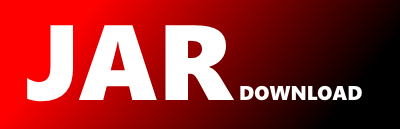
net.sf.gluebooster.java.booster.basic.container.BoostedHashSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.container;
import java.awt.event.ActionEvent;
import java.util.Collection;
import java.util.HashSet;
import net.sf.gluebooster.java.booster.basic.transformation.CallableByFactory;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.Callable;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.MultiListener;
import net.sf.gluebooster.java.booster.essentials.math.Condition;
/**
* A hash set with more functionality.
*
* @author CBauer
*
* @param
* the class of the elements of the hash set.
* @defaultParamText elementClass the class of the elements
*/
public class BoostedHashSet extends HashSet implements
Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy