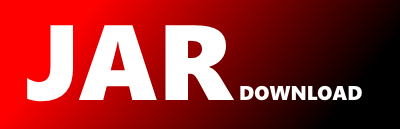
net.sf.gluebooster.java.booster.basic.container.ResourceBundleFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.container;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import java.util.ResourceBundle;
import net.sf.gluebooster.java.booster.basic.meta.DocumentationContext;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.Callable;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.CallableAbstraction;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.CallableByReflection;
import net.sf.gluebooster.java.booster.essentials.utils.Check;
import net.sf.gluebooster.java.booster.essentials.utils.Constants;
import net.sf.gluebooster.java.booster.essentials.utils.ThrowableBoostUtils;
/**
* Returns/Creates resource bundles
*
* @defaultParamText name the name of the instance
* @defaultParamText locale the given locale
* @defaultParamText key the given key
*
* @author cbauer
*
*/
public class ResourceBundleFactory extends CallableAbstraction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy