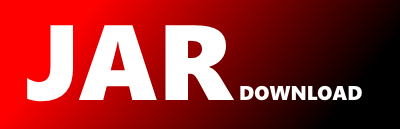
org.gwtwidgets.client.ui.cal.SimpleCalendar Maven / Gradle / Ivy
/*
* Copyright 2007
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gwtwidgets.client.ui.cal;
import java.util.Date;
import java.util.List;
import org.gwtwidgets.client.ui.cal.CalendarDate;
import org.gwtwidgets.client.ui.cal.CalendarEvent;
import org.gwtwidgets.client.ui.cal.CalendarListener;
import org.gwtwidgets.client.ui.cal.CalendarMonth;
import org.gwtwidgets.client.ui.cal.CalendarPanel;
import com.google.gwt.user.client.ui.ClickListener;
import com.google.gwt.user.client.ui.ClickListenerCollection;
import com.google.gwt.user.client.ui.Composite;
import com.google.gwt.user.client.ui.FlowPanel;
import com.google.gwt.user.client.ui.HorizontalPanel;
import com.google.gwt.user.client.ui.Label;
import com.google.gwt.user.client.ui.SourcesClickEvents;
/**
* Renders a simple calendar with (or optionally) without)
* controls to change the month and year of the calendar displayed.
* Allows events to be added to the calender, and a ClickListener
* may be added in order to receve notification when a date
* cell is clicked.
*
* SimpleCalendar ships with three sample CSS stylesheets
* that can be injected or linked in the HTML file. The
* following stylesheets are available.
*
* <stylesheet src="style/gwl-simplecalendar-blue.css" />
* <stylesheet src="style/gwl-simplecalendar-green.css" />
* <stylesheet src="style/gwl-simplecalendar-orange.css" />
*
* Style information
*
* .gwl-simpleCalendar { the root container }
* .gwl-calControls { the controls container }
* .gwl-calNavControl { each of the 4 control links }
* .gwl-calDateDisplay { displayed date }
*
* See CalendarPanel for a list of styles that apply to
* the calendar grid.
*
* NOTE: If you are using the supplied CSS files in conjunction
* with CalendarEvent objects that have CSS class names, you may
* encounder rending issues. For example, an event with the class
* name of "holiday" may not render as expected when you use the
* CSS selector, e.g. ".holiday". To work around this, include the parent
* class selector for the SimpleCalendar as well,
* e.g. ".gwl-simpleCalendar .holiday".
*
* @author rhanson
*/
public class SimpleCalendar extends Composite implements SourcesClickEvents
{
private final SimpleCalendar thisSimpleCalendar = this;
private FlowPanel layout = new FlowPanel();
private CalendarPanel cal;
private ClickListenerCollection clickListeners = new ClickListenerCollection();
private CalendarDate dateSelected = null;
/**
* Render a SimpleCalendar for the current month.
*/
public SimpleCalendar ()
{
this(new Date(), true);
}
/**
* Render a SimpleCalendar for the month specified Date.
* @param date
* @param showControls pass false to hide next/prev controls
*/
public SimpleCalendar (Date date, boolean showControls)
{
this(date.getMonth(), date.getYear() + 1900, showControls);
}
/**
* Render a SimpleCalendar for the month specified month/year.
* @param month 0-11
* @param year e.g. 2008
* @param showControls pass false to hide next/prev controls
*/
public SimpleCalendar (int month, int year, boolean showControls)
{
this(month, year, showControls, new CalendarFactory());
}
/**
* Render a SimpleCalendar for the month specified month/year.
* @param month 0-11
* @param year e.g. 2008
* @param showControls pass false to hide next/prev controls
* @param factory the CalendarFactory to use for tracking events
*/
public SimpleCalendar (int month, int year, boolean showControls, CalendarFactory factory)
{
cal = new CalendarPanel(month, year, factory);
initWidget(layout);
setStyleName("gwl-simpleCalendar");
final Label dateDisplay = new Label();
Label nextMonth = new Label(showControls ? ">" : "");
Label prevMonth = new Label(showControls ? "<" : "");
Label nextYear = new Label(showControls ? ">>" : "");
Label prevYear = new Label(showControls ? "<<" : "");
HorizontalPanel controls = new HorizontalPanel();
controls.add(prevYear);
controls.add(prevMonth);
controls.add(dateDisplay);
controls.add(nextMonth);
controls.add(nextYear);
controls.addStyleName("gwl-calControls");
nextMonth.addStyleName("gwl-calNavControl");
nextYear.addStyleName("gwl-calNavControl");
prevMonth.addStyleName("gwl-calNavControl");
prevYear.addStyleName("gwl-calNavControl");
dateDisplay.addStyleName("gwl-calDateDisplay");
cal.setBorderWidth(0);
cal.setCellPadding(0);
cal.setCellSpacing(0);
if (showControls) {
cal.addNextMonthActivator(nextMonth);
cal.addPrevMonthActivator(prevMonth);
cal.addNextYearActivator(nextYear);
cal.addPrevYearActivator(prevYear);
}
cal.addCalendarListener(new CalendarListener()
{
public void onDateClick (CalendarDate date)
{
dateSelected = date;
clickListeners.fireClick(thisSimpleCalendar);
}
public boolean onEventDateClick (CalendarDate date)
{
return true;
}
public void onMonthChange (CalendarMonth month)
{
String text = cal.getCurrentMonthName() + " " + month.getYear();
dateDisplay.setText(text);
}
});
layout.add(controls);
layout.add(cal);
}
/**
* Add a ClickListener that will receive events when a user
* clicks on a day cell.
*
* For example: the following code adds a listener that
* will popup a message with the date that was clicked.
*
*
* SimpleCalendar cal = new SimpleCalendar();
* cal.addClickListener(new ClickListener() {
* public void onClick (Widget sender) {
* CalendarDate date = ((SimpleCalendar) sender).getDateSelected();
* Window.alert(date.toString());
* }
* });
*
*/
public void addClickListener (ClickListener listener)
{
clickListeners.add(listener);
}
public void removeClickListener (ClickListener listener)
{
clickListeners.remove(listener);
}
/**
* Returns the CalendarDate object for the last date
* cell that was clicked.
* @return last CalendarDate clicked or null
*/
public CalendarDate getDateSelected ()
{
return dateSelected;
}
/**
* @param date
* @param timeSignificant
* @return
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#addEvent(java.util.Date, boolean)
*/
public CalendarEvent addEvent (Date date, boolean timeSignificant)
{
return cal.addEvent(date, timeSignificant);
}
/**
* @param start
* @param end
* @param timeSignificant
* @return
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#addEvent(java.util.Date, java.util.Date, boolean)
*/
public CalendarEvent addEvent (Date start, Date end, boolean timeSignificant)
{
return cal.addEvent(start, end, timeSignificant);
}
/**
* @return
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#getCurrentMonth()
*/
public int getCurrentMonth ()
{
return cal.getCurrentMonth();
}
/**
* @return
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#getCurrentMonthName()
*/
public String getCurrentMonthName ()
{
return cal.getCurrentMonthName();
}
/**
* @return
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#getCurrentYear()
*/
public String getCurrentYear ()
{
return cal.getCurrentYear();
}
/**
* @param date
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#setCalendarMonth(java.util.Date)
*/
public void setCalendarMonth (Date date)
{
cal.setCalendarMonth(date);
}
/**
* @param month
* @param year
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#setCalendarMonth(int, int)
*/
public void setCalendarMonth (int month, int year)
{
cal.setCalendarMonth(month, year);
}
/**
* @param inMonthNames
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#setMonthNames(java.lang.String[])
*/
public void setMonthNames (String[] inMonthNames)
{
cal.setMonthNames(inMonthNames);
}
/**
* @param inWeekDayNames
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#setWeekDayNames(java.lang.String[])
*/
public void setWeekDayNames (String[] inWeekDayNames)
{
cal.setWeekDayNames(inWeekDayNames);
}
/**
*
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#redraw()
*/
public void redraw ()
{
cal.redraw();
}
/**
* @return
* @see org.gwtwidgets.client.ui.cal.CalendarPanel#getEvents()
*/
public List getEvents ()
{
return cal.getEvents();
}
}