearchByLine() ? "Line by line" : "In file as a whole");
out.newLine();
if (line.isSourceMultiFile()) {
out.write("In files below ");
out.write(dir.getAbsolutePath());
out.newLine();
String includes = line.getIncludesPattern();
if ((includes != null) && !includes.trim().equals("")) {
out.write("Including ");
out.write(includes);
out.newLine();
}
String excludes = line.getExcludesPattern();
if ((excludes != null) && !excludes.trim().equals("")) {
out.write("Excluding ");
out.write(excludes);
out.newLine();
}
} else {
out.write("In file ");
out.write(line.getSource().getAbsolutePath());
out.newLine();
}
out.write("---------------------------------------------------------------------------------");
out.newLine();
}
}
public void process(InputStream is, InputFileInfo info, FileProcessorEnvironment env) throws IOException {
BufferedReader in = new BufferedReader(new InputStreamReader(is));
process(in, info, env);
}
public void process(BufferedReader in, InputFileInfo info, FileProcessorEnvironment env) throws IOException {
holder.inputFileStarted();
if (getSearchByLine()) {
searchLineByLine(in, info, env);
} else {
searchWholeFile(in, info, env);
}
}
private void searchLineByLine(BufferedReader in, InputFileInfo info, FileProcessorEnvironment env) throws IOException {
String searchString = getSearchString();
SearchMethod method = getSearchMethod();
OutputType outType = getOutputType();
boolean firstMatch = true;
int lineCount = 0;
String line;
while (((line = in.readLine()) != null) && env.shouldContinue()) {
lineCount++;
boolean found = false;
switch (method) {
case LITTERAL:
found = line.contains(searchString);
break;
case CASE_INSENSITIVE:
found = StringUtils.matchString(searchString, new StringCharIterator(line), true);
break;
case REG_EXPR:
found = regExprContains(line, searchString);
break;
case LENIENT:
found = StringUtils.matchPattern(searchString, new StringCharIterator(line));
break;
default:
throw new IllegalStateException("Unimplemented search method: " + method);
}
if (found) {
if (firstMatch) {
holder.outputFileStarted();
if (outType == OutputType.REPORT) {
out.newLine();
out.write("File ");
out.write(info.getInput().getAbsolutePath());
out.newLine();
} else if (outType == OutputType.LINES) {
out.write(info.getInput().getAbsolutePath());
out.newLine();
}
}
if (outType == OutputType.REPORT) {
out.write(" at line ");
out.write(String.valueOf(lineCount));
out.write(": ");
out.write(line);
out.newLine();
} else if (outType == OutputType.LINES) {
out.write(line);
out.newLine();
}
out.flush();
firstMatch = false;
}
holder.linesProcessed(1);
}
}
private boolean regExprContains(String line, String searchString) {
if (m == null) {
Pattern p = Pattern.compile(searchString);
m = p.matcher(line);
} else {
m.reset(line);
}
return m.find();
}
private void searchWholeFile(BufferedReader in, InputFileInfo info, FileProcessorEnvironment env) throws IOException {
String searchString = getSearchString();
SearchMethod method = getSearchMethod();
OutputType outType = getOutputType();
if (outType == OutputType.LINES) {
throw new IllegalArgumentException("Output type 'Lines' is only available when searching line by line.");
}
boolean found = false;
ReaderCharIterator charIterator = null;
String contentString = null;
if (env.shouldContinue()) {
switch (method) {
case LITTERAL:
charIterator = new ReaderCharIterator(in);
found = StringUtils.matchString(searchString, charIterator, false);
break;
case CASE_INSENSITIVE:
charIterator = new ReaderCharIterator(in);
found = StringUtils.matchString(searchString, charIterator, true);
break;
case REG_EXPR:
contentString = getReaderContent(in);
found = regExprContains(contentString, searchString);
break;
case LENIENT:
charIterator = new ReaderCharIterator(in);
found = StringUtils.matchPattern(searchString, charIterator);
break;
default:
throw new IllegalStateException("Unimplemented search method: " + method);
}
}
if (charIterator != null) holder.bytesProcessed(charIterator.getProcessedCharCount());
if (contentString != null) holder.bytesProcessed(contentString.length());
if (found && env.shouldContinue()) {
holder.outputFileStarted();
if (outType == OutputType.REPORT) out.write("File ");
out.write(info.getInput().getAbsolutePath());
out.newLine();
out.flush();
}
}
private String getReaderContent(BufferedReader in) throws IOException {
// Rem: this will explode the RAM with very big files
char[] charBuffer = new char[1024];
StringBuffer sb = new StringBuffer();
int readCount;
while ((readCount = in.read(charBuffer)) > 0) {
sb.append(charBuffer, 0, readCount);
}
return sb.toString();
}
public void endBatch(FileProcessorEnvironment env) throws IOException {
if (getOutputType() == OutputType.REPORT) {
out.newLine();
out.write(" found ");
out.write(String.valueOf(holder.getOutputFileCount()));
out.write(" file");
if (holder.getOutputFileCount() > 1) out.write("s");
out.write(" out of ");
out.write(String.valueOf(holder.getInputFileCount()));
out.newLine();
}
out.close();
holder.setCurrentPhase(env.getCurrentPhase());
}
public String getStatusMessage() {
return holder.getStatusMessage();
}
public int getOutputCardinality(int inputCardinality) {
return ONE;
}
public ObjectEditor getEditor() {
ObjectEditor ed = EditorFactory.getInstance().createEditor(getClass());
ed.setProperty(ObjectEditor.EDITOR_LABEL_PROPERTY, null);
ed.setProperty(ObjectEditor.EDITOR_DESCRIPTION_PROPERTY, getProcessorDescription());
return ed;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy