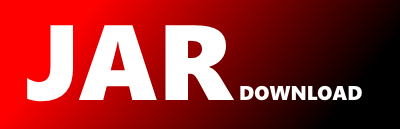
net.sf.gluebooster.java.booster.basic.math.Operation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.math;
import java.awt.Color;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.apache.commons.lang.ObjectUtils;
import net.sf.gluebooster.java.booster.basic.container.BoostedNode;
import net.sf.gluebooster.java.booster.basic.container.BoostedNodeUtils;
import net.sf.gluebooster.java.booster.basic.container.SimpleBoostedNode;
import net.sf.gluebooster.java.booster.basic.math.graph.BoostedNodeGraph;
import net.sf.gluebooster.java.booster.basic.math.planning.World;
import net.sf.gluebooster.java.booster.essentials.math.Condition;
import net.sf.gluebooster.java.booster.essentials.meta.Codable;
import net.sf.gluebooster.java.booster.essentials.meta.HasName;
import net.sf.gluebooster.java.booster.essentials.meta.Stringable;
import net.sf.gluebooster.java.booster.essentials.objects.BoostedObject;
import net.sf.gluebooster.java.booster.essentials.sourcecode.BasicJavaCodeGenerator;
import net.sf.gluebooster.java.booster.essentials.utils.MathBoostUtils;
import net.sf.gluebooster.java.booster.essentials.utils.ObjectBoostUtils;
import net.sf.gluebooster.java.booster.essentials.utils.TextBoostUtils;
/**
* An operation.
*
* @author CBauer
*
*
* @defaultParamText postcondition the postcondition of the operation
* @defaultParamText node the node of the operation
* @defaultParamText successor the successor node of the operation in a graph
* @defaultParamText predecessor the predecessor node of the operation in a graph
*
* @defaultParamText operator the operator of the operation
* @defaultParamText operatorDetails the operator details of the operation
* @defaultParamText solvingPriority the priority of the operation
*
*/
public class Operation
extends BoostedObject implements Stringable, Codable {
/**
* The precondition of the operation. The procondition states what is
* necessary that the operation will succeed.
*/
private Conditio precondition;
/**
* The postcondition of the operation. The postcondition states some
* (important) aspects of the result of the operation
*/
private Conditio postcondition;
/**
* The global precondition of the operation. The global precondition
* contains all informations about the world before the operation.
*
*/
private Conditio globalPrecondition;
/**
* The global postcondition of the operation. The global postcondition
* contains all informations about the world after the operation.
*
*/
private Conditio globalPostcondition;
/**
* The delete condition. The delete condition describes the parts of the
* world that are no longer valid after the operation has taken place.
*/
private Conditio deleteCondition;
/**
* The operator that is to be executed.
*/
private Operator operator;
/**
* Details (more specific) of the operator
*/
private Object operatorDetails;
/**
* The priority of this operation.
*
*/
private int solvingPriority;
public Operation() {
}
public Operation(Object operator) {
if (operator instanceof Operator) {
this.operator = (Operator) operator;
} else {
this.operator = new Operator(operator, 0);
}
}
public Operation(Operator operator,
Object operatorDetails,
int solvingPriority) {
this(operator, operatorDetails, solvingPriority, null, null);
}
public Operation(Operator operator,
Object operatorDetails,
int solvingPriority, Conditio precondition, Conditio postcondition) {
setOperator(operator);
setOperatorDetails(operatorDetails);
setSolvingPriority(solvingPriority);
setPrecondition(precondition);
setPostcondition(postcondition);
}
public Operation(Operator operator,
Object operatorDetails,
int solvingPriority, Conditio postcondition) {
this(operator, operatorDetails, solvingPriority, null, postcondition);
}
/**
* Create an operation with a postcondition
*
* @param operator
* @param operatorDetails
* @param postcondition
* @param solvingPriority
* @return the created condition
*/
public static Operation createWithPostcondition(
Operator operator, Object operatorDetails,
Conditio postcondition,
int solvingPriority) {
Operation result = new Operation(
operator,
operatorDetails,
solvingPriority);
result.setPostcondition(postcondition);
return result;
}
/**
* Craetes an operation that is to be solved.
*
* @param world
* the world of the objects
* @return the created condition
*/
public static Operation createProblemToSolveNode(
World world, Conditio globalPrecondition, Conditio postcondition) {
return world.createOperation(globalPrecondition,
world.getToSolveOperator(), postcondition, Integer.MAX_VALUE);
}
@Override
public String toString() {
StringBuilder result = new StringBuilder(operator.getName().toString());
if (operatorDetails != null) {
result.append(" ").append(operatorDetails);
}
return result.toString();
}
public Conditio getPrecondition() {
return precondition;
}
public void setPrecondition(Conditio precondition) {
this.precondition = precondition;
}
public Conditio getPostcondition() {
return postcondition;
}
public void setPostcondition(Conditio postcondition) {
this.postcondition = postcondition;
}
public Conditio getGlobalPrecondition() {
return globalPrecondition;
}
public void setGlobalPrecondition(Conditio globalPrecondition) {
this.globalPrecondition = globalPrecondition;
}
public Conditio getGlobalPostcondition() {
return globalPostcondition;
}
public void setGlobalPostcondition(Conditio globalPostcondition) {
this.globalPostcondition = globalPostcondition;
}
public Conditio getDeleteCondition() {
return deleteCondition;
}
public void setDeleteCondition(Conditio deleteCondition) {
this.deleteCondition = deleteCondition;
}
public Operator getOperator() {
return operator;
}
/**
* Gets the name from the operator.
*
* @return the name of the operator
*/
public Name getOperatorName() {
return (Name) operator.getName();
}
/**
* Has this operation a given name
*
* @param operatorname
* the wanted name
* @return this.name == operatorname
*/
public boolean hasOperatorname(Object operatorname) {
return operator.hasName(operatorname);
}
public void setOperator(Operator operator) {
this.operator = operator;
setName(operator.getName());
}
public int getSolvingPriority() {
return solvingPriority;
}
public void setSolvingPriority(int solvingPriority) {
this.solvingPriority = solvingPriority;
}
/**
* There is no need to solve an operation, if its postcondition is already fullfilled by the global precondition.
*
* @return true if this operation does not need to be solved
*/
public boolean isNoNeedToSolve() throws Exception {
if (globalPrecondition == null || postcondition == null) {
return false;
} else {
return globalPrecondition.implies(postcondition).isEmpty();
}
}
/**
* An operation is solved, if it has a global postcondition that implies the postcondition or it has a global precondition that implies the postcondition
* (in that case no operation has to take place)
*
* @return true if this operation is solved
*/
public boolean isSolved() throws Exception {
if (globalPostcondition == null) {
return false;
} else {
Collection problems = globalPostcondition.implies(postcondition);
return problems != null && problems.isEmpty();
}
}
/**
* Checks whether the level of this operator is a given level
*
* @param level
* the level to be compared with
* @return true if the levels are equal
*/
public boolean hasOperatorAbstractionLevel(int level) {
return operator.getAbstractionLevel() == level;
}
@Override
public String toString(int level) throws Exception {
StringBuilder result = new StringBuilder(toString());
if (level > 0){
if (isSolved()) {
result.append(" (solved) ");
} else if (isNoNeedToSolve()) {
result.append(" (no need to solve) ");
}
result.append( getStringIfNotNull("Precondition", precondition));
result.append( getStringIfNotNull("Postcondition", postcondition));
}
if (level > 1){
result.append( getStringIfNotNull("Global Postcondition", globalPostcondition));
}
if (level > 2){
result.append( getStringIfNotNull("Global Precondition: ",globalPrecondition));
}
if (level > 3) {
Object condition = globalPostcondition;
if (condition != null && condition instanceof Stringable) {
result.append("Global Precondition: \n" + ((Stringable) condition).toString(2));
}
}
// asSourcecode(result);
return result.toString();
}
/**
* Compute a string representation of a label and value.
*
* @param label
* the label
* @param value
* the value
* @return label: value
*/
private String getStringIfNotNull(String label, Conditio value) {
if (value == null)
return "";
else
return " \n" + label + ": " + value;
}
public Result getOperatorDetails() {
return (Result) operatorDetails;
}
public void setOperatorDetails(Object operatorDetails) {
this.operatorDetails = operatorDetails;
}
/**
* Gets the operation of a node
*
* @return the operation
*/
@SuppressWarnings("unchecked")
public static /*, NameOfObjects>*/ Operation/**/ getOperation(
BoostedNode node) {
return (Operation/**/) node.getAttributes()
.getValue();
}
/**
* Sets the operation of a node
*
* @param value
* the new operation
*/
public static void setOperation(BoostedNode node, Operation value) {
node.getAttributes().setValue(value);
}
/**
* Creates a new node
*
* @param predecessor
* will be predecessor of the new node
* @param content
* the content of the new node
* @return the created node
*/
public static BoostedNode createSuccessor(BoostedNode predecessor, Operation content)
throws Exception {
BoostedNode result = BoostedNodeUtils.createSuccessor(predecessor);
setOperation(result, content);
return result;
}
/**
* Create a new node.
*
* @param successor
* the successor of the new node
* @param content
* the content of the new node
* @return the created node
*/
public static BoostedNode createPredecessor(BoostedNode successor, Operation content)
throws Exception {
BoostedNode result = BoostedNodeUtils.createPredecessor(successor);
setOperation(result, content);
return result;
}
/**
* Create a new node.
*
* @param content
* the operation of the node
* @return the created node
*/
public static BoostedNode createBoostedNode(Operation content)
throws Exception {
BoostedNode result = new SimpleBoostedNode();
setOperation(result, content);
return result;
}
/**
* Creates a edges between nodes.
*
* @param nodes
* the content of the nodes
* @return the last node of the sequence
*/
public static BoostedNode createBoostedNodeSequence(
Operation>... nodes)
throws Exception {
BoostedNode last = null;
for (Operation> node : nodes) {
if (last == null) {
last = createBoostedNode(node);
} else {
last = createSuccessor(last, node);
}
}
return last;
}
/**
* Adds edges to form a sequence
*
* @param nodes
* will be connected
* @return the last node of the sequence
*/
public static BoostedNode createBoostedNodeSequence(
List> nodes) throws Exception {
BoostedNode last = null;
for (Operation> node : nodes) {
if (last == null) {
last = createBoostedNode(node);
} else {
last = createSuccessor(last, node);
}
}
return last;
}
/**
* Creates a seqence graph of nodes
*
* @param nodeSequence
* the operations of the nodes
* @return the sequence as graph
*/
public static BoostedNodeGraph createBoostedNodeGraph(
Operation>... nodeSequence) throws Exception {
BoostedNodeGraph result = new BoostedNodeGraph();
result.addNode(createBoostedNodeSequence(nodeSequence));
return result;
}
/**
* Creates a seqence graph of nodes
*
* @param nodeSequence
* the operations of the nodes
* @return the sequence as graph
*/
public static BoostedNodeGraph createBoostedNodeGraph(
List> nodeSequence) throws Exception {
BoostedNodeGraph result = new BoostedNodeGraph();
result.addNode(createBoostedNodeSequence(nodeSequence));
return result;
}
/**
* Is the operator elementary
*
* @return true if elementary
*/
public boolean isOperatorElementary() {
return operator.isElementary();
}
/**
* replace the name of a given object by another name
*
* @param object
* the object which name is to be replaced
* @param newName
* the new name
*/
@Override
public void replaceObjectName(Object oldName, Object newName) throws Exception {
if (precondition != null)
precondition.replaceObjectName(oldName, newName);
if (postcondition != null)
postcondition.replaceObjectName(oldName, newName);
if (globalPrecondition != null)
globalPrecondition.replaceObjectName(oldName, newName);
if (globalPostcondition != null)
globalPostcondition.replaceObjectName(oldName, newName);
if (operatorDetails instanceof Condition) {
((Condition) operatorDetails).replaceObjectName(oldName, newName);
}
}
/**
* Adds a condition to the precondition
*
* @param condition
* the added condition
*/
public void addToPrecondition(Conditio condition) throws Exception {
if (condition != null) {
if (precondition == null) {
precondition = condition;
} else {
precondition.add(condition);
}
}
}
/**
* Adds a condition to the global precondition
*
* @param condition
* the added condition
*/
public void addToGlobalPrecondition(Conditio condition) throws Exception {
if (condition != null) {
if (globalPrecondition == null) {
globalPrecondition = condition;
} else {
globalPrecondition.add(condition);
}
}
}
/**
* Adds a condition to the postcondition
*
* @param condition
* the added condition
*/
public void addToPostcondition(Conditio condition) throws Exception {
if (condition != null) {
if (postcondition == null) {
postcondition = condition;
} else {
postcondition.add(condition);
}
}
}
/**
* Adds a condition to the delete condition
*
* @param condition
* the added condition
*/
public void addToDeletecondition(Conditio condition) throws Exception {
if (condition != null) {
if (deleteCondition == null) {
deleteCondition = condition;
} else {
deleteCondition.add(condition);
}
}
}
/**
* Tries to find an element in the preconditions. Preconditions are global precondition and precondition
*
* @param template
* the template that is searched for
* @return the instance of the template if the template can be found
*/
public Result findInPreconditions(Result template)
throws Exception {
Result result = null;
if (globalPrecondition != null) {
result = globalPrecondition.findSubcondition(template);
}
if (result == null && precondition != null) {
result = precondition.findSubcondition(template);
}
return result;
}
/**
* Creates a list of operations.
*
* @param operatorDetails
* details of each created operation
* @param solvingPriority
* solving priority of each created operation
* @param operators
* the operators of the created operations
* @return the created operations
*/
public static List> createOperations(Object operatorDetails, int solvingPriority,
Operator... operators) throws Exception {
ArrayList> result = new ArrayList>();
for (Operator operator : operators) {
result.add(new Operation(operator,
ObjectBoostUtils.clone(operatorDetails), solvingPriority));
}
return result;
}
/**
* Creates a list of operations.
*
* @param solvingPriority
* solving priority of each created operation
* @param operatorsAndDetails
* each array contains the operator and the details
* @return the created operations
*/
public static List> createOperations(
int solvingPriority, Object[/*
* Operator,
* WorldObject
* operatorDetails
*/]... operatorsAndDetails)
throws Exception {
ArrayList> result = new ArrayList>();
for (Object[] operatorAndDetails : operatorsAndDetails) {
Operator operator = (Operator) operatorAndDetails[0];
HasName operatorDetail = (HasName) operatorAndDetails[1];
result.add(new Operation(operator,
operatorDetail, solvingPriority));
}
return result;
}
@Override
public String asSourcecode(StringBuilder result, Object... parameters)
throws Exception {
String uid = MathBoostUtils.getTemporaryId();
String objectname = "operation" + uid;
TextBoostUtils.append(result, "\nOperation ", objectname,
"= new Operation();\n");
TextBoostUtils.append(result, objectname, ".setSolvingPriority(",
solvingPriority, ");\n");
BasicJavaCodeGenerator.generateSetterInvocation(objectname, "setDeleteCondition",
(Codable) deleteCondition, result);
BasicJavaCodeGenerator.generateSetterInvocation(objectname, "setGlobalPostcondition",
(Codable) globalPostcondition, result);
BasicJavaCodeGenerator.generateSetterInvocation(objectname, "setGlobalPrecondition",
(Codable) globalPrecondition, result);
BasicJavaCodeGenerator.generateSetterInvocation(objectname, "setPostcondition",
(Codable) postcondition, result);
BasicJavaCodeGenerator.generateSetterInvocation(objectname, "setPrecondition",
(Codable) precondition, result);
BasicJavaCodeGenerator.generateSetterInvocation(objectname, "setOperator",
(Codable) operator, result);
BasicJavaCodeGenerator.generateSetterInvocation(objectname, "setOperatorDetails",
(Codable) operatorDetails, result);
return objectname;
}
/**
* Checks that the operator and operatordetails are valid. Must be
* overwritten by subclasses.
*
* @throws IllegalStateException
* if the operator is not valid
*/
public void checkOperator() throws Exception {
getLog().info(
getClass().getSimpleName()
+ ".checkOperator must be overwritten by subclasses to have an effect.");
}
/**
* Returns a color according to the state of this operation (problems: more red; solved green): solved: green; no need to solve: cyan, if the global
* precondition does not imply the precondition: red; a global postcondition exists: yellow; no global postcondition: orange
*
* @return the color of the state
*/
public Color getDefaultSolveStateColor() throws Exception {
Color result = null;
if (isSolved()) {
result = Color.GREEN;
} else if (isNoNeedToSolve()) {
result = Color.CYAN;
} else {
Conditio globalPrecondition = getGlobalPrecondition();
Conditio precondition = getPrecondition();
if (globalPrecondition != null && precondition != null) {
if (!globalPrecondition.implies(precondition).isEmpty()) {
result = Color.RED;
}
}
if (result == null) {
if (getGlobalPostcondition() != null) {
result = Color.YELLOW;
} else {
result = Color.ORANGE;
}
}
}
return result;
}
/**
* Is there a conflict between the pre- (or post)conditions of the two operations
*
* @param operation2
* the other operation
* @return true if a conflict exists
*/
public boolean hasPreOrPostconditionConflict(Operation operation2) throws Exception {
Collection resultCollection;
boolean result = false;
if (precondition != null && operation2.precondition != null) {
resultCollection = precondition.isInConflict(operation2.precondition);
if (resultCollection != null && !resultCollection.isEmpty()) {
return true;
}
}
if (postcondition != null && operation2.postcondition != null) {
resultCollection = postcondition.isInConflict(operation2.postcondition);
if (resultCollection != null && !resultCollection.isEmpty()) {
return true;
}
}
return false;
}
/**
* Are the operator and operator details equal
*
* @param otherOperation
* the operation to be compared with
* @return true iff equal
*/
public boolean equalOperatorAndDetails(Operation otherOperation) {
return ObjectUtils.equals(operator, otherOperation.getOperator()) && ObjectUtils.equals(operatorDetails, otherOperation.getOperatorDetails());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy