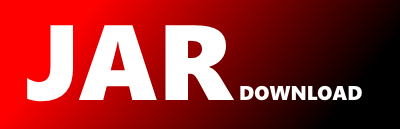
net.sf.gluebooster.java.booster.basic.transformation.CallableToString Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gb-basic Show documentation
Show all versions of gb-basic Show documentation
Basic classes to support the development of applications. There should be as few dependencies on other frameworks as possible.
The newest version!
package net.sf.gluebooster.java.booster.basic.transformation;
import java.io.InputStream;
import java.io.Reader;
import org.apache.commons.io.IOUtils;
import net.sf.gluebooster.java.booster.essentials.eventsCommands.CallableAbstraction;
/**
* Converts objects into strings.
*
* @defaultParamText name name of the callable
*
* @author cbauer
*
*/
public class CallableToString extends CallableAbstraction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy