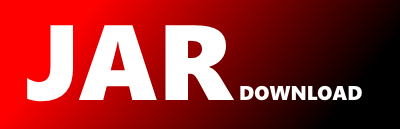
org.gwtwidgets.client.ui.canvas.impl.StringArray Maven / Gradle / Ivy
/*
* Copyright 2008 Robert Hanson
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gwtwidgets.client.ui.canvas.impl;
import com.google.gwt.core.client.JavaScriptObject;
/**
* Concatenates values and returns a comma separated list. This implementations exploits the
* fact that currently (FF2, IE7) operating on javascript arrays is faster than appending to a string.
* @author George Georgovassilis g.georgovassilis[at]gmail.com
*
*/
public class StringArray {
private JavaScriptObject buffer;
private native JavaScriptObject createArray()/*-{
return new Array();
}-*/;
public native StringArray append(String s)/*-{
[email protected]::buffer.push(s);
return this;
}-*/;
public native StringArray append(double d)/*-{
[email protected]::buffer.push(d);
return this;
}-*/;
public native StringArray append(int i)/*-{
[email protected]::buffer.push(i);
return this;
}-*/;
public native String toString()/*-{
return [email protected]::buffer.join(",");
}-*/;
public native String toString(String delimiter)/*-{
return [email protected]::buffer.join(delimiter);
}-*/;
public native void clear()/*-{
[email protected]::buffer.splice(0,[email protected]::buffer.length);
}-*/;
// special implementations of append for performance
public native void appendElement(String sElem, double left, double top, double width, double height)/*-{
[email protected]::buffer.push(
"<",sElem," style='left:",left,"px;top:",top,"px;width:",width,"px;height:",height,"px;position:absolute;' "
);
}-*/;
public native void appendImageData(String src, double relativeOffsetLeft, double relativeOffsetRight, double relativeOffsetTop, double relativeOffsetBottom)/*-{
[email protected]::buffer.push(
" "
);
}-*/;
public native void appendCoordinates(double x, double y)/*-{
[email protected]::buffer.push(x,y);
}-*/;
public native StringArray appendAttribute(String name, String value)/*-{
[email protected]::buffer.push(name,"='",value,"' ");
return this;
}-*/;
public native void closeElement(String elementName)/*-{
[email protected]::buffer.push("",elementName,">");
}-*/;
public StringArray(){
this.buffer = createArray();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy