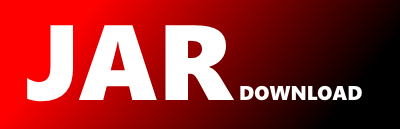
net.sf.gluebooster.java.booster.basic.gui.awt.BoostedRectangle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.gui.awt;
import java.awt.Dimension;
import java.awt.Point;
import java.awt.Rectangle;
import net.sf.gluebooster.java.booster.essentials.objects.BoostedObject;
/**
* Extension of the rectangle.
*
* @author CBauer
*
*/
public class BoostedRectangle extends Rectangle {
private static final long serialVersionUID = 1L;
/**
* The attributes of this rectangle
*/
private BoostedObject attributes = new BoostedObject();
public BoostedRectangle() {
super();
}
/**
* Constructor with name.
*
* @param name
* the name of this rectangle
*/
public BoostedRectangle(Object name) {
this();
attributes.setName(name);
}
/**
* Constructor with dimension
*
* @param d
* the dimension of this rectangle
*/
public BoostedRectangle(Dimension d) {
super(d);
}
/**
* Constructor with values.
*
* @param x
* x-coordinate
* @param y
* y-coordinate
* @param width
* the width of the rectangle
* @param height
* the height of the rectangle
*/
public BoostedRectangle(int x, int y, int width, int height) {
super(x, y, width, height);
}
/**
* Constructor with values.
*
* @param x
* x-coordinate
* @param y
* y-coordinate
* @param width
* the width of the rectangle
* @param height
* the height of the rectangle
* @param name
* the name of this rectangle
*/
public BoostedRectangle(Object name, int x, int y, int width, int height) {
this(x, y, width, height);
attributes.setName(name);
}
/**
* Constructor with values.
*
* @param width
* the width of the rectangle
* @param height
* the height of the rectangle
*/
public BoostedRectangle(int width, int height) {
super(width, height);
}
/**
* Constructor with values.
*
* @param p
* the origin
* @param d
* the dimension
*/
public BoostedRectangle(Point p, Dimension d) {
super(p, d);
}
/**
* Constructor with values.
*
* @param p
* the origin
*/
public BoostedRectangle(Point p) {
super(p);
}
/**
* Constructor with values.
*
* @param r
* the template
*/
public BoostedRectangle(Rectangle r) {
super(r);
}
public BoostedObject getAttributes() {
return attributes;
}
@Override
public String toString() {
return "{" + attributes.toString() + " " + "[x=" + x + ",y=" + y
+ ",width=" + width + ",height=" + height + "]" + "}";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy