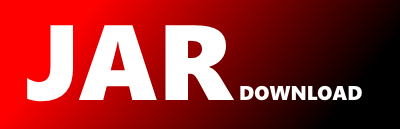
net.sf.gluebooster.java.booster.basic.container.IteratorOfContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.container;
import java.io.BufferedReader;
import java.util.Iterator;
import net.sf.gluebooster.java.booster.basic.transformation.CallableClassConverter;
import net.sf.gluebooster.java.booster.essentials.container.IteratorWithIterable;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.Callable;
import net.sf.gluebooster.java.booster.essentials.meta.objects.ObjectHolder;
import net.sf.gluebooster.java.booster.essentials.utils.Constants;
import net.sf.gluebooster.java.booster.essentials.utils.ThrowableBoostUtils;
/**
* Iterates over multiple classes. Simple converts should be done with the CallableClassConverter.
*
* @defaultParamText name the name of the iterator
* @defaultParamText type the type of the iterator
* @author cbauer
*
*/
public class IteratorOfContainer extends IteratorWithIterable {
/**
* The filter of objects (result false: ignore the object)
*/
private Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy