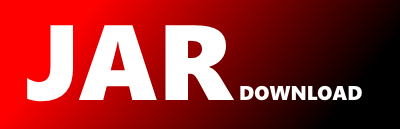
net.sf.filePiper.processors.XmlFormatProcessor_ Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-piper Show documentation
Show all versions of file-piper Show documentation
This project is a GUI utility for processing files. It allows selecting a set of source files and a pipeline
of processes to apply onto those files. The applications shows in a nice-looking user interface where you can
define profiles for your repetitive tasks.
It provides pre-defined processors doing usual file manipulation tasks like: Copy, Head, Tail, Chunk, Search, Replace, Zip, Unzip...
But the biggest value of this file processor tool is the ability to add easily custom file processors written in java.
The newest version!
package net.sf.filePiper.processors;
import java.io.InputStream;
import java.io.OutputStream;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import net.sf.filePiper.model.FileProcessorEnvironment;
import net.sf.filePiper.model.OneToOneByteFileProcessor;
import org.tinyWorkflow.utils.xml.serialize.StreamXMLSerializer;
import org.tinyWorkflow.utils.xml.serialize.XMLSerializer;
import org.xml.sax.Attributes;
import org.xml.sax.InputSource;
import org.xml.sax.Locator;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
import org.xml.sax.XMLReader;
import org.xml.sax.helpers.DefaultHandler;
/**
* Processor doing XML pretty formatting.
*
* @author BEROL
*/
public class XmlFormatProcessor extends OneToOneByteFileProcessor {
private XMLReader xReader;
public String getProcessorName() {
return "XML Format";
}
public String getProcessorDescription() {
return "Format & validate XML files";
}
public String getProposedNameSuffix() {
return "frmt";
}
@Override
public void process(InputStream is, OutputStream os, FileProcessorEnvironment env) throws Exception {
InputSource src = new InputSource(is);
StreamXMLSerializer ser = new StreamXMLSerializer(os);
PipeHandler handler = new PipeHandler(ser);
XMLReader reader = getXmlReader();
reader.setContentHandler(handler);
reader.setErrorHandler(handler);
reader.parse(src);
}
private XMLReader getXmlReader() throws SAXException, ParserConfigurationException {
if (xReader == null) {
SAXParserFactory parserFactory = SAXParserFactory.newInstance();
System.out.println("Use parser factory: " + parserFactory);
parserFactory.setNamespaceAware(true);
parserFactory.setValidating(false);
SAXParser parser = parserFactory.newSAXParser();
xReader = parser.getXMLReader();
}
return xReader;
}
static class PipeHandler extends DefaultHandler {
private XMLSerializer serializer;
private Locator saxLocator;
public PipeHandler(XMLSerializer ser) {
serializer = ser;
}
public void setDocumentLocator(Locator locator) {
saxLocator = locator;
}
public void startDocument() throws SAXException {
try {
serializer.startDocument();
} catch (Exception e) {
throw new SAXException("Cannot start document", e);
}
saxLocator = null;
}
public void endDocument() throws SAXException {
try {
serializer.endDocument();
} catch (Exception e) {
throw new SAXException("Cannot end document", e);
}
saxLocator = null;
}
public void startPrefixMapping(String prefix, String uri) throws SAXException {
try {
serializer.startPrefixMapping(prefix, uri);
} catch (Exception e) {
throw new SAXException("Cannot start prefix mapping xmlns:" + prefix + "='" + uri + "'", e);
}
}
public void endPrefixMapping(String prefix) throws SAXException {
}
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
try {
serializer.startElement(uri, localName);
int len = attributes.getLength();
for (int i = 0; i < len; i++) {
serializer.attribute(attributes.getURI(i), attributes.getLocalName(i), attributes.getValue(i));
}
} catch (Exception e) {
e.printStackTrace();
throw new SAXException("Cannot start element <" + localName + "> at " + saxLocator, e);
}
}
public void endElement(String uri, String localName, String qName) throws SAXException {
try {
serializer.endElement(uri, localName);
} catch (Exception e) {
throw new SAXException("Cannot end element <" + localName + "> at " + saxLocator, e);
}
}
public void error(SAXParseException e) throws SAXException {
throw e;
}
public void characters(char[] ch, int start, int length) throws SAXException {
try {
serializer.characters(new String(ch, start, length));
} catch (Exception e) {
throw new SAXException("Cannot serialize characters", e);
}
}
public void ignorableWhitespace(char ch[], int start, int length) throws SAXException {
try {
serializer.characters(new String(ch, start, length));
} catch (Exception e) {
throw new SAXException("Cannot serialize ignorable characters", e);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy