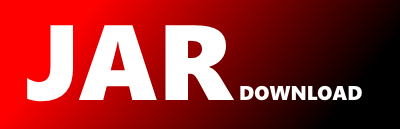
net.sf.gluebooster.java.booster.basic.meta.ObjectAnalyzer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.meta;
import java.lang.reflect.Field;
import java.util.ArrayList;
import net.sf.gluebooster.java.booster.essentials.utils.ReflectionBoostUtils;
/**
* Runtime analysis of objects.
*
* @author christian.bauer
*
* @param
* the class of the object
* @defaultParamText fieldName the name of the field
* @defaultParamText value the new value
*/
public class ObjectAnalyzer {
/**
* The object to analyze
*/
private Objectclass object;
/**
* Constructor without object.
* The object must be set separately.
*/
public ObjectAnalyzer()
{
}
/**
* Constructor with object.
* @param object the object to analyze
*/
public ObjectAnalyzer(Objectclass object)
{
setObject(object);
}
/**
* Getter of the object
* @return object
*/
public Objectclass getObject() {
return object;
}
/**
* Setter of the object
* @param object the new value
*/
public void setObject(Objectclass object) {
this.object = object;
}
/**
* Fetches the value of a private field
*
* @return the value of the field
*/
@SuppressWarnings("unchecked")
public static Fieldclass getPrivateField(Object object,
String fieldName) throws IllegalAccessException,
NoSuchFieldException {
return (Fieldclass) new ObjectAnalyzer(object)
.getPrivateField(fieldName);
}
/**
* Create an analyzer for the value of a private field
*
* @return the created analyzer
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public static ObjectAnalyzer getPrivateFieldAnalyzer(Object object,
String fieldName) throws IllegalAccessException,
NoSuchFieldException {
return new ObjectAnalyzer(getPrivateField(object, fieldName));
}
/**
* Fetches the value of a private field
* @param the class of the value.
* @return object.fieldName
* @throws IllegalAccessException if the access is not possible
* @throws NoSuchFieldException if the field is not found
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
public Fieldclass getPrivateField(String fieldName) throws IllegalAccessException, NoSuchFieldException
{
// Check we have valid arguments...
if (object == null) throw new IllegalArgumentException("object is null");
if (fieldName == null) throw new IllegalArgumentException("fieldname is null");
Class objectclass = object.getClass();
try
{
Field field = ReflectionBoostUtils.getField(objectclass, fieldName);
//TODO get inherited fields, too
field.setAccessible(true);
return (Fieldclass) field.get(object);
}
catch (NoSuchFieldException ex)
{
ArrayList fieldnames = new ArrayList();
for (Field field: objectclass.getDeclaredFields()) //TODO get inherited fields, too
fieldnames.add(field.getName());
NoSuchFieldException exception = new NoSuchFieldException("No field '" + fieldName + "' in " + fieldnames);
exception.initCause(ex);
throw exception;
}
}
/**
* Set the value of a private field
*/
public static void setPrivateField(Object object, String fieldName,
Object value) throws Exception {
new ObjectAnalyzer(object).setPrivateField(fieldName, value);
}
/**
* Set the value of a private field
*/
@SuppressWarnings("rawtypes")
public void setPrivateField(String fieldName, Object value)
throws IllegalAccessException, NoSuchFieldException
{
// Check we have valid arguments...
if (object == null) throw new IllegalArgumentException("object is null");
if (fieldName == null) throw new IllegalArgumentException("fieldname is null");
Class objectclass = object.getClass();
try
{
Field field = ReflectionBoostUtils.getField(objectclass, fieldName);
//TODO get inherited fields, too
field.setAccessible(true);
field.set(object, value);
}
catch (NoSuchFieldException ex)
{
ArrayList fieldnames = new ArrayList();
for (Field field: objectclass.getDeclaredFields()) //TODO get inherited fields, too
fieldnames.add(field.getName());
NoSuchFieldException exception = new NoSuchFieldException("No field '" + fieldName + "' in " + fieldnames);
exception.initCause(ex);
throw exception;
}
}
/**
* Invokes a private method of the object.
*
* @param
* the class of the return value
* @param methodName
* the name of the method
* @param params
* the parameters of the method
* @return object.methodName(params)
* @throws Exception
* if a problem occurs.
* @deprecated use the ReflectionBoostUtils directly
*/
@SuppressWarnings("unchecked")
@Deprecated
public Resultclass invokePrivateMethod(String methodName,
Object[] params) throws Exception
{
return ReflectionBoostUtils.invokePrivateMethod(object, methodName,
params);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy