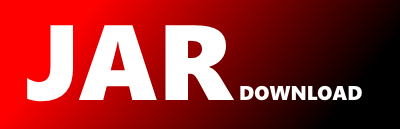
net.sf.gluebooster.java.booster.basic.gui.UserInteractionBoostUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.gui;
import net.sf.gluebooster.java.booster.basic.transformation.CallableByCalling;
import net.sf.gluebooster.java.booster.basic.transformation.CallableByChecking;
import net.sf.gluebooster.java.booster.basic.transformation.CallableChain;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.Callable;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.CallableAbstraction;
import net.sf.gluebooster.java.booster.essentials.math.ConditionBoostUtils;
import net.sf.gluebooster.java.booster.essentials.meta.objects.ObjectDescription;
/**
* Utility methods for user interactions.
*
* @author cbauer
*
*/
public class UserInteractionBoostUtils {
/**
* Create a callable that displayDialog a dialog and returns the result of the dialog.
*
* @param purpose
* purpose of the dialog
* @param userInteractionFactory
* will be called with the parameters of the call and has to return a userInteraction
* @param dialog
* the dialog to display
* @param callableResult
* the class of the result of the call
* @param appendCallParameters
* should the parameters of the call should be appended to the default call parameters.
* @return the result of the dialog. May be a file or a text or null if the dialog is cancelled
*/
public static Callable displayDialog(String purpose, Callable userInteractionFactory, DialogConfiguration dialog, boolean appendCallParameters,
Class callableResult)
throws Exception {
// Object pre = userInteractionFactory.getPrecondition();
// Object post = userInteractionFactory.getPostcondition(null);
// the result of the userInteractionFactory must be a userInteraction
ObjectDescription resultOfUserInteractionFactoryMustBeUserInteraction = new ObjectDescription(UserInteraction.class);
ConditionBoostUtils.assertNoConflict(resultOfUserInteractionFactoryMustBeUserInteraction, userInteractionFactory.getPostcondition(null),
UserInteractionBoostUtils.class.getSimpleName() + ".displayDialog");
CallableByCalling result = new CallableByCalling<>("display dialog and append parameters: " + purpose,
new Callable[] { new CallableChain("display dialog: " + purpose,
new CallableByCalling<>("user interaction, dialog configuration", new Object[] { userInteractionFactory, dialog }),
CallableByChecking.classInstanceChecker("check that there is really a user interaction", 2, UserInteraction.class,
"The result of the userInteractionFactory must be a userInteraction. Factory is " + userInteractionFactory + " ("
+ userInteractionFactory.getClass().getSimpleName()
+ ") Correct the invocation of UserInteractionBoostUtils.displayDialog",
DialogConfiguration.class, "the second parameter should be a DialogConfiguration"),
new CallableByCalling("do dialog")
// result of dialog
) });
result.setAppendCallParameters(appendCallParameters);
result.setResultClass(callableResult);
// Object pre2 = result.getPrecondition();
// Object post2 = result.getPostcondition(null);
return result;
}
/**
* Displays a dialog and handles the result
*
* @param purpose
* purpose of the dialog
* @param userInteractionFactory
* will be called with the parameters of the call and has to return a userInteraction
* @param dialog
* the dialog to display
* @param dialogResultHandler
* will be called with the result of the dialog and the original parameters of the call.
* @return the result of the dialog. May be a file or a text
* @return
* @throws Exception
*/
public static CallableAbstraction displayDialogHandleResult(String purpose, Callable userInteractionFactory, DialogConfiguration dialog,
Callable dialogResultHandler)
throws Exception {
return new CallableChain("displayDialogHandleResult: " + purpose, displayDialog(purpose, userInteractionFactory, dialog, true, null),
dialogResultHandler);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy