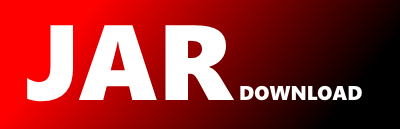
net.sf.gluebooster.java.booster.basic.resources.ClassResourceIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.resources;
import java.io.InputStream;
import java.util.Iterator;
import java.util.NoSuchElementException;
import net.sf.gluebooster.java.booster.basic.container.BoostedNode;
import net.sf.gluebooster.java.booster.basic.meta.DocumentationContext;
import net.sf.gluebooster.java.booster.basic.meta.HasDocumentation;
import net.sf.gluebooster.java.booster.basic.text.general.BookBoostUtils;
import net.sf.gluebooster.java.booster.basic.text.general.DocumentationResourcesId;
import net.sf.gluebooster.java.booster.essentials.objects.BoostedObject;
import net.sf.gluebooster.java.booster.essentials.utils.IoBoostUtils;
/**
*Creates input streams of a given class resource.
*
* @author Christian Bauer
*
*/
public class ClassResourceIterator extends BoostedObject implements Iterator, HasDocumentation
{
/**
* The class that is used to get the resource input stream.
*/
@SuppressWarnings("rawtypes")
private Class resourceGettingClass;
/**
* The name of the resource
*/
private String resourceName;
/**
* Should an exception be thrown when a resource is not found.
*/
private boolean throwExceptionIfResourceIsNotFound = false;
/**
* Should the hasNext method check whether the resource is available or not.
*/
private boolean checkHasNext = false;
public ClassResourceIterator() {
}
/**
* Constructor with all necessary parameters.
* @param resourceGettingClass The class that is used to get the resource input stream.
* @param resourceName The name of the resource
*/
@SuppressWarnings("rawtypes")
public ClassResourceIterator(Class resourceGettingClass, String resourceName)
{
this.resourceGettingClass = resourceGettingClass;
this.resourceName = resourceName;
}
/**
* Constructor with more parameters
* @param resourceGettingClass The class that is used to get the resource input stream.
* @param resourceName The name of the resource
* @param throwExceptionIfNotFound Should an exception be thrown when a resource is not found.
*/
@SuppressWarnings("rawtypes")
public ClassResourceIterator(Class resourceGettingClass, String resourceName, boolean throwExceptionIfNotFound)
{
this(resourceGettingClass, resourceName);
throwExceptionIfResourceIsNotFound = throwExceptionIfNotFound;
}
/**
* @return If checkHasNext is true, it checks whether the resource exists. Otherwise always returns true, even when the resource does not exist.
*/
@Override
public boolean hasNext()
{
if (checkHasNext)
return IoBoostUtils.getResource(resourceGettingClass, resourceName,
false, false) != null;
else
return true;
}
/**
* Returns always a new input stream or throws an exception if the resource does not exist.
* @throws NoSuchElementException if the resource is not found and throwExceptionIfResourceIsNotFound is true
*/
@Override
public InputStream next()
{
return IoBoostUtils.getResource(resourceGettingClass, resourceName,
true, throwExceptionIfResourceIsNotFound);
}
/**
* Always throws an UnsupportedOperationException.
*/
@Override
public void remove() throws UnsupportedOperationException
{
throw new UnsupportedOperationException();
}
/**
* Getter of the corresponding property.
* @return the value of the property
*/
public boolean isThrowExceptionIfResourceIsNotFound() {
return throwExceptionIfResourceIsNotFound;
}
/**
* Setter of the corresponding property.
* @param throwExceptionIfResourceIsNotFound the new value
*/
public void setThrowExceptionIfResourceIsNotFound(boolean throwExceptionIfResourceIsNotFound) {
this.throwExceptionIfResourceIsNotFound = throwExceptionIfResourceIsNotFound;
}
@Override
public void fillDocumentation(Object type, BoostedNode documentationRoot,
DocumentationContext context) {
if (DocumentationResourcesId.DOCUMENTATION_TECHNICAL
.equals(type)) {
BoostedNode div = BookBoostUtils.createDiv(documentationRoot);
BookBoostUtils.createText(div, "The class that fetches the resource is " + resourceGettingClass.getSimpleName() + ". The resource is '" + resourceName + "'");
}
}
public boolean isCheckHasNext() {
return checkHasNext;
}
public void setCheckHasNext(boolean checkHasNext) {
this.checkHasNext = checkHasNext;
}
/**
* Get the path to the resources.
*
* @return package-path/resourceName
*/
public String getResourcePath(){
return resourceGettingClass.getPackage().getName().replace('.', '/') + resourceName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy