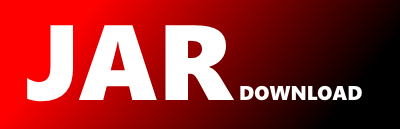
org.gwtwidgets.client.ui.pagination.DefaultPaginationBehavior Maven / Gradle / Ivy
/*
* Copyright 2006 Robert Hanson
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gwtwidgets.client.ui.pagination;
import com.google.gwt.user.client.ui.ClickListener;
import com.google.gwt.user.client.ui.FlexTable;
import com.google.gwt.user.client.ui.Hyperlink;
import com.google.gwt.user.client.ui.Widget;
/**
* Default Pagination Behavior
*
* CSS Style Rules
*
* - .oddRow { }
* - .evenRow { }
* - .oddCell { }
* - .evenCell { }
* - .headerRow { }
* - .headerCell { }
* - .pagingRow { }
* - .noPreviousPageCell { }
* - .previousPageCell { }
* - .pageCell { }
* - .currentPageCell { }
* - .noNextPageCell { }
* - .nextPageCell { }
*
*
* @author Joe Toth ([email protected])
*
*/
abstract public class DefaultPaginationBehavior {
private FlexTable[] pagingControls;
private SortablePaginationBehavior pagination;
private String nextPageText = "Next »";
private String previousPageText = "« Previous";
private int maxPageLinks = 11;
public DefaultPaginationBehavior(FlexTable pagingControl,
FlexTable resultsTable, int resultsPerPage) {
this(new FlexTable[] { pagingControl }, resultsTable, resultsPerPage);
}
public DefaultPaginationBehavior(FlexTable[] pagingControls,
FlexTable resultsTable, int resultsPerPage) {
this.pagingControls = pagingControls;
pagination = new SortablePaginationBehavior(resultsTable,
resultsPerPage) {
protected RowRenderer getRowRenderer() {
return DefaultPaginationBehavior.this.getRowRenderer();
}
protected DataProvider getDataProvider() {
return DefaultPaginationBehavior.this.getDataProvider();
}
protected Column[] getColumns() {
return DefaultPaginationBehavior.this.getColumns();
}
protected void onUpdateSuccess(Object result) {
updatePagingControls();
DefaultPaginationBehavior.this.onUpdateSuccess(result);
}
protected void onUpdateFailure(Throwable caught) {
DefaultPaginationBehavior.this.onUpdateFailure(caught);
}
};
updatePagingControls();
}
private void updatePagingControls() {
for (int i = 0; i < pagingControls.length; i++) {
if (pagingControls[i].getRowCount() > 0) {
pagingControls[i].removeRow(0);
}
pagingControls[i].insertRow(0);
if (pagination.getResults() != null) {
int totalPages = pagination.getResults().getSize()
/ pagination.getResultsPerPage();
if (pagination.getResults().getSize()
% pagination.getResultsPerPage() != 0) {
totalPages++;
}
// Previous Page
Hyperlink previousPageLink = new Hyperlink(previousPageText,
"previousPage");
pagingControls[i].setWidget(0, 0, previousPageLink);
if (pagination.getPage() == 1
|| pagination.getResults().getSize() == 0) {
pagingControls[i].getCellFormatter().addStyleName(0, 0,
"noPreviousPageCell");
} else {
previousPageLink.addClickListener(new ClickListener() {
public void onClick(Widget sender) {
pagination.showPage(pagination.getPage() - 1);
}
});
pagingControls[i].getCellFormatter().addStyleName(0, 0,
"previousPageCell");
}
// Pages
int currentPageForCell = 1;
int totalCells = totalPages;
if (totalPages > maxPageLinks) {
totalCells = maxPageLinks;
if (pagination.getPage() > maxPageLinks / 2) {
currentPageForCell = pagination.getPage()
- (maxPageLinks / 2);
if (pagination.getPage() >= totalPages
- (maxPageLinks / 2)) {
currentPageForCell = totalPages
- (maxPageLinks - 1);
}
}
}
int cellNumber = 1;
for (; cellNumber <= totalCells; cellNumber++) {
Hyperlink pageLink = new Hyperlink(currentPageForCell + "",
"page");
pagingControls[i].setWidget(0, cellNumber, pageLink);
final int tmp = currentPageForCell;
if (pagination.getPage() != currentPageForCell) {
pageLink.addClickListener(new ClickListener() {
public void onClick(Widget sender) {
pagination.showPage(tmp);
}
});
pagingControls[i].getCellFormatter().addStyleName(0,
cellNumber, "pageCell");
} else {
pagingControls[i].getCellFormatter().addStyleName(0,
cellNumber, "currentPageCell");
}
currentPageForCell++;
}
// Next Page
Hyperlink nextPageLink = new Hyperlink(nextPageText, "nextPage");
pagingControls[i].setWidget(0, cellNumber, nextPageLink);
if (pagination.getPage() == totalPages
|| pagination.getResults().getSize() == 0) {
pagingControls[i].getCellFormatter().addStyleName(0,
cellNumber, "noNextPageCell");
} else {
nextPageLink.addClickListener(new ClickListener() {
public void onClick(Widget sender) {
pagination.showPage(pagination.getPage() + 1);
}
});
pagingControls[i].getCellFormatter().addStyleName(0,
cellNumber, "nextPageCell");
}
}
}
}
public void setCell(int row, int column, Widget widget) {
pagination.setCell(row, column, widget);
}
public void showPage(int pageNumber, String orderByProperty,
boolean isAscending) {
pagination.showPage(pageNumber, orderByProperty, isAscending);
}
abstract protected DataProvider getDataProvider();
abstract protected RowRenderer getRowRenderer();
abstract protected Column[] getColumns();
protected void onUpdateSuccess(Object result) {
}
protected void onUpdateFailure(Throwable caught) {
throw new RuntimeException(caught);
}
public int getRowCount() {
return pagination.getRowCount();
}
public PaginationParameters getParameters() {
return pagination.getParameters();
}
public int getResultsPerPage() {
return pagination.getResultsPerPage();
}
public int getPage() {
return pagination.getPage();
}
public Results getResults() {
return pagination.getResults();
}
public String getNextPageText() {
return nextPageText;
}
public void setNextPageText(String nextPageText) {
this.nextPageText = nextPageText;
}
public String getPreviousPageText() {
return previousPageText;
}
public void setPreviousPageText(String previousPageText) {
this.previousPageText = previousPageText;
}
public int getMaxPageLinks() {
return maxPageLinks;
}
public void setMaxPageLinks(int maxPageLinks) {
this.maxPageLinks = maxPageLinks;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy