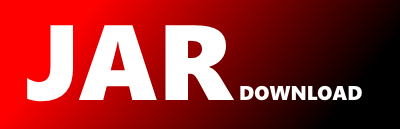
net.sf.gluebooster.java.booster.basic.gui.BoostedNodeVisualization Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.gui;
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Set;
import javax.swing.JFrame;
import org.apache.commons.lang3.tuple.ImmutablePair;
import org.apache.commons.lang3.tuple.Pair;
import edu.uci.ics.jung.algorithms.layout.FRLayout;
import edu.uci.ics.jung.algorithms.layout.Layout;
import edu.uci.ics.jung.graph.DirectedSparseMultigraph;
import edu.uci.ics.jung.visualization.VisualizationViewer;
import net.sf.gluebooster.java.booster.basic.container.BoostedNode;
import net.sf.gluebooster.java.booster.basic.container.SimpleBoostedNode;
import net.sf.gluebooster.java.booster.basic.gui.swing.SwingBoostUtils;
import net.sf.gluebooster.java.booster.basic.math.graph.JungVisualizationViewer;
import net.sf.gluebooster.java.booster.basic.transformation.CallableByMap;
import net.sf.gluebooster.java.booster.basic.transformation.FunctionByCallable;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.Callable;
/**
* Graphical display of one BoostedNode, its In- and Out-Points and the
* connection to the pre- and successors. It has methods to vizalize the
* changing of the connections.
*
* @author CBauer
*
*/
public class BoostedNodeVisualization {
/**
* The graph of the points
*/
private DirectedSparseMultigraph graph = new DirectedSparseMultigraph();
/**
* The labels of the points
*/
private HashMap labels = new HashMap();
/**
* The edges between the points
*/
private Set> edges = new HashSet>();
/**
* A counter to name the edges.
*/
private int counter = 0;
/**
* Constructor with values.
*
* @param nodesWithInterestInOutgoingEdges
* of which node is interest in the outgoing edges.
* @param nodesWithInterestInIngoingEdges
* of which node is interest in the ingoing edges.
*/
public BoostedNodeVisualization(
Collection nodesWithInterestInOutgoingEdges,
Collection nodesWithInterestInIngoingEdges) {
for (BoostedNode node : nodesWithInterestInOutgoingEdges) {
addNodeIfNecessary(node, ""
+ ((SimpleBoostedNode) node).getAttributes().getValue());
for (BoostedNode outpoint : node.getOutPoints()) {
addEdge(node, outpoint);
addEdges(outpoint, node.getSuccessorInPoints(outpoint));
}
}
for (BoostedNode node : nodesWithInterestInIngoingEdges) {
addNodeIfNecessary(node, ""
+ ((SimpleBoostedNode) node).getAttributes().getValue());
for (BoostedNode inpoint : node.getInPoints()) {
addEdge(inpoint, node);
addEdges(node.getPredecessorOutPoints(inpoint), inpoint);
}
}
Layout layout = new FRLayout(
graph);
layout.setSize(new Dimension(300, 300));
VisualizationViewer visualization = new JungVisualizationViewer(
layout);
visualization.setPreferredSize(new Dimension(700, 700));
visualization.getRenderContext().setVertexLabelTransformer(
new FunctionByCallable(
(Callable) CallableByMap.getFromMap("VertexLabel", labels, null)));
int size = 800;
SwingBoostUtils guiFactory = new SwingBoostUtils();
JFrame frame = guiFactory.createFrame("BoostedNodeVisualization", size,
size, true, new BorderLayout());
frame.getContentPane().add(visualization, BorderLayout.CENTER);
frame.setVisible(true);
}
/**
* If the node is not yet present, add it.
*
* @param node
* the new node
* @param label
* the label of the node
*/
private void addNodeIfNecessary(BoostedNode node, String label) {
if (!labels.containsKey(node)) {
labels.put(node, label);
graph.addVertex(node);
}
}
/**
* Adds an edge between two nodes
*
* @param from
* the starting of the edge
* @param to
* the end of the edge
*/
private void addEdge(BoostedNode from, BoostedNode to){
addNodeIfNecessary(from, "");
addNodeIfNecessary(to, "");
Pair edge = new ImmutablePair(
from, to);
if (!edges.contains(edge)) {
edges.add(edge);
graph.addEdge("" + counter++, from, to);
}
}
/**
* Adds edges from one node to multiple nodes
*
* @param from
* the start of the edges
* @param to
* the ends of the edges
*/
private void addEdges(BoostedNode from, Collection to) {
for (BoostedNode node : to) {
addEdge(from, node);
}
}
/**
* Adds edges from multiple nodes to one node
*
* @param from
* the start of the edges
* @param to
* the end of the edges
*/
private void addEdges(Collection from, BoostedNode to) {
for (BoostedNode node : from) {
addEdge(node, to);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy