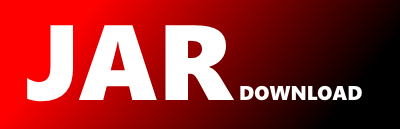
net.sf.gluebooster.java.booster.basic.transformation.CallableByCopying Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.transformation;
import java.util.Map;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.Callable;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.CallableAbstraction;
import net.sf.gluebooster.java.booster.essentials.meta.HasAttributes;
import net.sf.gluebooster.java.booster.essentials.objects.BoostedObject;
import net.sf.gluebooster.java.booster.essentials.utils.ThrowableBoostUtils;
/**
* Copies objects
*
* @author cbauer
*
*/
public class CallableByCopying extends CallableAbstraction {
/**
* The source to copy from
*/
private Object from;
/**
* The target to copy to
*/
private Object to;
/**
* The keys to copy
*/
private Object[] keys;
public CallableByCopying() {
}
/**
*
* @param name
* the name of the instance
*/
public CallableByCopying(Object name, Object from, Object to, Object... keys) {
setName(name);
this.from = from;
this.to = to;
this.keys = keys;
}
@Override
public Object callImpl(Object... parameters) throws Exception {
Object copyFrom = from;
Object copyTo = to;
Object[] copyKeys = keys;
int index = 0;
if (copyFrom == null) {
copyFrom = parameters[index++];
}
if (copyTo == null) {
copyTo = parameters[index++];
}
if (copyKeys == null) {
copyKeys = (Object[]) parameters[index++];
}
for (Object key : copyKeys) {
Object value;
if (copyFrom instanceof Map) {
value = ((Map) copyFrom).get(key);
} else if (copyFrom instanceof HasAttributes) {
value = ((HasAttributes) copyFrom).getAttribute(key);
} else {
value = copyFrom;
}
if (copyTo instanceof Map) {
((Map) copyTo).put(key, value);
} else if (copyTo instanceof HasAttributes) {
((HasAttributes) copyFrom).setAttribute(key, value);
} else {
throw ThrowableBoostUtils.createNotImplementedException("copy to unsupported class ", copyTo.getClass());
}
}
return copyTo;
}
public Object getFrom() {
return from;
}
public void setFrom(Object from) {
this.from = from;
}
public Object getTo() {
return to;
}
public void setTo(Object to) {
this.to = to;
}
public Object[] getKeys() {
return keys;
}
public void setKeys(Object[] keys) {
this.keys = keys;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy