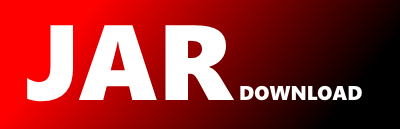
net.sf.flatpack.StreamingDataSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flatpack Show documentation
Show all versions of flatpack Show documentation
Simple Java delimited and fixed width file parser. Handles CSV, Excel CSV, Tab, Pipe delimiters, just to name a few.
Maps column positions in the file to user friendly names via XML. See FlatPack Feature List under News for complete feature list.
The newest version!
package net.sf.flatpack;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.Optional;
import java.util.Spliterator;
import java.util.Spliterators;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
/**
* New with jdk8, define stream() methods. You should
* start using this BUT note that it will only return VALID Records, any invalid row will be skipped.
*
* @author Benoit Xhenseval
* @since 3.4
*/
public interface StreamingDataSet extends RecordDataSet {
/**
* @since 4.0
* @return a stream of Records
*/
default Stream stream() {
return StreamSupport
.stream(Spliterators.spliteratorUnknownSize(spliterator(), Spliterator.ORDERED | Spliterator.NONNULL | Spliterator.IMMUTABLE), false);
}
/**
* @since 4.0
* @return a stream of Records
*/
default Stream parallelStream() {
return StreamSupport
.stream(Spliterators.spliteratorUnknownSize(spliterator(), Spliterator.ORDERED | Spliterator.NONNULL | Spliterator.IMMUTABLE), true);
}
default Iterator spliterator() {
return new Iterator() {
Optional nextData = Optional.empty();
@Override
public boolean hasNext() {
if (nextData.isPresent()) {
return true;
} else {
if (StreamingDataSet.this.next()) {
nextData = getRecord();
} else {
nextData = Optional.empty();
}
return nextData.isPresent();
}
}
@Override
public Record next() {
if (nextData.isPresent() || hasNext()) {
final Record line = nextData.isPresent() ? nextData.get() : null;
nextData = Optional.empty();
return line;
} else {
throw new NoSuchElementException();
}
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy