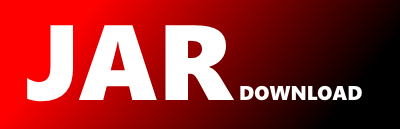
org.gwtwidgets.client.wrap.JSCalendar Maven / Gradle / Ivy
/**
* Copyright [Aaron Watkins]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gwtwidgets.client.wrap;
import org.gwtwidgets.client.style.Color;
import org.gwtwidgets.client.ui.ImageButton;
import com.google.gwt.user.client.Command;
import com.google.gwt.user.client.DOM;
import com.google.gwt.user.client.DeferredCommand;
import com.google.gwt.user.client.ui.HTMLPanel;
import com.google.gwt.user.client.ui.HorizontalPanel;
import com.google.gwt.user.client.ui.TextBox;
/**
* Created on 31/05/2006
* Updated on 19/08/2007
*
* Wrapper for JSCalendar
* NOTE: Expects JavaScript includes to be in enclosing HTML
*
* Author: Aaron Watkins (aaronDOTjDOTwatkinsATgmailDOTcom)
* Website: http://www.goannatravel.com
* Home Page for initial release of this widget: http://consult.goannatravel.com/code/gwt/jscalendar.php
*
* Example Usage
*
* Add the following code to your HTML page in the <head>.
* These point to files that come with JSCalendar when you downloaded
* it. GWT-WL does not show with JSCalendar, so you will need to
* download it yourself from http://www.dynarch.com/projects/calendar/,
* and then change the paths in the example below to point to the
* directory where you placed them. In the example below, the
* JSCalendar files are in /jscalendar.
*
*
*
* <script src='/jscalendar/calendar_stripped.js' type='text/javascript'></script>
* <script src='/jscalendar/calendar-setup_stripped.js' type='text/javascript'></script>
* <script src='/jscalendar/lang/calendar-en.js' type='text/javascript'></script>
* <link rel="stylesheet" type="text/css" href="/jscalendar/skins/aqua/theme.css" />
*
*
* With that in place, you can use GWT to create a JSCalendar TextBox with a
* calendar button next to it. The calendar button uses an image that comes
* with JSCalendar. Assuming that you installed JSCalender at /jscalendar, the
* image lives at /jscalendar/img.gif. This is the location used by the default
* constructor. If you installed JSCalendar elsewhere, or want to use a
* different image, select one of the constructors that takes an image
* path as the first argument.
*
*
* JSCalendar cal = new JSCalendar();
* RootPanel.get().add(cal);
*
*
* The user can now click the calendar button to have a pop-up calendar
* appear. Once a date is clicked the calendar will disappear and the
* TextBox will be populated with the selected date.
*
* @author Aaron Watkins (aaronDOTjDOTwatkinsATgmailDOTcom)
* @author Additional class docs by Robert Hanson
*/
public class JSCalendar extends HorizontalPanel {
private ImageButton button;
private TextBox date;
private String textId;
private String buttonId;
private static final String defaultImgLocation = "jscalendar/img.gif";
/**
* JSCalendar() -
*
* Create a new instance of JSCalendar.
* Default constructor
*/
public JSCalendar ()
{
this(defaultImgLocation);
}
/**
* JSCalendar() -
*
* Create a new instance of JSCalendar.
* Default constructor
* @param imgLocation - the path to the image to use for the button
*/
public JSCalendar (String imgLocation)
{
this(imgLocation, new Color(255, 0, 0));
}
/**
* JSCalendar() -
*
* Create a new instance of JSCalendar.
* Default constructor
* @param imgLocation - the path to the image to use for the button
* @param background - the background colour to use on mouseover
*/
public JSCalendar (String imgLocation, Color background)
{
super();
date = new TextBox();
date.setEnabled(false);
date.setVisibleLength(10);
textId = HTMLPanel.createUniqueId();
DOM.setElementAttribute(date.getElement(), "id", textId);
add(date);
button = new ImageButton(imgLocation, 20, 14);
button.setBackgroundOnColor(background);
buttonId = HTMLPanel.createUniqueId();
DOM.setElementAttribute(button.getElement(), "id", buttonId);
add(button);
}
/**
* getDateBox() -
*
* Simple getter for the datebox
* @return the datebox widget
*/
public TextBox getDateBox ()
{
return date;
}
/**
* onLoad() -
*
* Runs when widgets has been loaded into the browser.
* Initialises the calendar widget
*/
protected void onLoad ()
{
DeferredCommand.addCommand(new Command()
{
public void execute ()
{
initCalendar(textId, buttonId);
}
});
}
/**
* initCalendar() -
*
* Javascript native function that initialises the calendar widget
* @param textId - the DOM id of the textbox to display the date in
* @param buttonId - the DOM id of the button to trigger from
*/
protected native void initCalendar (String textId, String buttonId) /*-{
$wnd.Calendar.setup({
inputField : textId, // id of the input field
button : buttonId, // trigger for the calendar (button ID)
align : "Bl", // alignment (defaults to "Bl")
singleClick : true
});
}-*/;
/**
* getDate() -
*
* Simple function that formats the date.
* NOTE: This should use Java built in date formatting
* Possibly not supported by GWT yet
* @param year
* @param month
* @param day
* @return the formatted date
*/
public static String getDate (int year, int month, int day)
{
String y = year + "";
String m = month + "";
if (month < 10) {
m = "0" + month;
}
String d = day + "";
if (day < 10) {
d = "0" + day;
}
return y + "/" + m + "/" + d;
}
}