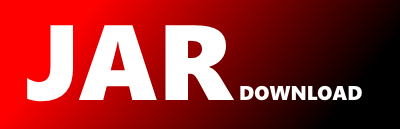
net.sf.gluebooster.java.booster.basic.container.ParameterizedStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.container;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import net.sf.gluebooster.java.booster.basic.db.ResultSetIterator;
import net.sf.gluebooster.java.booster.basic.db.Sql;
/**
* Configuration of a statement with parameters
*
* @defaultParamText statement the statement that is parameterized.
* @defaultParamText parameters the parameters of the statement
*
* @author cbauer
*
*/
public class ParameterizedStatement {
/**
* the type of the statement
*/
private Object type;
/**
* the statement that is parameterized.
*/
private String statement;
/**
* the parameters of the statement
*/
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy