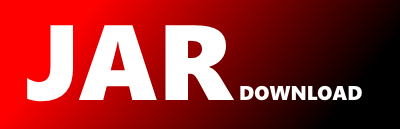
net.sf.gluebooster.java.booster.basic.transformation.CallableByChecking Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.transformation;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.Callable;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.CallableAbstraction;
import net.sf.gluebooster.java.booster.essentials.math.Condition;
import net.sf.gluebooster.java.booster.essentials.math.ConditionBoostUtils;
import net.sf.gluebooster.java.booster.essentials.meta.objects.ObjectDescription;
import net.sf.gluebooster.java.booster.essentials.utils.Constants;
/**
* Does some checks of the parameters and returns the parameters. Throws an exception if a check fails.
*
* @defaultParamText name the name of the callable
*
* @author cbauer
*
*/
public class CallableByChecking extends CallableAbstraction {
/**
* Checks the given indices
*/
private Callable[] checkPerIndex;
/**
* Checks the given indices
*/
private ObjectDescription[] valueOfIndex;
/**
* The condition the parameter array must fulfill.
*/
private ObjectDescription checkValue;
/**
* Is to be checked that that call-parameter must not be null
*/
private boolean checkNotNull = false;
/**
* The number of mandatory call-parameters. If there are more parameters, they are optional.
*/
private Integer minLength;
/**
* Is only the first parameter be returned (true) or the parameter array (false)
*/
private boolean returnOnlyFirstParameter = false;
public CallableByChecking(Object name) {
super(name);
}
public CallableByChecking(Object name, Callable... checkPerIndex) {
this(name);
this.checkPerIndex = checkPerIndex;
}
public CallableByChecking(Object name, ObjectDescription[] valueOfIndex) {
this(name, valueOfIndex, false);
}
/**
*
* @param returnOnlyFirstParameterNoArray
* should only the first parameter be returned from the call
*/
public CallableByChecking(Object name, ObjectDescription[] valueOfIndex, boolean returnOnlyFirstParameterNoArray) {
this(name);
this.valueOfIndex = valueOfIndex;
returnOnlyFirstParameter = returnOnlyFirstParameterNoArray;
}
/**
*
* @param valueCheck
* configuration of the expected value
*/
public CallableByChecking(Object name, ObjectDescription valueCheck) {
this(name);
checkValue = valueCheck;
}
/**
* Create a callable that checks that the call-parameter is not null
*
* @return the created callable
*/
public static CallableByChecking notNullChecker(Object name) {
CallableByChecking result = new CallableByChecking(name);
result.setCheckNotNull(true);
return result;
}
/**
* Create a callable that checks that the call-parameter is not null
*
* @param classOfObject
* the class of the result
* @return the created callable
*/
public static CallableAbstraction notNullChecker(Object name, Class classOfObject) {
return new CallableChain("not null and a " + classOfObject.getSimpleName(), CallableByChecking.notNullChecker("not null"),
classInstanceChecker("check class " + classOfObject.getSimpleName(), 1, classOfObject)
// new CallableByChecking("check class " + classOfObject.getSimpleName(), ObjectDescription.createArrayCondition(new ObjectDescription(classOfObject),
// 1, null))
);
}
/**
* Create a callable that checks that the parameter is an instance of a given class
*
* @param classAndOptionalDescription
* the class and optional a description
* @return the created callable
*/
public static CallableByChecking classInstanceChecker(Object name, Integer minLength, Object... classAndOptionalDescription) {
return classInstanceChecker(false, name, minLength, classAndOptionalDescription);
}
/**
* Creates a callable that checks that the call-parameter has a given value
*
* @param value
* the expected value
* @return the created callable
*/
public static CallableAbstraction singleValueChecker(Object name, Object value) {
return new CallableByChecking(name, ObjectDescription.createArrayCondition(null, ObjectDescription.createWithValue(value), 1, 1));
}
/**
* Create a callable that checks that the parameter is an instance of a given class
*
* @param breakpoint
* not used yet
* @param classAndOptionalDescription
* the class and optional a description
* @param minLength
* the number of mandatory parameters of the call. The following are optional
* @param classAndOptionalDescriptionMandatory
* the class which instance is expected, optional a description of the test, the next class, the next optional description, the next class, ...
* @return the created callable
*/
public static CallableByChecking classInstanceChecker(boolean breakpoint, Object name, Integer minLength, Object... classAndOptionalDescription) {
CallableByChecking result = new CallableByChecking(name);
ArrayList checks = new ArrayList();
for (Object classOrDescription : classAndOptionalDescription) {
if (classOrDescription instanceof Class) {
checks.add(new ObjectDescription<>((Class) classOrDescription));
} else {// description
ObjectDescription lastCheck = checks.get(checks.size() - 1);
if (lastCheck.getName() != null) {
throw new IllegalStateException("name already set. Maybe the classAndOptionalDescription has two following descriptions");
} else {
lastCheck.setName(classOrDescription);
}
}
}
return result;
}
@Override
protected Object callImpl(Object... parameters) throws Exception {
int parametersLength = parameters.length;
if (minLength != null && minLength > parametersLength) {
throw new IllegalStateException("minLength " + minLength + " not reached. Got " + parametersLength);
}
if (checkPerIndex != null) {
for (int index = 0; index < checkPerIndex.length; index++) {
if (parametersLength > index) {
Callable checker = checkPerIndex[index];
if (checker != null) {
checker.call(parameters[index]);
}
} else {
// optional parameter, skip the test
}
}
}
if (valueOfIndex != null) {
for (int index = 0; index < valueOfIndex.length; index++) {
ObjectDescription checker = valueOfIndex[index];
ConditionBoostUtils.assertNoConflict(checker, ObjectDescription.createWithValue(parameters[index]), getName());
}
}
if (checkValue != null) {
ConditionBoostUtils.assertNoConflict(checkValue, ObjectDescription.createWithValue(parameters), getName());
}
if (checkNotNull) {
for (int index = 0; index < parameters.length; index++) {
if (parameters[index] == null) {
throw new IllegalStateException("Parameter at index " + index + " must not be null: " + Arrays.asList(parameters));
}
}
}
if (returnOnlyFirstParameter) {
return parameters[0];
} else {
return parameters;
}
}
public boolean isCheckNotNull() {
return checkNotNull;
}
public void setCheckNotNull(boolean checkNotNull) {
this.checkNotNull = checkNotNull;
}
public ObjectDescription getCheckValue() {
return checkValue;
}
public void setCheckValue(ObjectDescription checkValue) {
this.checkValue = checkValue;
}
@Override
public Condition getPrecondition() throws Exception {
if (checkPerIndex != null || valueOfIndex != null) {
ObjectDescription result = new ObjectDescription();
result.setSubdescriptionType(Constants.ARRAY);
int minLength = 0;
if (checkPerIndex != null) {
// List subdescriptions = new ArrayList(checkPerIndex.length);
minLength = checkPerIndex.length;
// TODO add subdescriptions from callables
// for (Callable callable: checkPerIndex){
// callable.get
// }
}
if (valueOfIndex != null) {
minLength = Math.min(minLength, valueOfIndex.length);
}
result.setMinSize(minLength);
return result;
} else {
return super.getPrecondition();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy